Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial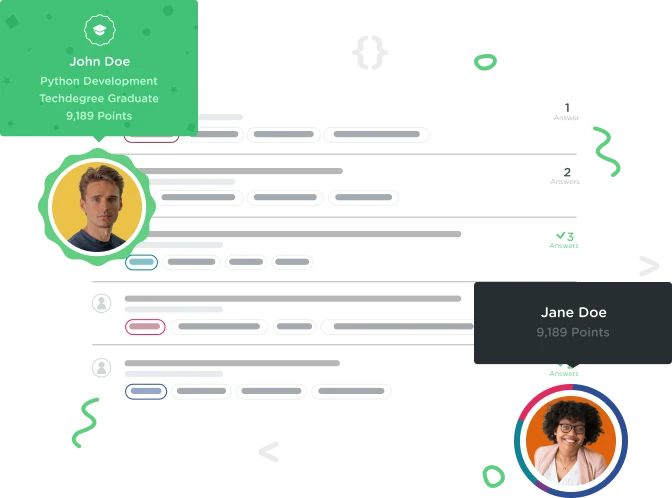
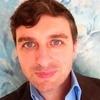
Arsene Lavaux
4,342 PointsReplace anonymous Instagram profile picture by app logo on UICollectionView
When Instagram returns an anonymous profile picture from a user, I would like to display my app's logo instead.
For now, I am setting my friends dictionary with the raw data coming back from Instagram:
- (void)refreshFriends {
if (self.loading) {
return;
}
self.loading = YES;
NSURLSession *session = [NSURLSession sharedSession];
NSString *urlString = [[NSString alloc] initWithFormat:@"https://api.instagram.com/v1/users/self/follows?count=-1&access_token=%@", self.accessToken]; //Get avatar pictures of follows from Instagram
NSURL *url = [[NSURL alloc] initWithString:urlString];
NSURLRequest *request = [[NSURLRequest alloc] initWithURL:url];
NSURLSessionDownloadTask *task = [session downloadTaskWithRequest:request completionHandler:^(NSURL *location, NSURLResponse *response, NSError *error) {
NSData *data = [[NSData alloc] initWithContentsOfURL:location];
NSDictionary *responseDictionary = [NSJSONSerialization JSONObjectWithData:data options:kNilOptions error:nil];
self.friends = [responseDictionary valueForKeyPath:@"data"];
dispatch_async(dispatch_get_main_queue(), ^{
[self.collectionView reloadData];
self.loading = NO;
});
NSLog(@"Data on friends: %@", self.friends);
}];
[task resume];
}
The url to the anonymousUser picture on Instagram is http://scontent-b.cdninstagram.com/hphotos-xpf1/outbound-distillery/l/t0.0-20/OBPTH/profiles/anonymousUser.jpg
So, the idea would be to implement a cleanUpArray method which uses an NSArray as argument and would return a clean NSArray (where the NSString representing the anonymousUser photo is replaced by something that can allocate my app's logo, ideally from a logo that I drop locally in my project, otherwise on box I guess).
Here is the structure of the array I get back from instagram: https://www.dropbox.com/s/wfrtbp3loyux5el/CleanUp_NSArray_Method.png
Here is the beginning of an attempt on the cleanUpArray method:
- (NSArray)cleanUpArray:(NSArray *)array {
for (int i = 0; i < [array count]; i++)
{
if ([array[i][@"caption"][@"from"][@"profile_picture"] isEqualToString:@"http://scontent-b.cdninstagram.com/hphotos-xpf1/outbound-distillery/l/t0.0-20/OBPTH/profiles/anonymousUser.jpg"]) {
array[i][@"caption"][@"from"][@"profile_picture"] = [array[i][@"caption"][@"from"][@"profile_picture"] stringByReplacingOccurrencesOfString:[@"http://scontent-b.cdninstagram.com/hphotos-xpf1/outbound-distillery/l/t0.0-20/OBPTH/profiles/anonymousUser.jpg" objectAtIndex:i] withString:[stringsReplaceBy objectAtIndex:i]];
}
}
}
Which seems way too complicated. I am sure more experienced coders could do that in a more concise way.
Looking forward to feedback from community.
Thanks/Merci!
2 Answers

kerdeseverin
9,688 PointsRather than putting that long url, you could check whether anonymousUser is included in the url and if it is then you can make the changes.
if ([[array[i][@"caption"][@"from"][@"profile_picture"] rangeOfString:@"anonymousUser"].location != NSNotFound) {
NSLog(@"I have that string!");
array[i][@"caption"][@"from"][@"profile_picture"] = @"yourImage.png"
[(NSDictionary*)myArray[i] setValue:@"myFlag" forKey:@"mykey"];
}
I created a flag in the dictionaries at array[i] only for those where the image was changed to yourImage.png. This is to let me know if I need to parse the url or use UImageNamed to load my local file.
So in a later method you have this check:
if( [(NSDictionary*)myArray[i] objectForKey:@"mykey"]){
//if the key has a value do UIImageNamed
}
else{
//parse url
}
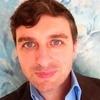
Arsene Lavaux
4,342 PointsHi Kerde: Not sure if you'll be reading this.
If you are, would value your point of view on this: https://teamtreehouse.com/forum/remove-nsmutabledictionary-entry