Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial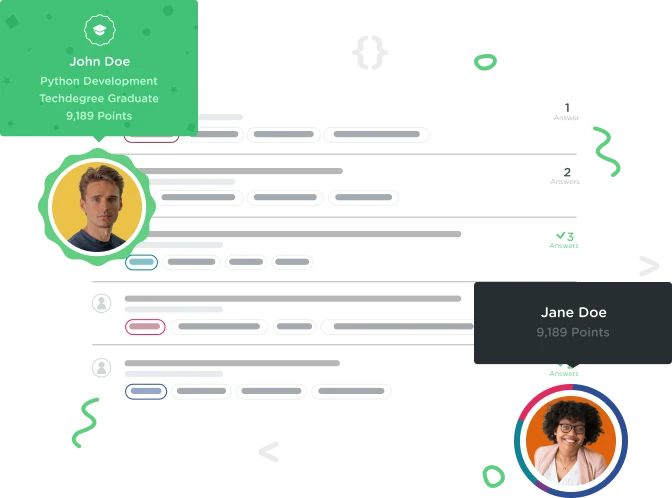
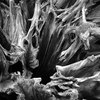
circlewave
8,232 Pointsreturn index vs return list[index] vs ...
In the code challenge for random_member task #3, I don't understand why we are required to return the function using square brackets.
My original solution to the challenge was as follows, and it works as expected when I run the code using the treehouse workspace:
import random
def random_member(list):
index = random.randint(0, len(list)-1)
return index
Yet the code challenge doesn't accept that as an answer, instead, what it wants is the following code (which I only learned by reading other answers here in the forums):
import random
def random_member(list):
index = random.randint(0, len(list)-1)
return list[index]
So my question is, why the square brackets? why list[index] and not just index?
or... why not just use the following code?
import random
def random_member(list):
return random.randint(0, len(list)-1)
^ which also works when I run it using workspaces. All of these other solutions work just fine in workspaces, yet the code challenge requires the square bracket solution.
This is weird because no-where in the course up until this point does it mention anything about doing returns using square brackets.
Can someone explain to me returning the function using the square brackets?
Very confusing and frustrating!
Thanks so much for your time, really appreciate it!
3 Answers
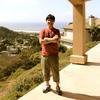
Richard Lu
20,185 PointsHey circlewave,
The question, from what I understand, is that you're trying to figure out why it isn't accepting the integer generated from the random number function as a return value. The challenge states that you must return the list item with that index, not just the index. For example:
import random
a_list = ["a", "b", "c", "d"]
def random_member(list):
index = random.randint(0, len(list)-1)
return list[index]
random_member(a_list)
# if the randomly generated number was 2
# the function returns list[index] which equals "c", the value extracted from (a_list[2])
If you're still having trouble with this challenge, I would suggest to review this video: https://teamtreehouse.com/library/python-basics/shopping-list/lists
Hope I've helped. Happy Coding! :)
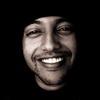
miikis
44,957 PointsMy original solution to the challenge was as follows, and it works as expected when I run the code using the treehouse workspace
Actually, the code you posted doesn't work as expected; it only returns a random number. The purpose of the random_member() function (according to the Code Challenge) was to be able to pick a random member of a list and return that. Here's what you posted:
import random
def random_member(list):
# index is a random number between 0 and the length of the list minus 1
index = random.randint(0, len(list)-1)
# you just returned that number, not what the question asked for
return index
Here's the correct answer:
import random
def random_member(my_list):
# 'randy' here generates a random member between '0' and 'the length of the list minus one'
randy = random.randint(0, len(my_list)-1)
# here, remember that 'my_list' is a list/array
# this means that you can select 'members' out of it by doing something like 'my_list[0]'
# but, instead of 'my_list[0]' we do 'my_list[randy]', where randy is a random number
return my_list[randy]
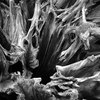
circlewave
8,232 PointsHi guys,
Thanks so much for the help. It makes total sense now. Can't believe I missed something so obvious.
Thanks again!! :)
*edit: wish i could select both as best answer, as they both helped me, but will select first one on a technicality ;)