Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial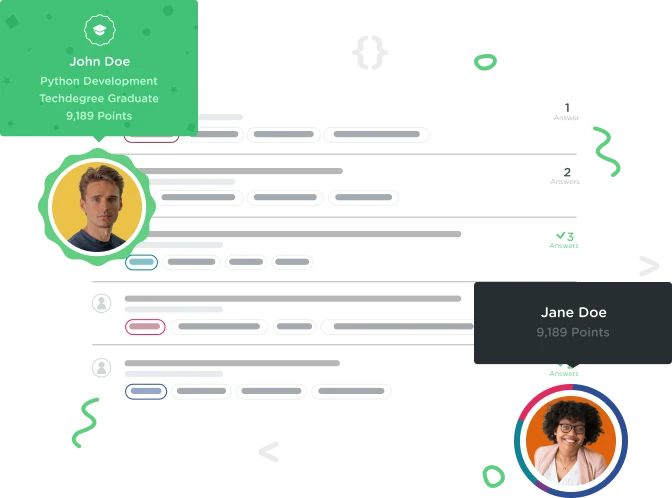
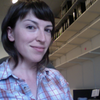
Margaret James
9,444 PointsRetrieving removed variable for New Array
var orderQueue = ['1XT567437','1U7857317','1I9222528'];
var shipping = [];
var firstItem = orderQueue.shift();
shipping.push(firstItem);
I'm in the Code Challenge and not sure why the 'firstItem' variable I created isn't functioning when I try to use it to add to my new 'shipping' array . What am I missing?
My error is "It doesn't look like you stored the first item from the array in the shipping variable."
Am I able to use the array storing property as the value of a new variable? Or am I just executing it incorrectly?
1 Answer
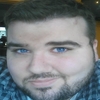
Marcus Parsons
15,719 PointsHi Margaret,
Your first code is technically correct and works as expected. But for challenges, you should only do what you're asked to do. You're only asked to create one variable, not two, so that's why the challenge won't let you pass.
Also, in your 2nd set of code, even though this works, too, it's unnecessary to include the brackets because the shift
method returns an array (in circumstances such as this) so you don't need to explicitly define it as an array by using brackets.
This is the simplest solution:
var orderQueue = ['1XT567437','1U7857317','1I9222528'];
var shipping = orderQueue.shift();

Iain Simmons
Treehouse Moderator 32,305 PointsActually Marcus Parsons, shift
returns the value of the removed element, not an array containing the value, so you would need to include the square brackets, or declare the variable as an empty array and then push
to it.
Alternatively, you could use the splice
Array method, which does return an array of any removed elements (and can also be used to simultaneously add elements to the array):
var orderQueue = ['1XT567437','1U7857317','1I9222528'];
var shipping = orderQueue.splice(0, 1); // start at index 0, remove 1 item
Margaret James
9,444 PointsMargaret James
9,444 PointsAlso - technically I don't understand why this alternate solution isn't working either.
P.S. I know the simple way of doing this, just curious about the transitive properties of array variables.