Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial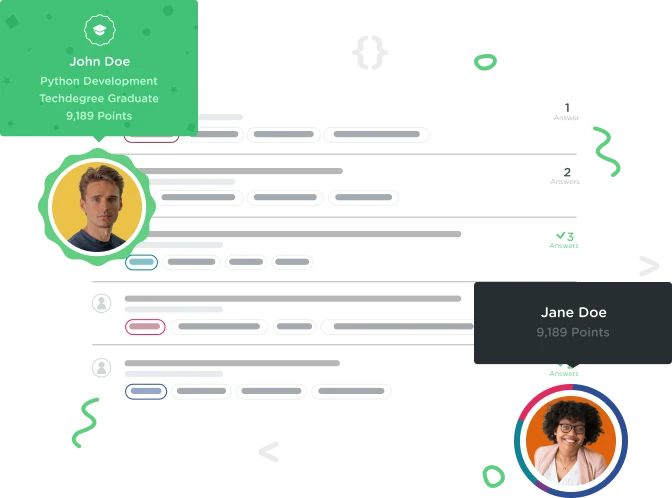
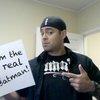
Eduardo Vargas
5,871 PointsReplacing the NOT OPERATOR with ( correctGuess === false )?
Like a lot of people here, the NOT OPERATOR on this exercise kinda through me for a loop (no pun intended). After staring at it for about 30 minutes or so, it began to make some sense. With that said, I replaced ( ! correctGuess ) with ( correctGuess === false ). Isn't this pretty much the same thing and a bit more straight forward?
while ( correctGuess === false )
I ran the the code and it seems to work.
var randomNumber = getRandomNumber(10);
var guess;
var guessCount = 0;
var correctGuess = false;
function getRandomNumber( upper ) {
var num = Math.floor(Math.random() * upper) + 1;
return num;
}
do {
guess = prompt("Guess a number from 1 - 10. What is it?");
guessCount += 1;
if ( parseInt( guess ) === randomNumber ) {
correctGuess = true;
}
} while ( correctGuess === false )
3 Answers
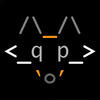
Cazden Gabrielse
Full Stack JavaScript Techdegree Student 9,819 PointsYou are correct in that it provides the exact same functionality and how you write it is ultimately a matter of preference. If using the comparison operator makes it more readable to you, then by all means. The ! operator is just a shorthand way of writing it and that's what the teacher is trying to convey. It's very common in development and there's actually a term for situations like these, called syntactic sugar. You'll notice he uses syntactic sugar in several other areas of this course and previous courses. Here's a very basic example of syntactic sugar in use:
var number = 1; // Initialize our variable
// Compare these two lines
number = number + 1; // Add 1 to number, and store it back into number, which now holds the value of 2
number += 1; // Same functionality as the line above, but shorthand and easier way of writing it, "syntactic sugar"
It's one of those things you'll get used to the more you code, especially when you're handling other people's code.

KRIS NIKOLAISEN
54,971 PointsYour correctGuess variable is intialized to false and only has one statement that would reassign it to true so those are the only two possible values, so yes the results would be the same.
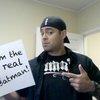
Eduardo Vargas
5,871 PointsAh, cool. With that cleared up, what is the advantage or purpose of writing it the way Dave did? I feel like I'm missing something by dismissing it as mere preference.

Duncan Murtagh
1,557 PointsI was wondering the same Eduardo.
A follow on question, does: while ( ! correctGuess ) ....mean that correctGuess is False, regardless of whether or not you earlier declared: var CorrectGuess = false; ???