Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial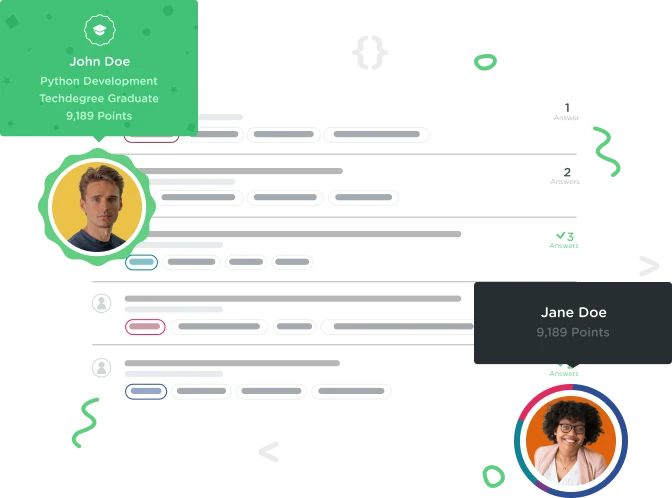

Dylan Gaffney
Courses Plus Student 4,781 PointsRequired prop 'id' was not specified in 'Player'. Check the render method of 'Application'.
Not sure what's wrong here. I've added 'id' to the player array objects, and have the following code declaring Player and Application.propTypes:
Player.propTypes = {
id: React.PropTypes.number.isRequired,
name: React.PropTypes.string.isRequired,
score: React.PropTypes.number.isRequired
};
Application.propTypes = {
title: React.PropTypes.string,
players: React.PropTypes.arrayOf(React.PropTypes.shape({
id: React.PropTypes.number.isRequired,
name: React.PropTypes.string.isRequired,
score: React.PropTypes.number.isRequired
})).isRequired
};
Application.defaultProps = {
title: "Scoreboard",
};
Here's the code rendering the Application component:
function Application(props) {
return (
<div className="scoreboard">
<Header title={props.title}/>
<div className="players">
{props.players.map(function (player){
return <Player name={player.name} score={player.score} key={player.id}/>
})}
</div>
</div>
);
}
From what I can tell, this is in line with the video. The "key" property is being passed to the player elements correctly in the DOM and is visible using the React dev tools, but I'm still getting this error.
2 Answers

Nicolai Rosdahl Tellefsen
4,215 PointsMy best guess is that you are telling React that the id property is a required property for your Player component:
Player.propTypes = {
id: React.PropTypes.number.isRequired,
name: React.PropTypes.string.isRequired,
score: React.PropTypes.number.isRequired
};
When generating each Player element in the player list your are passing name, score and key, but not an id property:
No "id=" property (your code):
return <Player name={player.name} score={player.score} key={player.id}/>
With an "id=" property:
return <Player name={player.name} score={player.score} key={player.id} id={player.id}/>
Since the Player component doesn't really need to know what id it has, I would just recommend that you just remove the id property from it's propTypes.
Player.propTypes = {
name: React.PropTypes.string.isRequired,
score: React.PropTypes.number.isRequired
};

Jeffrey James
2,636 PointsRemoving it as a Player proptype worked for me. Not sure why it would conceptually help this psuedo type checking strategy to begin with since it mainly supports their dom diffing algorithm and it just an seldom used arg available in the anon func that goes along with map(...)

Dylan Gaffney
Courses Plus Student 4,781 PointsThis worked! I believe the video had us adding id to the player propTypes as a required propType, but removing that requirement fixed the issue. To test this, I also passed in id to the player component (in addition to key), which fixed the error as well, despite the obvious redundancy. Thanks for your help!
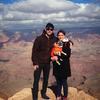
Daniel Crittenden
9,308 PointsDylan Gaffney - They must have updated the video, because it does not ask the viewer to add the ID to Player.proptype (only to Application.proptype). I made the same mistake initially, which is why I chimed in here.
Jennifer Nordell
Treehouse TeacherJennifer Nordell
Treehouse TeacherThis is probably going to be easiest to help you troubleshoot if we can get a snapshot to your workspace