Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial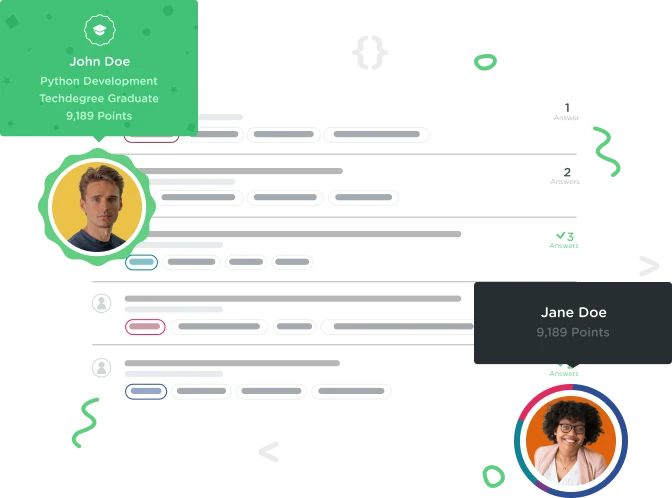

Aleks Dahlberg
19,103 PointsRetrieving weather data from Dark Sky API instead of user data from Treehouse
I am stuck trying to retrieve weather data from the Dark Sky API using longitude and latitude instead of using the treehouse JSON from this video. Unsure what to do. (Check bottom for hardcoded attempt)
Here is my code.
weather.js
var EventEmitter = require("events").EventEmitter;
var https = require("https");
var util = require("util");
var apiKey = "SECRETAPIKEYIOIOIOIOIOIO";
//get data from API
function Weather(weather) {
EventEmitter.call(this);
weatherEmitter = this;
//connect to the dark sky api
var request = https.get("https://api.forecast.io/forecast/" + apiKey + weather +".json", function (response) {
var body = "";
if (response.statusCode !== 200) {
request.abort();
//status code error
//weatherEmitter.emit("error", new Error("There was an error retreiving the weathe for that location. Location: " weather + ". (" + https.statusCode + ")"));
}
//read the data
response.on('data', function (chunk) {
body += chunk;
weatherEmitter.emit("data", chunk);
});
response.on('end', function () {
if (response.statusCode === 200) {
try {
//parse the data
var weather = JSON.parse(body);
console.dir(weather);
weatherEmitter.emit("end", weather);
} catch (error) {
weatherEmitter.emit("error", error);
}
}
}).on("error", function (error) {
weatherEmitter.emit("error", error);
});
});
}
util.inherits( Weather, EventEmitter);
module.exports = Weather;
router.js
/*Route file*/
var Weather = require("./weather.js");
/* Handle HTTp route GET / and POST / i.e Home */
function homeRoute(request, response) {
//if url == "/" && GET
if (request.url === "/"){
//show serach field
response.writeHead(200, {'Content-Type': 'text/plain'});
//set something to happen on an interval
response.write("Header\n");
response.write("Search\n");
response.end("Footer\n");
}
setInterval(function () {
console.log('this is before the end ');
}, 5000);
//if the URL == "/" && POST
//redirect to /:long,lat
}
/* Handle HTTP route GET /:longitude,latitude */
function weatherRoute(request, response) {
//if url == "/...."
var weather = request.url.replace("/","");
if(weather.length > 0){
response.writeHead(200, {'Content-Type': 'text/plain'});
response.write("Header\n");
//get json from darksky
var weatherProfile = new Weather(weather);
//on 'end'
weatherProfile.on("end", function (weatherJSON) {
//show weather info
//store the values we need
var values = {
hourly_summary: weatherJSON.hourly.summary,
currently_temperature: weatherJSON.currently.temperature,
daily_summary: weatherJSON.daily.summary
}
//simple response
response.write(values.weather + " is " + values.hourly_summary + "\n");
response.end("Footer\n");
});
//on 'error'
weatherProfile.on("error", function (error) {
console.console.error();
response.end("Footer\n");
//show error
});
}
}
/*export routes*/
module.exports.home = homeRoute;
module.exports.weather = weatherRoute
app.js
var router = require("./router.js");
/*Get Date time*/
function getSeconds() {
var date = new Date()
var seconds = date.getSeconds();
return seconds
}
/* Create server */
var port = 20000;
var http = require("http");
http.createServer(function (request, response) {
router.home(request, response);
router.weather(request, response);
}).listen(port);
console.log('Server is running on port ' + port);
I can retrieve hard coded data by changing this (in weather.js):
....
//connect to the dark sky api
var request = https.get("https://api.forecast.io/forecast/" + apiKey + weather
.....
to this (Adding coordinates in manually):
....
//connect to the dark sky api
var request = https.get("https://api.forecast.io/forecast/" + apiKey + "/45.5555,45.555",
.....
I want to be able to eventually type in the url address bar localhost:3000/[longitude],[latitude]
1 Answer

Seth Kroger
56,413 PointsI think the problem is you were forgetting to put the slash between the apiKey and the coordinates.
Aleks Dahlberg
19,103 PointsAleks Dahlberg
19,103 PointsThanks a lot for pointing that out!