Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial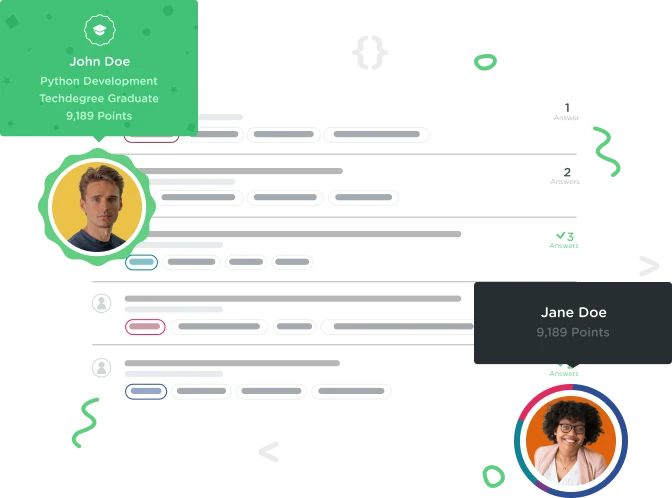

Jerome Obba
7,384 PointsResource(s) on javascript asynchronous programing that explains callbacks for users with at a novice level.
I have a beginner level knowledge of javascript ( I know about functions, loops, objects and a little json). I went to Amazon.com to look for books, but the reviews don't recommend them for relative newbies, but for experienced programmers. I went onto the web, and found a few isolated articles. What I'm looking for is either a book or a mini course that starts simply, explains the concepts and uses many easy to follow examples to reinforce the knowledge. I'm struggling with these concepts, and would like to consolidate it, thank you in advance.
5 Answers

Aaron Martone
3,290 PointsTry the TeamTreehouse course on understanding JavaScript Promises. They are the epitome of asynchronous handling. For example:
// If we did things synchronously (only 1 thing happens at a time)
// the moment we performed an action that took time, like loading
// data, accessing network resources, reading information, etc.,
// JavaScript would stop moving to the next line until the synchronous
// task is done. Some devs think this is ideal, but it's not. It wastes time
// and JS is bound to the same thread that renders UI updates in your
// browser. This means that while JS does it's sync task, you cannot
// scroll, click, move the mouse, etc. That's why this is known as
// "blocking". That's why async (doing more than 1 thing at a time) is
// important. And for that, we use Promises
var myPromise = new Promise(function(resolve, reject) {
// Here I simulate a task that takes time, but making this function
// run after 4 seconds.
setTimeout(function() {
// When data comes back, we pass it in as the arg to our resolve func.
// In this case, the data comes back (the number 20). This value is
// passed to our .then() method below as the 'data' variable. This
// sequential hand off of an async event allows us to be sure that the
// value has been resolved and data exists.
resolve(20);
}).then(function(data) {
console.log('Our data is ' + data); // Outputs 'Our data is 20'
});
Compare this to a synchronous task:
var data = functionThatGetsDataButTakesTime();
// Because the function does something synchronously, it returns a value
// of undefined and JS continues to move on to the next code. As long as
// the task does not block JS, it continues to the next line.
console.log('Our data is ' + data); // Outputs 'Our data is undefined'
Moments later, the synchronous task resolves to 20, and data
is updated accordingly, but no matter, our code has already gone past where it expected to use data
(which at the time was undefined
) and we've lost the benefit of coding asynchronously.

Aaron Martone
3,290 PointsIt's a tough road. Many resources on JS are sporadic and not courses designed to teach things sequentially where one builds on the thing before it. I've suffered from reading up on blog posts online only to hear them speak of tech or processes I was not familiar with, making comprehension very difficult.
Getting an understanding of event-driven programming would be very helpful. Events, by their very nature, are asynchronous. They can happen at anytime. Since JS is 1 thread, usually, how we bind handlers (the functions that process those events) are where synchronous programming ends up blocking us or limiting how powerful JS can be.
I wish I could recommend a book or resource. Kyle Simpson offers an amazing series called "You Don't Know JS" in a 6-part small book series that I HIGHLY recommend. One of the later books is about Async, but you might want to read them in sequence to make sure you have a solid understanding of concepts that the book may reference.

Jerome Obba
7,384 PointsAaron, many thanks for taking the time to explain this. I read it a couple of times (to understand more fully what was being said). If nothing else, I realise I will have to get to grips with asynchronous programming - that much is very clear.
Did you start off with asynchronous programming from taking the 'promises' course? If not where did you get your firsst useful lessons from?
I liked the way you explained the difference between 'callback' and 'callback()'. I understood the distinction, (after you explained it). I appreciate your time in wanting to share your knowledge with me. Thank you very much.

Aaron Martone
3,290 PointsGlad you understood the part about functions. People usually forcibly see them always with their parens, not understanding that the parens merely invoke the function.
function abc() { return 'Hi'; }
console.log(abc); // outputs 'function abc() { return 'Hi'; }', since 'abc' is a variable that points to the function statement.
console.log(abc()); // outputs 'Hi', which is the same thing, 'abc' (thus pointing to, and returning the function', but then seeing '()' after, so it executes, and evaluates to the returned value, 'Hi', which is then passed to the 'console.log()' method for output.
In this way:
function abc() { /* ... */ }
// is like
var abc = function() { /* ... */ };
The former is known as 'function declaration' syntax, and the latter is known as 'function expression' syntax.

Jerome Obba
7,384 PointsThanks for the recommendation, this doesn't sound like a quick fix, but more of medium term approach with that series. Is it pitched at those with basic understanding or those who are considered to be 'intermediate' level?

Aaron Martone
3,290 PointsIf you go sequentially through the books, Kyle makes no assumption about your level of understanding with JS. So it's a great read for new-intermediate-experienced. The great thing about his series is that he wants you to understand HOW JS does what it does, not just the end result, so that you can apply the comprehension to your workflow.
In my experience, too many people regurgitate JS in their tutorials without explaining the how's or why's. Those are the important questions, and those are the answers that Kyle gives in his book. He has a Github repo where all 6 books are free to read in full, but I HIGHLY recommend buying them from his publisher if you like them.

Jerome Obba
7,384 PointsYour recommendation seems sound and I will more than likely check out his books, as you have an idea of where I'm at and where my shortcomings are and how these books can plug that knowledge gap. Thank you Aaron, these posts have helped me a lot.
Aaron Martone
3,290 PointsAaron Martone
3,290 PointsThey're hard to explain. Basically, a callback is a function that executes when a process is complete. Take this example