Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial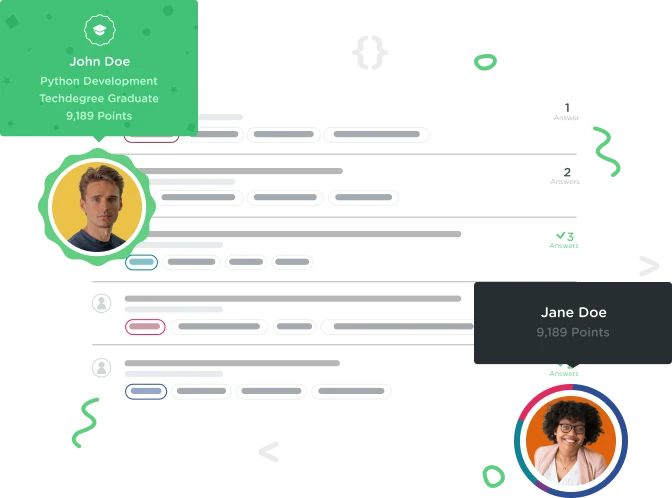

Severin Haselmann
24,517 PointsRepository code Challenge 2
Can someone help explain the logic of this exercise? I cant seem to return the same type.
using Treehouse.Models;
namespace Treehouse.Data
{
public class VideoGamesRepository
{
// TODO Add GetVideoGames method
public VideoGame[] GetVideoGames(int id)
{
VideoGame[] videoToReturn= null;
foreach (var videoGame in _videoGames)
{
if (videoGame.Id == id)
{
videoToReturn = videoGame;
break;
}
}
return videoToReturn;
}
// TODO Add GetVideoGame method
private static VideoGame[] _videoGames = new VideoGame[]
{
new VideoGame()
{
Id = 1,
Title = "Super Mario 64",
Description = "Super Mario 64 is a 1996 platform video game developed and published by Nintendo for the Nintendo 64.",
Characters = new string[]
{
"Mario",
"Princess Peach",
"Bowser",
"Toad",
"Yoshi"
},
Publisher = "Nintendo",
Favorite = true
},
new VideoGame()
{
Id = 2,
Title = "Mario Kart 64",
Description = "Mario Kart 64 is a 1996 go-kart racing game developed and published by Nintendo for the Nintendo 64 video game console.",
Characters = new string[]
{
"Mario",
"Princess Peach",
"Bowser",
"Toad",
"Yoshi"
},
Publisher = "Nintendo",
Favorite = false
}
};
}
}
namespace Treehouse.Models
{
// Don't make any changes to this class!
public class VideoGame
{
public int Id { get; set; }
public string Title { get; set; }
public string Description { get; set; }
public string[] Characters { get; set; }
public string Publisher { get; set; }
public bool Favorite { get; set; }
public string DisplayText
{
get
{
return Title + " (" + Publisher + ")";
}
}
}
}
2 Answers
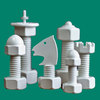
Steven Parker
229,783 PointsYou put a lot of code in there, but what the challenge asked for is way simpler. Remember, the challenge gave you two objectives:
- The GetVideoGames method shouldn't accept any parameters. — but you wrote it to accept an int named "id".
- The GetVideoGames method should return the _videoGames private static field. — but you wrote code to search for and return a specific item.
Your code looks a good deal like what will be needed for task 2. Did you perhaps overwrite your task 1 function with the code for task 2? The task 2 function should be added to the class below the one created for task 1 (and the name is slightly different).
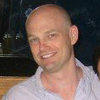
Noah Yasskin
23,947 Pointsusing Treehouse.Models;
namespace Treehouse.Data
{
public class VideoGamesRepository
{
// TODO Add GetVideoGames method
public VideoGame[] GetVideoGames()
{
return _videoGames;
}
// TODO Add GetVideoGame method
private static VideoGame[] _videoGames = new VideoGame[]
{
new VideoGame()
{
Id = 1,
Title = "Super Mario 64",
Description = "Super Mario 64 is a 1996 platform video game developed and published by Nintendo for the Nintendo 64.",
Characters = new string[]
{
"Mario",
"Princess Peach",
"Bowser",
"Toad",
"Yoshi"
},
Publisher = "Nintendo",
Favorite = true
},
new VideoGame()
{
Id = 2,
Title = "Mario Kart 64",
Description = "Mario Kart 64 is a 1996 go-kart racing game developed and published by Nintendo for the Nintendo 64 video game console.",
Characters = new string[]
{
"Mario",
"Princess Peach",
"Bowser",
"Toad",
"Yoshi"
},
Publisher = "Nintendo",
Favorite = false
}
};
}
}
Severin Haselmann
24,517 PointsSeverin Haselmann
24,517 PointsOh my....Didnt realize I need to add another method -.- . Thanks again Steven!
Saula Sautia
1,634 PointsSaula Sautia
1,634 PointsHi Steve,
Please what does the "return the _videoGames private static field" means?