Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial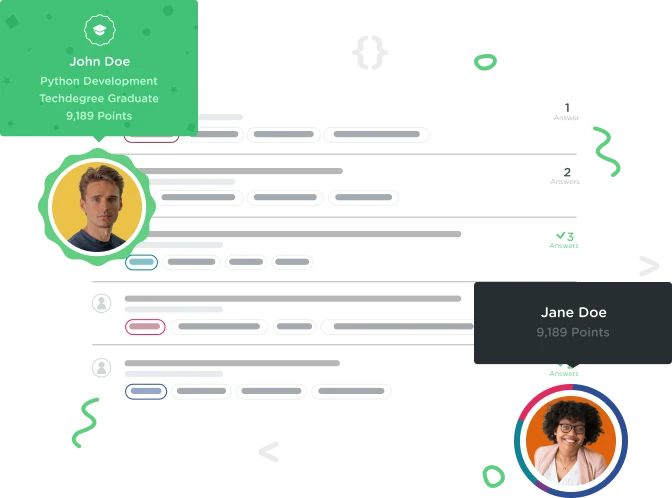

name564681351614
3,497 Points"Return" Function
I'm stuck again (happening a lot lately - contemplating giving up actually). Anyway - I really do not understand what I'm doing wrong with this code challenge. Please let me know.
Thank You, Eric
<!DOCTYPE html>
<html lang="en">
<head>
<title>JavaScript Foundations: Functions</title>
<style>
html {
background: #FAFAFA;
font-family: sans-serif;
}
</style>
</head>
<body>
<h1>JavaScript Foundations</h1>
<h2>Functions: Return Values</h2>
<script>
function arrayCounter (array) {
return array.length;
if (array === 'string', 'number', 'undefined') {
return = 0;
}
}
</script>
</body>
</html>
2 Answers
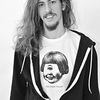
eck
43,038 PointsSo, you have a some mistakes with your conditional if statement and your use of "return" Below is code that will pass the challenge, that I have commented.
// you declared you function and parameter correctly, so we can skip that...
function arrayCounter(array){
/**
* Here are checking if array is a string, number, or undefined. To do this, we use something called
* "typeof" (See link below for more info) which returns a string value, which we are
* then comparing to see if it matches either number, string or undefined.
*
* Another piece this if statement is the "or" logical operator (See link below for more info). In an JS
* if statement, instead of using commas, we use "||" to represent mulitple conditions.
*/
if(typeof array === "string" || typeof array === "number" || typeof array === "undefined"){
/** if any of the above three conditions are true, then this code is run.
* return does a couple things:
*
* One, it will stop the function so that all code written after
* the return does not execute. This can be useful when using if statements to check functions from
* running unneccesary code.
*
* Two, it can send back a variable outside the scope of the function, which will be the number 0
* in this case.
*/
return 0;
} else {
// if none of the above conditions were true, then we assume array is in fact and Array, and return it's length
return array.length;
}
}
Don't give up, getting stuck is all part of the game! Every time you push through you will come out more skilled and knowledgeable. When I was first exposed to programming languages I was put off by my lack of understanding and wanted to quite. Having my mindset change over time, I came back to it, and over the course of ~ a year of learning I moved leaps and bounds beyond what I originally thought I would ever know.
Some tips I have for learning this stuff:
- Don't fear the challenge. Once you know you will be rewarded with personal growth on the other end, you can embrace it or possibly even crave it.
- Google is a super awesome resource, especially if you seek answers to programming problems. Learn how to ask it the right questions and with a little patients you can usually find an answer that will help you.
- Keep at it, and after a few months of consistent practice, see how far you have come :D
I hope this helps!! Good Luck!

Baruch Velez
275 PointsReturn isn't a variable so you can't give it a value. If you want it to return 0, the code should be
return 0;
if you want the function to return the length of the array, you have to place the array.length; after the if statement or it'll break the function.
name564681351614
3,497 Pointsname564681351614
3,497 PointsThank you Erik. That was very helpful. Learning programming is a weird process. I never studied it in college and am attempting to learn this part time in between full time work, kids, house, wife, etc. It's tough. I often feel that I NEED some sort of code bootcamp but I just can't quit my job. :)
I think that I have resigned to the fact that the best strategy for me is to really take small chunks (like one lesson and code challenge per day)...but do them everyday. No more trying to plow through numerous bits at a time. That just leads to me wanting to give up. Taking it this slow might mean that it takes me a year, maybe two, before I feel like I have a decent grasp. However, that's really not THAT long....and the other approach just makes me want to give up.
I'm 35 now so I often question if I have that kind of time to get on board. I think I do. Either way....I really think it's the only thing I can stick with given my situation.
What has your experience been with folks my age trying to get on board with this "stuff"?
Thanks Again! Eric