Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial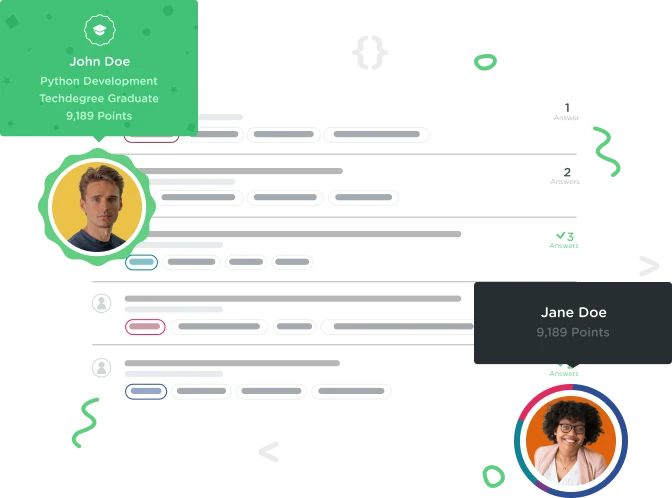
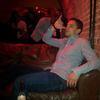
Michael Jones
Python Development Techdegree Graduate 38,554 PointsRetrieving a value from array inside of a dictionary.
Hey Coders,
I want to look inside of an Array, which is an value of a dictionary and return the key. So the dictionary would look something like this -
let carDictionary = [
"Cars" : ["Car 1", "Car 2", "Car 3"]
]
I'd like to know how to retrieve the 1st value from the array and return the key.
Hope someone can help me.
Thanks, Michael J
1 Answer

Anjali Pasupathy
28,883 PointsTo retrieve the first value of the array, you need to retrieve the array from the dictionary, and then retrieve the element at index 0. Because carDictionary returns an Optional when you retrieve the array, you need to unwrap the optional before you access the array.
// FORCE UNWRAP - NOT RECOMMENDED UNLESS YOU KNOW FOR SURE THE DATA WILL BE THERE
let firstCarForceUnwrapped = carDictionary["Cars"]![0]
// SAFE UNWRAPPING OF OPTIONAL WITH IF LET STATEMENT
if let carArray = carDictionary["Cars"] {
let firstCarIfLetUnwrapped = carArray[0]
// Can access first car inside this statement
}
// Can't access first car outside if let statement
// SAFE UNWRAPPING OF OPTIONAL WITH GUARD LET STATEMENT
guard let carArray = carDictionary["Cars"] else {
// Use control flow statement to exit scope - return, break, continue, throw, etc.
// Can't access first car inside of else block of guard let statement
}
let firstCarGuardLetUnwrapped = carArray[0]
If you want to see more about using Optionals and Optional unwrapping, check out this course: https://teamtreehouse.com/library/swift-20-enumerations-and-optionals
I hope this helps!