Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial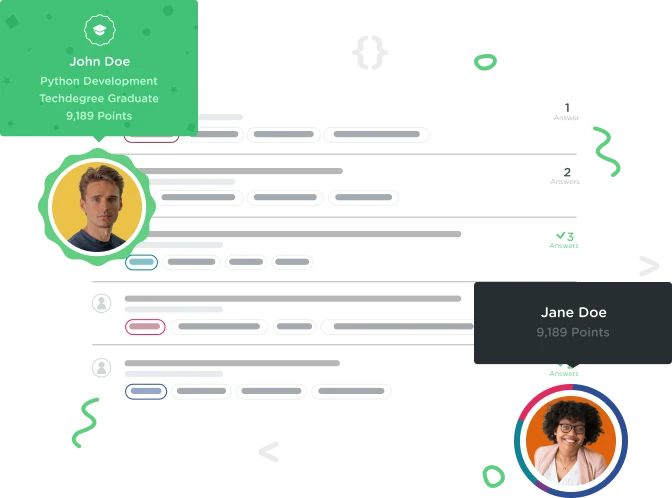

Nick Tolbert
12,003 Points[RESOLVED] am getting an error of Uncaught ReferenceError: song is not defined
can someone tell me why am getting this and how to correct it?
//song.js
function Song(title,artist,duration) {
this.title= title;
this.artist= artist;
this.duration = duration;
this.isplaying= false;
}
Song.prototype.play = function() {
this.isplaying= true;
};
Song.prototype.stop = function() {
this.isplaying = false;
};
Song.prototype.toHTML = function() {
var htmlString = '<li';
if(this.isplaying){
htmlString+=' class="current"';
}
htmlString+='>';
htmlString+=this.title;
htmlString+=' - ';
htmlString+=this.artist;
htmlString+='<span class="duration">';
htmlString+= this.duration;
htmlString+='</span></li>';
return htmlString;
};
//playlist.js
function Playlist() {
this.songs = [];
this.nowPlayingInex = 0;
}
Playlist.prototype.add = function(song) {
this.songs.push(song);
};
Playlist.prototype.play = function() {
var currentSong = this.songs[this.nowPlayingInex];
currentSong.play();
};
Playlist.prototype.stop = function(){
var currentSong = this.songs[this.nowPlayingInex];
currentSong.stop();
};
Playlist.prototype.next = function() {
this.stop();
this.nowPlayingInex++;
if(this.nowPlayingInex === this.songs.length){
this.nowPlayingInex= 0
this.play();
}else{
this.play();
};
};
Playlist.prototype.renderInElement = function(list) {
list.innerHTML="";
for(var i=0;i<Songs.length;i++){
list.innerHTML+= this.songs[i].tohtml();
}
};
//app.js
var Playlist = new Playlist();
var hereComesTheSun = new song("Here Comes The Sun","The beatles", "2:54");
var iGotAFeeling = new song("I Got a Feeling","The Beatles", "4:30");
Playlist.add(hereComesTheSun);
Playlist.add(iGotAFeeling);
var playlistElement = document.getElementById("playlist");
Playlist.renderInElement(playlistElement);
4 Answers

Chad Donohue
5,657 Points//song.js
function Song(title,artist,duration) {
this.title= title;
this.artist= artist;
this.duration = duration;
this.isplaying= false;
}
Song.prototype.play = function() {
this.isplaying= true;
};
Song.prototype.stop = function() {
this.isplaying = false;
};
Song.prototype.toHTML = function() {
var htmlString = '<li';
if(this.isplaying){
htmlString+=' class="current"';
}
htmlString+='>';
htmlString+=this.title;
htmlString+=' - ';
htmlString+=this.artist;
htmlString+='<span class="duration">';
htmlString+= this.duration;
htmlString+='</span></li>';
return htmlString;
};
//playlist.js
function Playlist() {
this.songs = [];
this.nowPlayingIndex = 0;
}
Playlist.prototype.add = function(song) {
this.songs.push(song);
};
Playlist.prototype.play = function() {
var currentSong = this.songs[this.nowPlayingIndex];
currentSong.play();
};
Playlist.prototype.stop = function(){
var currentSong = this.songs[this.nowPlayingIndex];
currentSong.stop();
};
Playlist.prototype.next = function() {
this.stop();
this.nowPlayingIndex++;
if ( this.nowPlayingIndex === this.songs.length ){
this.nowPlayingIndex= 0;
}
this.play();
};
Playlist.prototype.renderInElement = function(list) {
list.innerHTML="";
for ( var i = 0; i < this.songs.length; i++ ) {
list.innerHTML+= this.songs[i].toHTML();
}
};
//app.js
var playlist = new Playlist();
var hereComesTheSun = new Song("Here Comes The Sun","The beatles", "2:54");
var iGotAFeeling = new Song("I Got a Feeling","The Beatles", "4:30");
playlist.add(hereComesTheSun);
playlist.add(iGotAFeeling);
var playlistElement = document.getElementById("playlist");
playlist.renderInElement(playlistElement);

Chad Donohue
5,657 PointsYou need to capitalize 'Song' when you are assigning the object to a variable.
var hereComesTheSun = new Song(...);

Nick Tolbert
12,003 PointsI went through my code and change both song and songs to Song/Songs but now I have the same error in my for loop on line 35 of playlist.js
Playlist.prototype.renderInElement = function(list) {
list.innerHTML="";
for(var i=0; i< Songs.length; i++){
list.innerHTML+= this.Songs[i].tohtml();
}
};

Chad Donohue
5,657 PointsWhere is Songs declared in playlist.js?

Nick Tolbert
12,003 Pointsat the top of playlist.js inside the first function
function Playlist() {
this.Songs = [];
this.nowPlayingInex = 0;
}

Chad Donohue
5,657 PointsI think you want
for(var i=0;i<this.songs.length;i++){
list.innerHTML+= this.songs[i].tohtml();
}

Nick Tolbert
12,003 Pointsthank you for the help chad, after I add the missing this.Songs in the for loop I also needed to change tohtml() to toHML()

Chad Donohue
5,657 PointsGlad you figured it out :)
Chad Donohue
5,657 PointsChad Donohue
5,657 PointsI have changed some capitalizations and changed your call in the for loop to
toHTML()
.A good rule of thumb is to keep your class definitions capitalized and your variable declarations camel-cased.
This way, you know if you are dealing with a class definition or a variable.
Nick Tolbert
12,003 PointsNick Tolbert
12,003 Pointscan you give me an example of class definition ?
Chad Donohue
5,657 PointsChad Donohue
5,657 PointsClass definition
Using the class with a new instance
var hereComesTheSun = new Song("Here Comes The Sun","The beatles", "2:54");
Nick Tolbert
12,003 PointsNick Tolbert
12,003 PointsOK, I understand it now, this course is kinda fast pace ; I understand the concepts but there is a lot function grabbing from other function and I don't feel to confident in my ability to layout all the necessary functions. is there any resources that can help with to planing stage or dose this come with practice?
Chad Donohue
5,657 PointsChad Donohue
5,657 PointsIt's really a bit of both. Keep practicing and don't be afraid to ask questions like you already have.
As far as resources go, you should check out Eloquent JavaScript
Nick Tolbert
12,003 PointsNick Tolbert
12,003 Pointsok cool, and thanks again for all the help!