Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial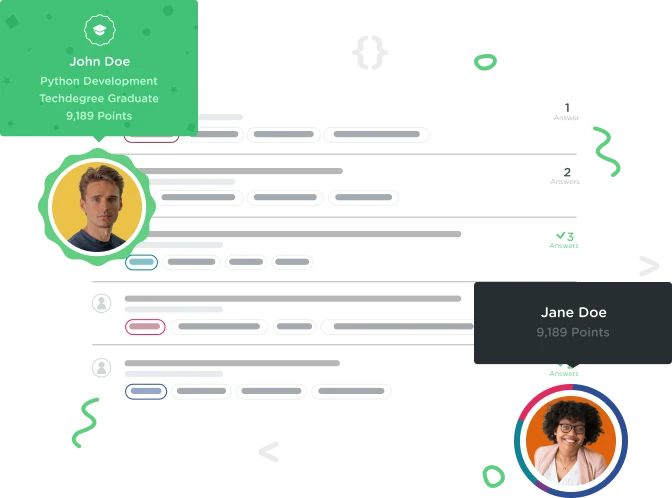

Nick Burton
7,387 Pointsres.render passing in object vs res.render.locals variables?
So to my understanding there are (at least) 2 ways of passing data into your views. 1 - passing in an object into your res.render method. 2 - assigning the value to the res.locals object Are there certain situations where one method of passing in data is preferred over the other? How do I know which approach is best? Or is it just preference? Thank you!
1 Answer

Brian Anstett
5,831 PointsHey Nick, I think probably at the end of the day it just comes down to preference. That being said there are some difference and definitely some use cases where you would want to res.locals over just passing in an object to res. render().
The properties added to the res.locals object persist through the entire request/response cycle meaning you could assign a value in a middleware function somewhere and then access it in another middleware function later or in PUG. If you haven't talked about middleware functions yet this may not make that must sense to you but if you have, let's look at this example.
File Structure:
views/example.pug
app.js
app.js Javascript File
const express = require('express');
const app = express();
app.set('view engine', 'pug');
//Route for 'example'
//Call the middleware function first, then go to my anonymous function
app.use('/example', middleware, (req, res, next)=>{
console.log(res.locals.secret);
return res.render('example', {
prompt: "Hello World!"
})
})
//Define a middleware function
function middleware(req, res ,next){
res.locals.secret = 'SuperSecret';
return next();
}
app.listen(3000, ()=>{
console.log("listening on port 3000");
});
example.pug pug file
html
body
h1= secret
h1= prompt
console output
listening on port 3000
SuperSecret
Let's walk through this now. 1) We define a middleware function called 'middleware' which assigns the value 'SuperSecret' to the secret property of the res.locals object. The function then calls the next() method telling express we're ready to move to the next middleware function, in this case our anonymous function. 2) After the first middleware function completes ( next() ) our anonymous function gets called. In this function we print to the console the value res.locals.secret which was defined in our middleware function. We then render our example pug file. 3) In our pug file we have two headers, one that prints our prompt ('Hello World!') and the other prints the value secret which was defined in our first middleware function.
So in conclusion, we can use res.locals to "pass" values between our middleware functions and sort of "append" to the res.locals object as we move through the request/response cycle. Also notice that we can define a value in one function and render a pug template in another and PUG still can access that res.locals value. Pretty Cool!