Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial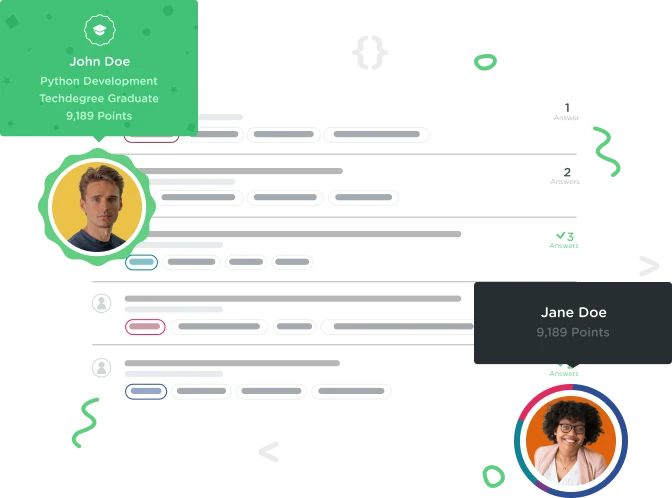

William Schultz
2,926 PointsRepeatDetector.Scan detects repetitions when there are none.
Ok, so I know there is one answer to this question already, BUT that answer does not address why this error is happening. As you can see from my code all I have done is copy the method from the Parent class and changed the word "virtual" to "override". Nothing else has been done. So why the error about repetitions? What repetitions is it referring to? I don't get it. No matter what code I use inside my method that overrides the parent member I get the same error. The error has to refer to something besides the code inside the method and I just don't know what.
namespace Treehouse.CodeChallenges
{
class SequenceDetector
{
public virtual bool Scan(int[] sequence)
{
return true;
}
}
}
namespace Treehouse.CodeChallenges
{
class RepeatDetector : SequenceDetector
{
public override bool Scan(int[] sequence)
{
return true;
}
}
}

andren
28,558 PointsSorry for the late reply Yi Tian, I realize you might have noticed the issue yourself by now but in case you haven't the issue with your code is that both of the return
statements in your code return true
. Your last return
statement should be returning false
instead.
Like this:
namespace Treehouse.CodeChallenges {
class RepeatDetector : SequenceDetector
{
public override bool Scan(int[] sequence)
{
for (int i = 1; i < sequence.Length; ++i)
{
if ( sequence[i] == sequence[i-1] )
{
return true;
}
}
return false; // Replaced true with false
}
}
}
2 Answers

andren
28,558 PointsThe method you are writing is meant to check the sequence
parameter it gets in and return true
if there are any duplicate values immediately preceding one another. In your example solution you are doing nothing but returning true
, which means the function will always respond with true even if the sequence passed in has no repetitions. That's what the error message refers to.
If you change the true
statement in your code to false
you would get a different error message, essentially stating that it doesn't detect repetitions when there is one.
So to answer your question more directly, the error is one generated by Treehouse's code checker (not by C# itself) due to the fact that the method does not perform the task they instructed it to do. The error will continue to pop up until you write code that correctly identifies repetitions in the sequence that is passed to the method.

William Schultz
2,926 PointsOk, that makes sense. Thank you for taking the time to explain it to me!
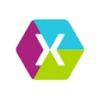
Chanuka Weerasinghe
2,337 PointsWhenever you need an array check, this block will comes in handy.
for (int i = 0; i < a.Length; i++) { for (int j = i + 1; j < a.Length; j++) { if (a[i] == a[j]) return true; } } return false;
Yi Tian
Courses Plus Student 1,663 PointsYi Tian
Courses Plus Student 1,663 Pointsnamespace Treehouse.CodeChallenges {
}
what are wrong with my code? It still shows RepeatDetector.Scan detects repetitions when there are none.