Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial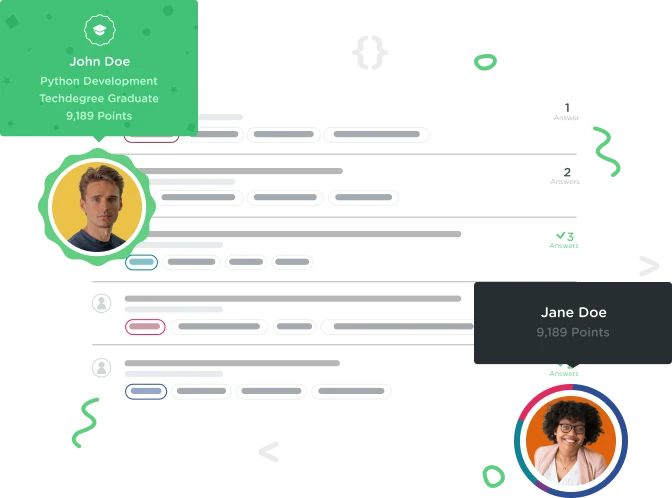

Gary Gibson
5,011 PointsRepeating Spaces in Hangman Game
Here is my code:
import random
words = [
"apple"
"banana"
"orange"
"coconut"
"strawberry"
"lime"
"grapefruit"
"lemon"
"blueberry"
"melon"
]
while True:
start = input("Press any key to start, or enter 'q' to quit.")
if start.lower() == "q":
break
secret_word = random.choice(words)
bad_guesses = []
good_guesses = []
while len(bad_guesses) < 7 and len(good_guesses) != len(list(secret_word)):
for letter in secret_word:
if letter in good_guesses:
print(letter, end='')
else:
print('_', end='')
print("")
print("Strikes: {}/7".format(len(bad_guesses)))
print("")
guess = input("Guess a letter: ").lower()
if len(guess) != 1:
print("You can only guess a single letter a time!")
continue
elif guess in bad_guesses or guess in good_guesses:
print("You've already guessed that letter!")
elif not guess.isalpha():
print("You can only guess letters!")
continue
if guess in secret_word:
good_guesses.append(guess)
if len(good_guesses) == len(list(secret_word)):
print("You win! The word was {}.".format(secret_word))
break
else:
bad_guesses.append(guess)
else:
print("You didn't guess the word. My secret word was {}.".format(secret_word))
And here is an example of what I get:
You can only guess a single letter a time!
l________________________l_______ll____
Strikes: 0/7
Why are so many underscores appearing?
4 Answers
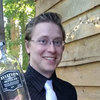
Evan Demaris
64,262 PointsAh, I was spot checking before. Looking through the rest of the code, you've got a problematic section of while
that's also nearly repeated in the later if
block;
len(good_guesses) != len(list(secret_word))
This doesn't take into account having repeat letters in your secret word; for example, 'banana' would require 6 correct guesses even though it should only require 3 correct guesses.
Also, you skipped the 'continue' for the clause;
elif guess in bad_guesses or guess in good_guesses:
print("You've already guessed that letter!")
which means that it will still append the guess to good or bad guesses, even if you've already guessed it before. So with your code as-is (with some commas), you could win, but you'd need to re-guess a letter you'd already guessed until you'd accounted for every repeating character in secret_word.
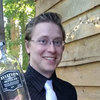
Evan Demaris
64,262 PointsHi Gary,
Because you didn't include any commas in your words
list, the entire list is being read as a single string.
Hope that helps!

Gary Gibson
5,011 PointsHi Evan,
Thank you...but now the code won't tell me when I've guessed the word. It just keeps asking for more letters and only breaks when I use up my chances with the wrong letters..?

Gary Gibson
5,011 PointsThanks for getting back so quickly, Evan!
I caught the missing "continue"...but I only had to guess a letter once and it would fill in all throughout the blank spaces where it should.

Gary Gibson
5,011 PointsIt's still not going to either:
if len(good_guesses) == len(list(secret_word)):
print("You win! The word was {}.".format(secret_word))
break
or:
else:
print("You didn't guess the word. My secret word was {}.".format(secret_word))
when either the appended string matches the secret word or all seven guesses are used up.
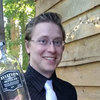
Evan Demaris
64,262 PointsHi Gary,
You've definitely got a correct for
block going on the underscores, but that doesn't impact the while
statement above it or the if
block below it.

Gary Gibson
5,011 PointsHow do I correct? I was trying to follow along with the lesson (and still creating bugs unwittingly).
Right now it's only giving me the message if I run out of attempts.
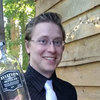
Evan Demaris
64,262 PointsI believe your code is now functioning as expected at this point in the lesson, so from here you'd either want to continue updating with the lesson, or diverge onto your own path and write the program out so that it functions in the way you want it to.