Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial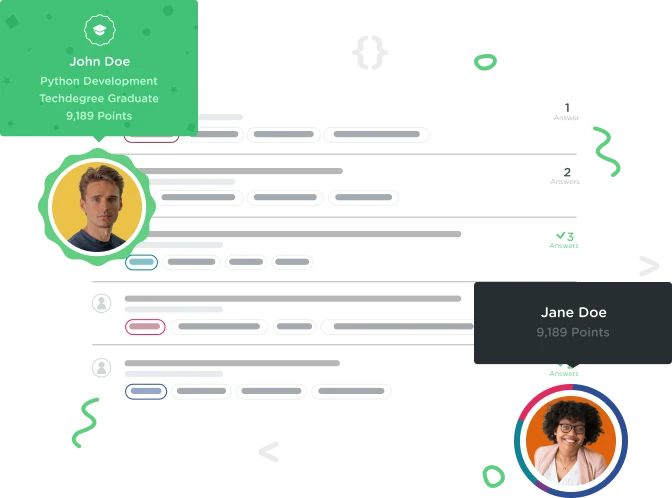

Logan Fox
Courses Plus Student 3,379 PointsReplace the hard coded values in the output with the name and email values from the contacts array
What am I doing wrong here?
<?php
//edit this array
$contacts = array('Alena Holligan', 'Dave McFarland', 'Treasure Porth', 'Andrew Chalkley');
echo "<ul>\n";
//$contacts[0] will return 'Alena Holligan' in our simple array of names.
echo "<li>$contacts[0]['name'] : $contacts[0]['email']</li>\n";
echo "<li>$contacts[1]['name'] : $contacts[1]['email']</li>\n";
echo "<li>$contacts[2]['name'] : $contacts[2]['email']</li>\n";
echo "<li>$contacts[3]['name'] : $contacts[3]['email']</li>\n";
echo "</ul>\n";
$contacts = [
['name' => 'Alena Holligan',
'email' => 'alena.holligan@teamtreehouse.com'
],
['name' => 'Dave McFarland',
'email' => 'dave.mcfarland@teamtreehouse.com'
],
['name' => 'Treasure Porth',
'email' => 'treasure.porth@teamtreehouse.com'
],
['name' => 'Andrew Chalkley',
'email' => 'andrew.chalkley@teamtreehouse.com'
]
];
4 Answers
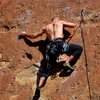
Shawn Flanigan
Courses Plus Student 15,815 PointsHey Logan,
Just looking at your code from my phone, so it's tough to try any edits (also haven't looked at the challenge), but I'm guessing you need to move the edited array so that it will be above your echo statements. As it's written now, you don't add the name and email parameters to your array until after you've tried to echo them out.
Does that make sense? Hopefully that helps!

Logan Fox
Courses Plus Student 3,379 PointsI tried that but now it says: Oops! It looks like Task 1 is no longer passing.
<?php
//edit this array
$contacts = array('Alena Holligan', 'Dave McFarland', 'Treasure Porth', 'Andrew Chalkley');
echo "<ul>\n";
//$contacts[0] will return 'Alena Holligan' in our simple array of names.
echo "<li>" . $contacts[0]['name'] . ':' . $contacts[0]['email']"</li>\n";
echo "<li>" . $contacts[1]['name'] . ':' . $contacts[1]['email']"</li>\n";
echo "<li>" . $contacts[2]['name'] . ':' . $contacts[2]['email']"</li>\n";
echo "<li>" . $contacts[3]['name'] . ':' . $contacts[3]['email']"</li>\n";
echo "</ul>\n";
$contacts = [
['name' => 'Alena Holligan',
'email' => 'alena.holligan@teamtreehouse.com'
],
['name' => 'Dave McFarland',
'email' => 'dave.mcfarland@teamtreehouse.com'
],
['name' => 'Treasure Porth',
'email' => 'treasure.porth@teamtreehouse.com'
],
['name' => 'Andrew Chalkley',
'email' => 'andrew.chalkley@teamtreehouse.com'
]
];
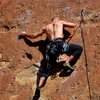
Shawn Flanigan
Courses Plus Student 15,815 PointsGetting close! You'll still need to move your new $contacts up above your echo statements. You're also missing the period between each ['email'] and "</li>..."

Logan Fox
Courses Plus Student 3,379 PointsI did this and it says: Bummer! I do not see the required output. You should not be modifying the output at this point.
<?php
//edit this array
$contacts = array('Alena Holligan', 'Dave McFarland', 'Treasure Porth', 'Andrew Chalkley');
echo "<ul>\n";
//$contacts[0] will return 'Alena Holligan' in our simple array of names.
$contacts = [
['name' => 'Alena Holligan',
'email' => 'alena.holligan@teamtreehouse.com'
],
['name' => 'Dave McFarland',
'email' => 'dave.mcfarland@teamtreehouse.com'
],
['name' => 'Treasure Porth',
'email' => 'treasure.porth@teamtreehouse.com'
],
['name' => 'Andrew Chalkley',
'email' => 'andrew.chalkley@teamtreehouse.com'
]
];
echo "<li>" . $contacts[0]['name'] . ':' . $contacts[0]['email'] . "</li>\n";
echo "<li>" . $contacts[1]['name'] . ':' . $contacts[1]['email'] . "</li>\n";
echo "<li>" . $contacts[2]['name'] . ':' . $contacts[2]['email'] . "</li>\n";
echo "<li>" . $contacts[3]['name'] . ':' . $contacts[3]['email'] . "</li>\n";
echo "</ul>\n";
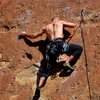
Shawn Flanigan
Courses Plus Student 15,815 PointsIt's a picky formatting thing. They want to see a space before and after each colon. So...where you have ':' you should have ' : '
Without the spaces, your new output isn't exactly the same as the original output. The little details can drive you crazy, can't they?

Logan Fox
Courses Plus Student 3,379 PointsYay it finally worked!! yes those little details are buggers!
Logan Fox
Courses Plus Student 3,379 PointsLogan Fox
Courses Plus Student 3,379 PointsI will try that
Logan Fox
Courses Plus Student 3,379 PointsLogan Fox
Courses Plus Student 3,379 PointsI tried that but it said: I do not see the required output. You should not be modifying the output at this point.
Shawn Flanigan
Courses Plus Student 15,815 PointsShawn Flanigan
Courses Plus Student 15,815 PointsAh, I see. You also have a little syntax problem with your
echo
statements. You'll need to break the variables out from your strings so that the PHP processor knows to replace them with the variable values. For example...this code:will output My name is $name
Taking the variable out of the string literal, and concatenating the value to your string like so:
will output My name is Shawn
You can use multiple variables in the same echo statement like so:
Use those principles to put it all together and you should be good to go! If you have questions about the . $variable . syntax, let me know.