Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial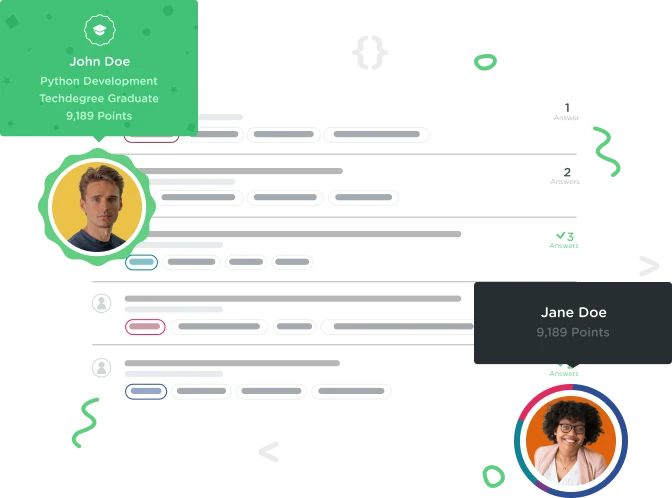

John Brady
Full Stack JavaScript Techdegree Student 14,013 PointsResults work on regexpal.com, but not on the quiz.
This one appears to work pretty well on regexpal.com. It limits the results to the # sign plus 6 alphanumeric characters. I also put the flag at the end for case insensitivity. Like this:
/#[A-Z0-9]{6}/i
Not sure why it doesn't pass, the quiz feedback says, "Bummer: ... see if you can limit the regex to narrow the results ..."
So narrowing it down might be limiting the length of the string so that there are no more possible characters than 7 by adding the $ at the end. Adding a carrot ^ at the beginning would ensure that the string starts with a # character. To my surprise this doesn't work on the quiz or regexpal.com:
/^#[A-Z0-9]{6}$/i
So I'm stuck here. Help is appreciated.
// Type inside this function
function isValidHex(text) {
const hexRegEx = /#[A-Z0-9]{6}/i
}
const hex = document.getElementById("hex");
const body = document.getElementsByTagName("body")[0];
hex.addEventListener("input", e => {
const text = e.target.value;
const valid = isValidHex(text);
if (valid) {
body.style.backgroundColor = "rgb(176, 208, 168)";
} else {
body.style.backgroundColor = "rgb(189, 86, 86)";
}
});
<!DOCTYPE html>
<html>
<head>
<title>DOM Manipulation</title>
</head>
<link rel="stylesheet" href="style.css" />
<body>
<div id="content">
<p>Enter a valid hex value below to make the screen turn green.</p>
<input type="text" id="hex">
</div>
<script src="app.js"></script>
</body>
</html>
1 Answer
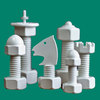
Steven Parker
231,275 PointsAs you discovered, it's necessary to restrict both the size and character set.
And it does work in regexpal if you are careful to make sure that the test string is a correct match (and has no extra white space or newlines). If you were trying to test it with multiple lines of matches, you'd need to add the global (g) and multiline (m) flags, which are not on by default.

John Brady
Full Stack JavaScript Techdegree Student 14,013 PointsThanks, I put in the (m) for multiline and that did the trick.
John Brady
Full Stack JavaScript Techdegree Student 14,013 PointsJohn Brady
Full Stack JavaScript Techdegree Student 14,013 PointsAnswered my own question ... This passed the quiz when I changed A-Z to A-F and then put the ^ and $ at the end.
However, for some reason it doesn't show that this solution (with the carrot at the beginning and dollar sign at the end) works on regexpal.com.