Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial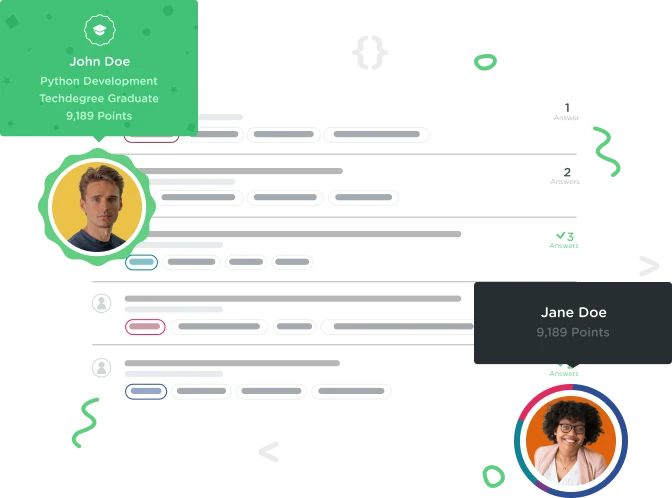

Tony Miri
6,508 Points[RESOLVED] Code Broke when I added the Ajax request.
Hello. I'm having some trouble finding the errors in my code. After adding the ajaxRequest function and adding the event listeners, when I preview the page, the add button no longer works at all. Also, when I try to add a task with the JavaScript console open there is no error code thrown. The functionality just doesn't work.
//Problem: user interaction doesn't provide desired results
//Solution: Add interactivity so the user can manage daily tasks
var taskInput = document.getElementById("new-task"); //new-task
var addButton = document.getElementsByTagName("button")[0]; //first-button
var incompleteTasksHolder = document.getElementById("incomplete-tasks"); //incomplete-tasks
var completedTasksHolder = document.getElementById("completed-tasks"); //completed-tasks
//New task list item
var createNewTaskElement = function(taskString){
//Create list item
var listItem = document.createElement("li");
//Input (checkbox)
var checkBox = document.createElement("input");
//Label
var label = document.createElement("label");
//Input (text)
var editInput = document.createElement("input");
//button.edit
var editButton = document.createElement("button");
//button.delete
var deleteButton = document.createElement("button");
//each element, needs modifying and appending
checkBox.type = "checkbox";
editInput.type = "text";
editButton.innerText = "Edit";
editButton.className = "edit";
deleteButton.innerText = "Delete";
deleteButton.className = "delete";
label.innerText = taskString;
listItem.appendChild(checkBox);
listItem.appendChild(label);
listItem.appendChild(editInput);
listItem.appendChild(editButton);
listItem.appendChild(deleteButton);
return listItem;
}
//Add a new task
var addTask = function() {
console.log("Add task...");
//Create a new list item with the text from #new-task:
var listItem = createNewTaskElement(taskInput.value);
//Append listItem to incompleteTasksHolder
incompleteTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
taskInput.value = "";
}
//Edit an Existing task
var editTask = function() {
console.log("Edit task...");
var listItem = this.parentNode;
var editInput = listItem.querySelector("input[type=text");
var label = listItem.querySelector("label");
var containsClass = listItem.classList.contains("editMode");
//if the class of the parent is .editMode
if(containsClass){
//Switch from editMode
//label text becomes input value
label.innerText = editInput.value;
} else {
//else
//Switch to editMode
//Input value becomes label's text
editInput.value = label.innerText;
}
//Toggle .editMode on the parent
listItem.classList.toggle("editMode");
}
//Delete an existing task
var deleteTask = function() {
console.log("Delete task...");
//remove the parent list item from the ul
var listItem = this.parentNode;
var ul = listItem.parentNode;
ul.removeChild(listItem);
}
//Mark a task as complete
var taskCompleted = function() {
console.log("Completed task...");
//append the task list item to #completed-tasks
var listItem = this.parentNode;
completedTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskIncomplete);
}
//Mark a task as incomplete
var taskIncomplete = function() {
console.log("Incomplete task...");
//append the task list item to #incomplete-tasks
var listItem = this.parentNode;
incompleteTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
}
var bindTaskEvents = function(taskListItem, checkBoxEventHandler){
console.log("Bind list item events");
//select taskListItem's children
var checkBox = taskListItem.querySelector("input[type=checkBox]");
var deleteButton = taskListItem.querySelector("button.delete");
var editButton = taskListItem.querySelector("button.edit");
//bind editTask to edit button
editButton.onclick = editTask;
//bind deleteTask to delete button
deleteButton.onclick = deleteTask;
//bind checkBoxEventHandler to checkbox
checkBox.onchange = checkBoxEventHandler;
}
var ajaxRequest = function() {
console.log("AJAX Request");
}
//Set the click handler to the addTask function
addButton.addEventListener = ("click", addTask);
addButton.addEventListener = ("click", ajaxRequest);
//cycle over the incompleteTasksHolder ul li items
for (var i = 0; i < incompleteTasksHolder.children.length; i++) {
bindTaskEvents(incompleteTasksHolder.children[i], taskCompleted);
}
//for each list item
//bind events to list item's children (taskCompleted)
//cycle over the completedTasksHolder ul li items
for (var i = 0; i < completedTasksHolder.children.length; i++) {
bindTaskEvents(completedTasksHolder.children[i], taskIncomplete);
}
//for each list item
//bind events to list item's children (taskIncomplete)
Tony Miri
6,508 PointsTony Miri
6,508 PointsNevermind. I found it.
Simple syntax error. I should not have had the "=" after the addEventListener.
It's always the simplest thing.