Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial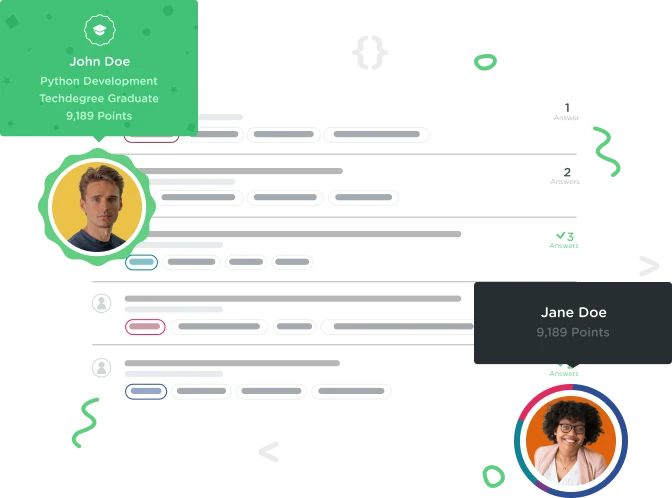

Jake Basten
16,345 PointsRepeatDetector.Scan detects repetitions when there are none.
I can't see what i am doing wrong here; i have tried out several attempts in the REPL and they all work :/
namespace Treehouse.CodeChallenges
{
class SequenceDetector
{
public virtual bool Scan(int[] sequence)
{
return true;
}
}
}
namespace Treehouse.CodeChallenges
{
class RepeatDetector : SequenceDetector{
public override bool Scan(int[] sequence){
foreach(int i in sequence){
if (i.Equals(sequence[i-1])){
return true;
}
}
return false;
}
}
}
1 Answer
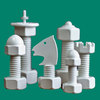
Steven Parker
229,788 PointsThose REPL tests may not be doing what you think.
Take a look at these 2 lines of your method:
foreach(int i in sequence){
if (i.Equals(sequence[i-1]))
The foreach will assign the value of an array element to your variable i. But on the next line, you treat i as both a value and an index. This may have appeared to work if the array contents were sequential integers starting with 1. But what if the array contained something like this: { 21, 99, 3003, 22788 }
? (Try it in the REPL and see!)
But the idea of using an index is good, and while you could maintain an index in a foreach by using a separate variable, a simple for loop is more suited to indexing. Plus, you can start your index with 1 instead of 0 to insure the first comparison will be in bounds.
So if you wrote your loop like this:
for (var i = 1; i < sequence.Length; i++)
That would produce your indexes. Then you just have to be sure you're checking values against values on the next line. So that might look like one of these:
if (sequence[i].Equals(sequence[i-1]) // effective, but perhaps a bit esoteric
if (sequence[i] == sequence[i-1]) // simpler and more direct
Code below this line is not "best practice":
Now, just for fun (and being deliberately esoteric), you could also complete this challenge using a while loop:
public override bool Scan(int[] s)
{
int i = s.Length - 1;
while (i > 0 && s[i] != s[--i]);
return i > 0;
}
Jake Basten
16,345 PointsJake Basten
16,345 PointsThanks Steven, that's great :)