Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial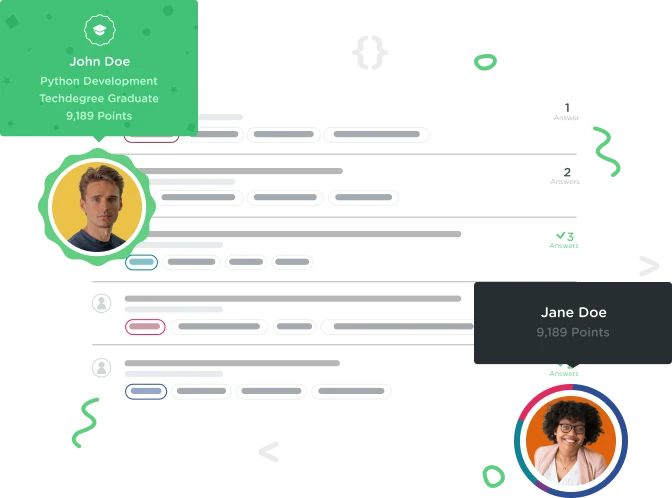
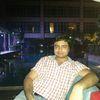
KnowledgeWoods Consulting
5,607 PointsReplace hyphen (-) with comma (,) in php
Hi, Thanks in advance. Replace - with , in php
Nov 07 ‐ 08 & 14 ‐ 15 (Hyphen is occurring twice)
2 Answers
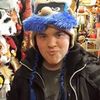
Codin - Codesmite
8,600 PointsYou can use PHP function str_replace().
<?php
$string = "Nov 07 ‐ 08 & 14 ‐ 15";
$string = str_replace("‐",",",$string);
echo $string;
// This will output: "Nov 07 , 08 & 14 , 15"
?>
To remove the first space before the comma simply include a whitespace before the - in your str_replace():
<?php
$string = "Nov 07 ‐ 08 & 14 ‐ 15";
$string = str_replace(" ‐",",",$string);
echo $string;
// This will output: "Nov 07, 08 & 14, 15"
?>
Another quick example that shows a bit more clearly and easier to understand how the syntax for str_replace works:
<?php
$string = "The dog is brown and the cat is also brown.";
$string = str_replace("brown", "black", $string);
echo $string;
// Will output: "The dog is black and the cat is also black."
?>
You can also use arrays for multiple values:
<?php
$string = "You should eat fruits, vegetables, and fiber every day.";
$healthy = array("fruits", "vegetables", "fiber");
$yummy = array("pizza", "beer", "ice cream");
$string = str_replace($healthy, $yummy, $string);
echo $string;
// This will output "You should eat pizza, beer and ice cream every day."
?>
There is more you can do with str_replace() check out the PHP manual for more info: http://php.net/manual/en/function.str-replace.php
Hope this helps :)
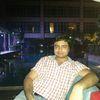
KnowledgeWoods Consulting
5,607 PointsHi, Thanks for the reply. I tried this but its not working.
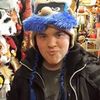
Codin - Codesmite
8,600 PointsThere should be no reason why the code above does not work, double check that in your code it is a hyphen and not an ndash, this will matter even though similiar in look they are different characters.
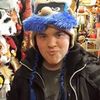
Codin - Codesmite
8,600 PointsAh just seen in my examples for some reason my hyphens were relaced with endash. So if you copy and pasted it, it probably didn't work for your string.
I fixed it now.
Your best bet is to copy and paste the hyphen from your data output into your code so you are definitally getting the correct character.