Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial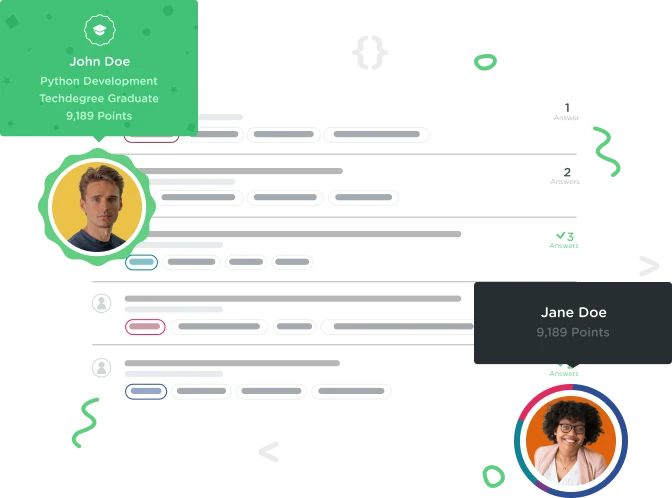

Diego Marrs
8,243 PointsRetrieve Object From Listener
Hello.
So the scenario here is that I have an activity that has an ArrayList that I want to display in a DialogFragment. I used an interface to deliver the data from the activity to the DialogFragment:
@Override
public void addListClickListener(View v, ArrayList<ListObject> objects, ListsAdapter adapter) {
mObjects = objects; //Delivering the data to the fragment
mListsAdapter = adapter;
mNewListFragment.setListener(mListener5);
mNewListFragment.setListener1(mListener6);
mTodoListsFragment.dismiss();
mNewListFragment.show(getSupportFragmentManager(), NEW_LIST_FRAGMENT);
}
Then in my fragment I display, or at least try to, the data from the interface to the ListAdapter:
public interface AddListClickListener {
void addListClickListener(View v, ArrayList<ListObject> objects, ListsAdapter adapter);
}
mAddListImage.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
mListener.addListClickListener(v, mObjects, mListsAdapter);
}
});
protected void RecyclerViewSetup() {
mListsAdapter = new ListsAdapter(mListener1, mListener2, getActivity(), mObjects);
mListsAdapter.registerAdapterDataObserver(new RecyclerView.AdapterDataObserver() {
@Override
public void onChanged() {
super.onChanged();
}
@Override
public void onItemRangeChanged(int positionStart, int itemCount) {
super.onItemRangeChanged(positionStart, itemCount);
}
@Override
public void onItemRangeChanged(int positionStart, int itemCount, Object payload) {
super.onItemRangeChanged(positionStart, itemCount, payload);
}
@Override
public void onItemRangeInserted(int positionStart, int itemCount) {
super.onItemRangeInserted(positionStart, itemCount);
}
@Override
public void onItemRangeRemoved(int positionStart, int itemCount) {
super.onItemRangeRemoved(positionStart, itemCount);
}
@Override
public void onItemRangeMoved(int fromPosition, int toPosition, int itemCount) {
super.onItemRangeMoved(fromPosition, toPosition, itemCount);
}
});
RecyclerView.LayoutManager layoutManager = new LinearLayoutManager(getActivity());
mRecyclerView.setLayoutManager(layoutManager);
mRecyclerView.setHasFixedSize(true);
mRecyclerView.setAdapter(mListsAdapter);
checkAdapterIsEmpty();
However, I also need to define the mObjects array in the fragment without recreating itself every time the user opens the DialogFragment. How would I do this?
Thank you.
2 Answers

Ben Deitch
Treehouse TeacherHey Diego! You could try making the ArrayList static in your Activity. Just make sure it gets populated before you launch the DialogFragment.

Elikem Kuivi
12,024 PointsI think there are two ways you could do this:
- (Option 1) One way is to send the ArrayList as an argument to your Fragment, and retrieve it in the Fragment. However, to do this you will have to make the object in your ArrayList parcelable. To make your object Parcelable, take a look at this helpful guide to do that http://guides.codepath.com/android/Using-Parcelable. Once your object is Parcelable all you need to do is send it to the Fragment as an argument. For example:
In your Activity:
Bundle args = new Bundle();
args.putParcelableArrayList("key", mObjects);
mNewListFragment.setArguments(args);
...
In your Fragment:
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
...
ArrayList<ListObject> mObjects = new ArrayList<>();
Bundle args = getArguments();
if(args != null) {
mObjects = args.getParcelableArrayList("key");
}
...
}
- (Option 2) I created a small Android utility class that provides a simple way to pass objects between Fragments and Activities. It works pretty much in the same way as you would use Intent Extras to pass a String between Activities. The link to it is (https://gist.github.com/kkuivi/161f254374a4007ae6014f67ae45789e). To use it, first copy the class into your project and then:
In your Activity
ReferenceMap.getInstance().putObjectReference(NEW_FRAGMENT.class, "myKey", mObjects);
In your fragment
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
...
ArrayList<ListObject> mObjects = new ArrayList<>();
ReferenceMap.ReferenceList refList = ReferenceMap.getInstance().getReferenceList(this);
if(refList != null) {
mObject = (ObjectType) refList.get("myKey");
}
...
}
@Override
public void onStop(){
ReferenceMap.getInstance().releaseRefList(this);
super.onStop();
}
Let me know if this helps
Diego Marrs
8,243 PointsDiego Marrs
8,243 PointsWhat if the data is user-entered in my case? There is a button in another fragment that adds items to the list:
'createListListener' code in MainActivity:
Ben Deitch
Treehouse TeacherBen Deitch
Treehouse TeacherI think you'd still be fine with a static ArrayList.
Diego Marrs
8,243 PointsDiego Marrs
8,243 PointsI get an error:
MainActivity error:
mObjects.add(mListObject);
NewListDialogFragment error:
mListener1.createListListener(v, item, mListObject);
The mObjects ArrayList in my TodoListsFragment is still unassigned too.
Ben Deitch
Treehouse TeacherBen Deitch
Treehouse TeacherAh yep. You'll need to initialize the ArrayList when you declare it:
ArrayList mListObject = new ArrayList();
Diego Marrs
8,243 PointsDiego Marrs
8,243 PointsBut if I initialized mObjects in my TodoListFragment, it would override the previous one every time I reopen the fragment (which is why I'm creating the list, that I want to display in the DialogFragment, in the MainActivity). I made my mObjects list in my MainActivity static and initialized the other mObjects list in my DialogFragment and that's whats happening.
Ben Deitch
Treehouse TeacherBen Deitch
Treehouse TeacherHmm, maybe I'm not understanding it correctly. Is the project on Github?
Diego Marrs
8,243 PointsDiego Marrs
8,243 PointsI'll put it on GitHub as soon as I polish a few things.
Diego Marrs
8,243 PointsDiego Marrs
8,243 PointsThe project is up: https://github.com/StaticCloud/Unfinished_ATM
Ben Deitch
Treehouse TeacherBen Deitch
Treehouse TeacherHave you thought about storing your list as a SharedPreference? That way you could access it from anywhere relatively easily.
Diego Marrs
8,243 PointsDiego Marrs
8,243 PointsHmm...not entirely sure how to do that...at least with lists.