Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial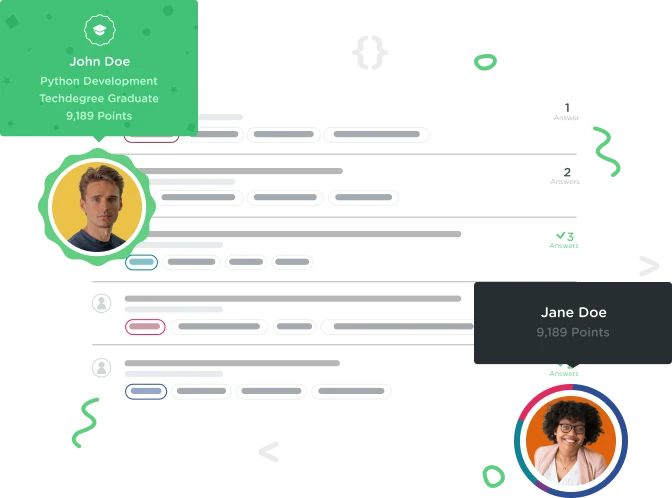

Uriel Fuentes
4,154 PointsReturn in callback of map function.
Why do we need to add a return when the callback returns a Pormise but we hadn´t before?
2 Answers
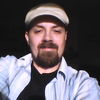
Robert Manolis
Treehouse Guest TeacherHi Uriel Fuentes, I can't say for sure with out seeing the exact code you're referring to, but I imagine it has something to do with the format of the code. In certain circumstances, the return
is implicit. For example, with simple one line arrow functions or what are sometimes called "concise body" arrow functions, the result of the expression is returned even without the use of the return
keyword.
param => expression
But whether implicit or explicit, the result of the promise needs to be returned. Hope that helps.

Keith Alorro
Full Stack JavaScript Techdegree Student 8,684 PointsHi Robert Manolis. I believe what Uriel Fuentes is referring to is an earlier exercise where we were instructed to declare the Promise instance as a variable without returning it. Such was the case with promises-breakfast.js:
const breakfastPromise = new Promise( (resolve, reject) => {
setTimeout(() => {
if (order) {
resolve('Your order is ready. Come and get it!');
} else {
reject( Error('Your order cannot be made.') );
}
}, 3000);
});
breakfastPromise
.then( val => console.log(val) )
.catch( err => console.log(err) )
Now we are being instructed to return the Promise instance during this lesson resulting in:
function getJSON(url) {
return new Promise((resolve, reject) => {
const xhr = new XMLHttpRequest();
xhr.open('GET', url);
xhr.onload = () => {
if(xhr.status === 200) {
let data = JSON.parse(xhr.responseText);
resolve(data);
} else {
reject( Error(xhr.statusText) );
}
};
xhr.onerror = () => reject( Error('A network error occurred') );
xhr.send();
});
}
btn.addEventListener('click', (event) => {
getJSON(astrosUrl)
.then(getProfiles)
.then( data => console.log(data) )
.catch( err => console.log(err) )
event.target.remove();
});
My understanding is the first example demonstrates the Promise being declared within the same block of code as the then() and catch() functions. This means the Promise did not need to be returned as it is within scope.
And if my understanding is still correct, the second example demonstrates an EventListener with the then() and catch() functions expecting a return value from a Promise created inside the invoked getJSON function. We cannot access this Promise outside of the getJSON function unless we return it.
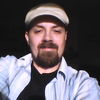
Robert Manolis
Treehouse Guest TeacherHi Keith Alorro, yeah, scope matters for reference, but I believe here it has more to do with how the .then() method works. That method is a built in part of the Promise API. And it expects to be called on a promise. So in your first example, .then() is being called directly on the promise that is created. No need to return anything because that the thing the .then method is being called on is actually a promise. But in the second example, that method is being called on a function that isn't a promise. So that function needs to return a promise for .then method to work.

Crystal Vesely
14,500 PointsSo if I am correct, the resolve() and reject() do not need return because it’s implicit?

Crystal Vesely
14,500 PointsThe more I think on it, the .then() and .catch() methods actually consume (pull out) the data inside resolve()/reject(). Therefore, no return is needed.