Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial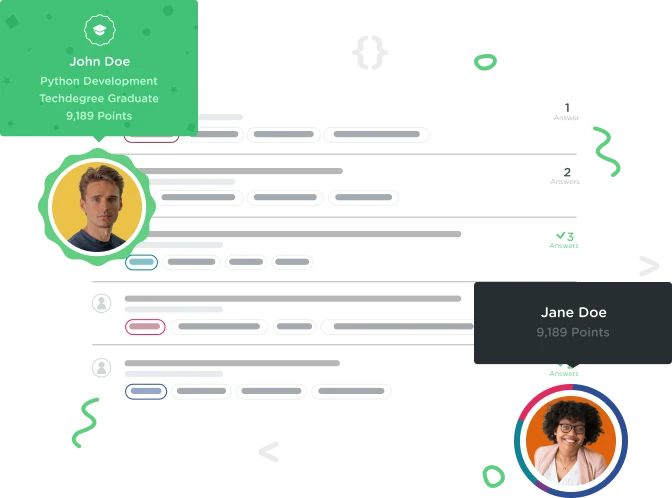

Ethan Anderson
Courses Plus Student 848 PointsReturn Images Instead of Strings
Hi, I've gone through the entire Crystal Ball project.
Currently, when I shake the device, 1 of 9 strings from the 'mAnswers' array is returned. Is there a way to tweak the code to return 1 of 9 .png files I have included in the appropriate drawable folder instead?
1 Answer
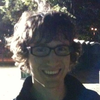
Ben Rubin
Courses Plus Student 14,658 PointsYes there is. I'll assume that your drawable files are named image1, image2, and so on. The first thing you'll need to do it store a reference to your application's context in your CrystalBall class. The reason you need this is that you have to know the application's context in order to access its resources. You could do this a few different ways, but I'll update the CrystalBall class to store the application's context.
public class CrystalBall
{
// This property will store the application's context
private Context context;
// We'll pass the context into CrystalBall's constructor, and store it within this object's context property
public CrystalBall(Context context)
{
this.context = context;
}
// Your other stuff
}
Make sure to update the line where you create a new CrystalBall object to pass in the main application's context, like this.
public class MainActivity extends Activity
{
// Other stuff
// Don't create the new CrystalBall object in the property's declaration anymore
private CrystalBall crystalBall; // Do it like this
// private CrystalBall crystalBall = new CrystalBall(); // Not like this anymore since the constructor is different
@Override
protected void onCreate(Bundle savedInstanceState)
{
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Create the CrystalBall object here, so that getApplicationContext() already has its context created. If not, getApplicationContext() will crash the application
this.crystalBall = new CrystalBall(getApplicationContext());
// Other stuff
}
// Other stuff
}
Once we have the context, we can get drawables like this.
public class CrystalBall
{
// This property will store the activity's context
private Context context;
// We'll pass the context into CrystalBall's constructor
public CrystalBall(Context context)
{
this.context = context;
}
public Drawable getDrawable()
{
Random randomGenerator = new Random();
int randomNumber = randomGenerator.nextInt(this.answers.length);
switch (randomNumber)
{
case 0:
return this.context.getResources().getDrawable(R.drawable.image01);
break;
case 1:
return this.context.getResources().getDrawable(R.drawable.image02);
break;
// .. and so on
}
}
// Your other stuff
}