Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial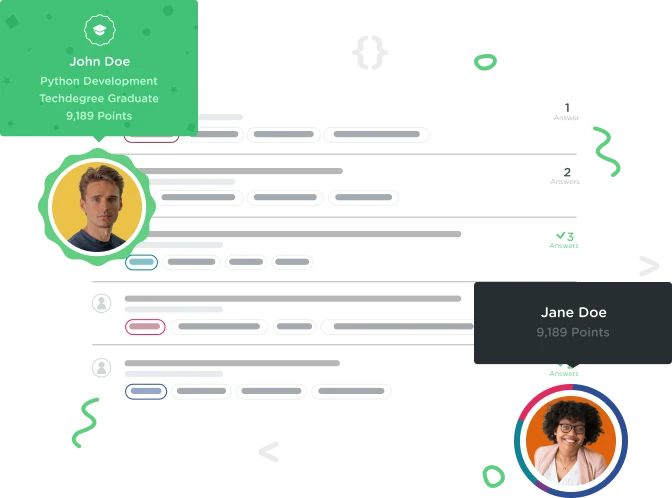

Ryu Blunder
Courses Plus Student 605 PointsReturn Condition
idk if the condition i've set is wrong, i can't figure out how to set up the code to make this true.
//Problem: Hints are shown even when form is valid
//Solution: Hide and show them at appropriate times
var $password = $("#password");
var $confirmPassword = $("#confirm_password");
var $username = $("#username");
//Hide hints
$("form span").hide();
function isUsernamePresent() {
if ($(username > 0));
return true;
}
function isPasswordValid() {
return $password.val().length > 8;
}
function arePasswordsMatching() {
return $password.val() === $confirmPassword.val();
}
function canSubmit() {
return isPasswordValid() && arePasswordsMatching();
}
function passwordEvent(){
//Find out if password is valid
if(isPasswordValid()) {
//Hide hint if valid
$password.next().hide();
} else {
//else show hint
$password.next().show();
}
}
function confirmPasswordEvent() {
//Find out if password and confirmation match
if(arePasswordsMatching()) {
//Hide hint if match
$confirmPassword.next().hide();
} else {
//else show hint
$confirmPassword.next().show();
}
}
function enableSubmitEvent() {
$("#submit").prop("disabled", !canSubmit());
}
function usernameEvent() {
if(isUsernamePresent()) {
$username.next().hide();
} else {
$username.next().show();
}
}
//When event happens on password input
$password.focus(passwordEvent).keyup(passwordEvent).keyup(confirmPasswordEvent).keyup(enableSubmitEvent);
//When event happens on confirmation input
$confirmPassword.focus(confirmPasswordEvent).keyup(confirmPasswordEvent).keyup(enableSubmitEvent);
$username.focus(usernameEvent).keyup(usernameEvent).keyup(enableSubmitEvent);
enableSubmitEvent();
<!DOCTYPE html>
<html>
<head>
<title>Sign Up Form</title>
<link rel="stylesheet" href="css/style.css" type="text/css" media="screen" title="no title" charset="utf-8">
</head>
<body>
<form action="#" method="post">
<p>
<label for="username">Username</label>
<input id="username" name="username" type="text">
<span>Please enter username</span>
</p>
<p>
<label for="password">Password</label>
<input id="password" name="password" type="password">
<span>Enter a password longer than 8 characters</span>
</p>
<p>
<label for="confirm_password">Confirm Password</label>
<input id="confirm_password" name="confirm_password" type="password">
<span>Please confirm your password</span>
</p>
<p>
<input type="submit" value="SUBMIT" id="submit">
</p>
</form>
<script src="//code.jquery.com/jquery-1.11.0.min.js" type="text/javascript" charset="utf-8"></script>
<script src="js/app.js" type="text/javascript" charset="utf-8"></script>
</body>
</html>
3 Answers
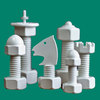
Steven Parker
231,248 PointsThere's a few issues here:
- you put a parenthesis so it separated the variable name (between the $ and the rest of $username)
- you put a semicolon after the if condition, which makes it a no-op (does nothing)
- if you fix both of the previous issues, the function still would not return anything if the condition was not true
- you are performing a numeric inequality test (>) on a string
Take a look at how the isPasswordValid() function is implemented just below on line 15. What you want to do is nearly the exact same thing, but instead of testing $password, you want to test $username, and you want to check for any characters instead of more than 8. If you model your function after that one, you should get it.
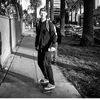
Joshua Paulson
37,085 PointsI'd recommend re-watching some of the videos in this track to get a better grasp on JS.
For the first task you need to remove several parenthesis so it looks like this
function isUsernamePresent() { return $username.val().length > 0; }

Ryu Blunder
Courses Plus Student 605 Pointsya, i got it, i post too quick before really figuring the problem.
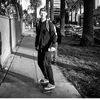
Joshua Paulson
37,085 PointsYou might still want to go back and refresh whenever you get the chance. When I started learning front-end, it was easy to skim along but it really pays off when you pay attention and learn to apply what's being taught in the lessons. If you ever have any questions or just want to brainstorm website ideas check out the forum. There's always help for those who seek it out.