Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial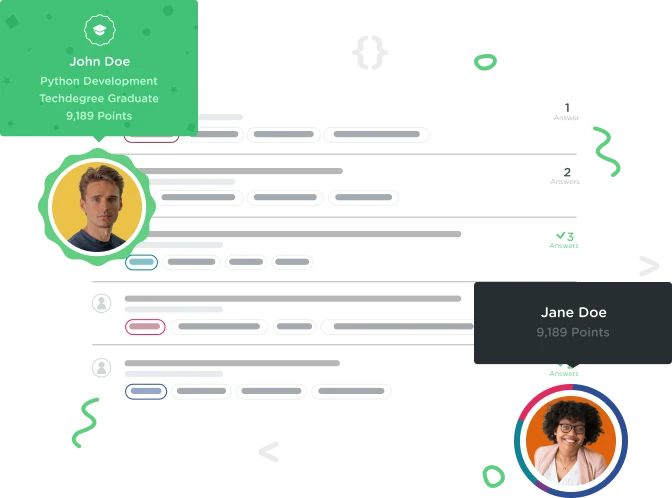
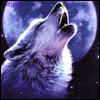
Daniel Hernandez
13,437 Pointsres.redirect won't work and main website won't display typed cookie username
I have run into two problems. One is that res.redirect won't work, after submitting the form, the page stays at "/hello". And also my main page will not display the typed username, instead displaying absolutely nothing where the variable "name" is. Here is my code. Thanks ahead for any help you guys can give.
Here is the app.js file.
const express = require('express');
const bodyParser = require('body-parser');
const cookieParser = require('cookie-parser');
const app = express();
app.use(bodyParser.urlencoded({extended: false }));
app.use(cookieParser());
app.set('view engine', 'pug')
app.get('/', (req, res) =>{
const name = req.cookies.username;
res.render("index" , { name});
});
app.get('/cards', (req, res) =>{
res.render('card', { prompt: "Who is buried under Grant's Tomb"});
});
app.get('/hello', (req, res) =>{
res.render('hello');
});
app.post('/hello', (req, res) =>{
res.cookie('username', req.body.username);
res.redirect('/');
});
app.listen(3001, ()=> {
console.log('The application is running on port 3000')
});
And here is the index.pug file
extends layout.pug
block content
section#content
h2 Welcome,#{name}!
1 Answer

Kevin Gates
15,053 PointsFor the redirect, the console for me said I needed to change what I sent, namely that I needed a status code. So I'm sending this now:
app.post('/hello', (req, res) => {
res.cookie('username', req.body.username);
res.redirect( '/', 302, {name: req.cookies.username});
});
The whole answer is: app.js
const express = require('express');
const bodyParser = require('body-parser');
const cookieParser = require('cookie-parser');
const app = express();
app.use(bodyParser.urlencoded({extended: false}));
app.use(cookieParser());
app.set('view engine', 'pug');
app.get('/', (req, res) => {
const name = req.cookies.username;
res.render('index', {name});
});
app.get('/hello', (req, res) => {
res.render('hello');
});
app.post('/hello', (req, res) => {
res.cookie('username', req.body.username);
res.redirect( '/', 302, {name: req.cookies.username});
});
app.get('/cards', (req, res) => {
res.locals.prompt = "Who is buried in Grant's tomb?";
res.render('card', {});
});
app.listen(3000, ()=> {
console.log('The application is running on localhost:3000!');
});
Which it does work for me now.
Presuming you updated index.pug to
extends layout.pug
block content
section#content
h2 Welcome, #{name}!
Travis Lloyd
9,479 PointsTravis Lloyd
9,479 PointsLooks like you accidentally set app.listen to port 3001 instead of 3000. Hope that helps! Cheers!