Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial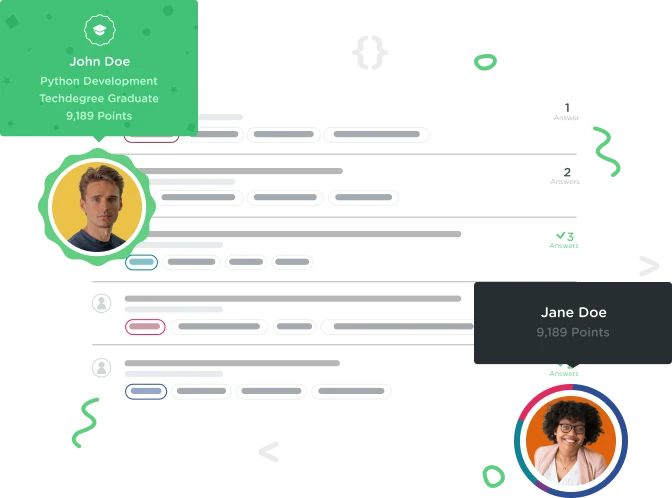

Greg Schudel
4,090 PointsResponding to the syntax
/* I have questions on each piece */
function printList( list /*why does this have to have it's own unique name?*/ ){
var listHTML = '<ol>'; /*I get this!*/
for (var i = 0; i < list.length; i += 1){
listHTML += '<li>' + list[i] /*Is this another array from the above value?? */ + '</li>';
}
listHTML += '</ol>'; /* how can this be declared twice like this with two different values and not give an error? how can you declare it without a var at the beginning?*/
print(listHTML); /*What's the difference between this and document.write? Why do some devs say "document.write" is bad to use in a browser? I cannot use the document.write method in JS Fiddle for warnings about breaking it, I know that's not your tech, but any idea why. */
}
printList(playList);
/*The whole purpose of involving a for loop in "printing" this out is to allow this data to be dynamic from user interaction, yes (hence why javascript is for "behavior of a website", yes?)? How can you tell when something is printed out from JS vs something that is just static HTML from the Chrome dev tools?* /
2 Answers
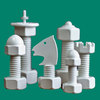
Steven Parker
230,274 PointsI'll try to address each question.
function printList( list /*why does this have to have it's own unique name?*/ ){
Any argument must have a name so that you can reference it inside the function.
listHTML += '<li>' + list[i] /*Is this another array from the above value?? */ + '</li>';
list[i]
is one of the elements from the array that was provided as the function argument.
listHTML += '</ol>'; /* how can this be declared twice like this with two different values and not give an error? how can you declare it without a var at the beginning?*/
This is not a declaration. The variable listHTML which was previously declared is being appended to here.
print(listHTML); /*What's the difference between this and document.write?
Though not shown in your code, I believe that the print function is defined elsewhere in this exercise and does in fact call document.write. Using a local function makes it easy to potentially change how the output is displayed with only minimal code modification later.
- Why do some devs say "document.write" is bad to use in a browser?
When improperly used, document.write can cause unintentional erasure of page contents, or it may delay the initial rendering of the page.
- I cannot use the document.write method in JS Fiddle for warnings about breaking it, I know that's not your tech, but any idea why.
You could store your Fiddle and share the link to it to facilitate further analysis. But my first guess would be that their page is vulnerable to the content erasure that I mentioned previously.
- How can you tell when something is printed out from JS vs something that is just static HTML ...
Unless you intentionally provide a method of distinction in your code, there won't be any difference between the appearance of static HTML elements and ones that are created by the JavaScript code.
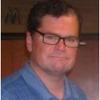
Mark Wilkowske
Courses Plus Student 18,131 PointsThink of variables as blocks in memory. With the var keyword you are telling the JavaScript interpreter to open up some memory for the actual content of the variable be it string, decimal, array, whatever. After that the variable is referenced without the var keyword because the variable is already known to the interpreter.
You can better understand JS variables by reading from right to left so this:
listHTML += '<ol>';
Really means a <ol> start tag is now the contents of a variable (a space in memory) known as listHTML.
The script executes from top to bottom so in this code the content of listHTML goes through three changes:
first it is an <ol> start tag
then it wraps <li></li>'s around each array item from 'list' - looping until done
and finally it is an </ol> closing tag.
Greg Schudel
4,090 PointsGreg Schudel
4,090 PointsWOW! Soooooo much more clear!!!
So should I use "document.write" or "print" going forward to write to the DOM?
What do you mean by "method of distinction"? You mean inline comments?
how do you tag code text in this editor(I have yet to find that out)? For example, do I use "<code></code>" tags?
Steven Parker
230,274 PointsSteven Parker
230,274 Pointsprint is not a JavaScript function. It is implemented somewhere in the parts of the code you did not show. As I mentioned before, I believe it uses document.write internally. But in later lessons you will learn even better ways to create and alter DOM elements.
The method of distinction would be your choice. You could make the created code look different by using a different color, or font, or font size, or with bold weight or italics, etc.
For posting code here in the forum use the instructions provided in the Markdown Cheatsheet pop-up found below the "Add an Answer" area.