Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial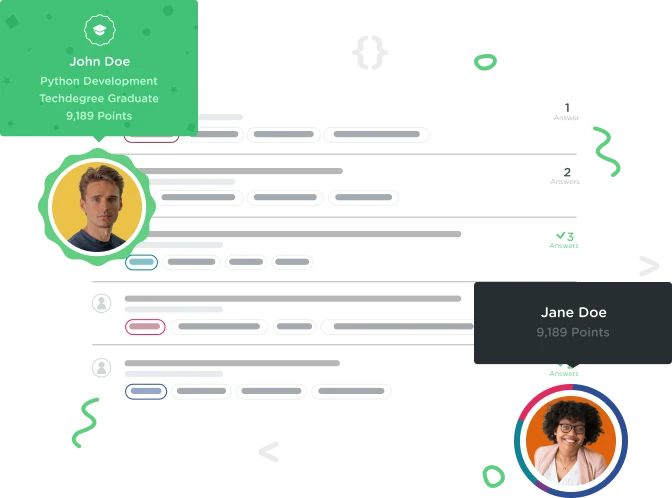
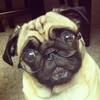
Keith Short
5,971 PointsReplacing elements on the webpage with node.js
I am making a web app which will query a database and display some data in a <table>.
As node.js is non-blocking, the entire webpage will have been created and response.end(); will have happened before the function that queries data from the database has finished.
As such I need to manipulate elements on the page once the query has finished.
I have a placeholder {{table}} on my html page, how will I replace this with my <table> filled with data from the database?
1 Answer
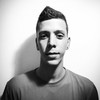
Jesus Mendoza
23,289 PointsYou add the response inside the query for the database. Example:
app.get('/', function(req, res) {
DbCollection.find(function(err, docs) {
if (err) return console.log(err);
res.render('index', { docs: docs });
})
}
DbCollection is the name of your database model
Jesus Mendoza
23,289 PointsJesus Mendoza
23,289 PointsYou can also make a local variable to use with your application where you do exactly the same but use it globally.
And now you can send that data from your routes as json with your render response