Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial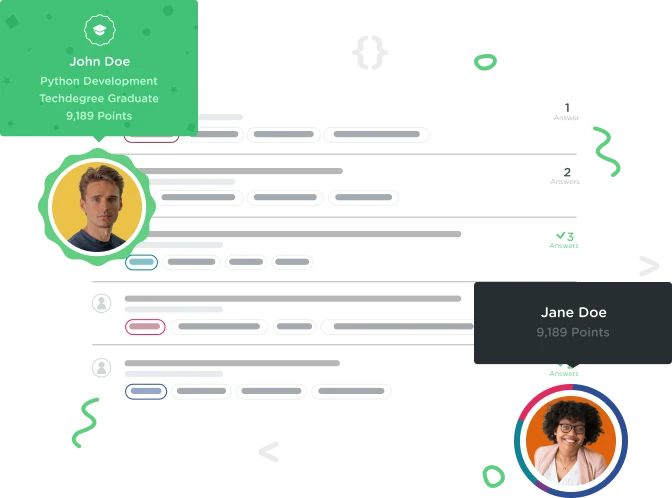
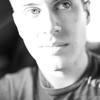
Eric Ewers
13,976 PointsReturn key=>value pair from $_POST variable
I would like to return the selected key=>value pairs after POST and put them into two separate variables.
I am using PHPMailer to send out a receipt to the customer and it needs to say what they ordered and how many of each product.
<!-- Products -->
<?php
// Standard Prices
$products = array(
'Director\'s Chair' => 60,
'4\' x 6\'\' H Table' => 40,
'6\' x 30\'\' H Table' => 45,
'8\' x 30\'\' H Table' => 50,
'2 - 6\'\' x 4\' Wide Step Risers' => 25,
'4\' x 30\'\' H Draped Table' => 80,
'6\' x 30\'\' H Draped Table' => 90,
'8\' x 30\'\' H Draped Table' => 100,
'Extensions to make above 42\'\' H' => 20,
'2 - 6\'\' x 4\' Wide Step Draped Risers' => 45,
'High Top Table with Linen' => 100,
'30\'\' Round Table w/ cover 30\'\' H' => 75,
'Waste Basket w/ Liner' => 20,
'Tripod Easel' => 25,
'Literature Racks' => 60
);
foreach($products as $product=>$price) {
?>
<div class="form-group">
<label class="col-sm-4 control-label" for="textinput"><?php echo $product ?></label>
<div class="col-sm-6">
<select name="product[]" class="product form-control">
<option value=0>0</option>
<option value=<?php echo $price * 1 ?>>1 ($<?php echo $price * 1 ?>)</option>
<option value=<?php echo $price * 2 ?>>2 ($<?php echo $price * 2 ?>)</option>
<option value=<?php echo $price * 3 ?>>3 ($<?php echo $price * 3 ?>)</option>
<option value=<?php echo $price * 4 ?>>4 ($<?php echo $price * 4 ?>)</option>
<option value=<?php echo $price * 5 ?>>5 ($<?php echo $price * 5 ?>)</option>
</select>
</div>
</div>
<?php } ?>
2 Answers
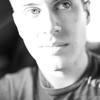
Eric Ewers
13,976 Points-SOLUTION IS BELOW THIS POST-
Hugo, I'm hoping you are still reading this. I have found a partial solution, but could use some more help on a smaller issue to get it working just the way I want. I appreciate all the help you've given so far!
I need to get each individual value from each select box and put those values into an array (it has to be an array). The way it is right now, the foreach loop outputs all of the values, but they are bunched together without commas. How can I get these values into an array?
<?php
$products = array(
"Director's Chair" => 60,
"4' x 6\" H Table" => 40,
"6' x 30\" H Table" => 45,
"8\' x 30\" H Table" => 50,
"2 - 6\" x 4' Wide Step Risers" => 25,
"4' x 30\" H Draped Table" => 80,
"6' x 30\" H Draped Table" => 90,
"8' x 30\" H Draped Table" => 100,
"Extensions to make above 42\" H" => 20,
"2 - 6\" x 4' Wide Step Draped Risers" => 45,
"High Top Table with Linen" => 100,
"30\" Round Table w/ cover 30\" H" => 75,
"Waste Basket w/ Liner" => 20,
"Tripod Easel" => 25,
"Literature Racks" => 60
);
foreach((array)$_POST['product'] as $sum) {
}
$price = array(VALUES FROM EACH SELECT BOX SHOULD GO HERE);
?>
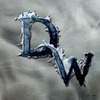
Hugo Paz
15,622 PointsHi Eric,
<!-- Products -->
<?php
// Standard Prices
$products = array(
'Director\'s Chair' => 60,
'4\' x 6\'\' H Table' => 40,
'6\' x 30\'\' H Table' => 45,
'8\' x 30\'\' H Table' => 50,
'2 - 6\'\' x 4\' Wide Step Risers' => 25,
'4\' x 30\'\' H Draped Table' => 80,
'6\' x 30\'\' H Draped Table' => 90,
'8\' x 30\'\' H Draped Table' => 100,
'Extensions to make above 42\'\' H' => 20,
'2 - 6\'\' x 4\' Wide Step Draped Risers' => 45,
'High Top Table with Linen' => 100,
'30\'\' Round Table w/ cover 30\'\' H' => 75,
'Waste Basket w/ Liner' => 20,
'Tripod Easel' => 25,
'Literature Racks' => 60
);
?>
<!-- begin form -->
<div class="form-group">
<form method='post' action='receipt.php'>
<?php
/* populate form */
foreach($products as $product=>$price) {
?>
<label class="col-sm-4 control-label" for="textinput"><?php echo $product ?></label>
<div class="col-sm-6">
<select name="<?php echo $product?>" class="product form-control">
<option value=0>0</option>
<option value=<?php echo $price * 1 ?>>1 ($<?php echo $price * 1 ?>)</option>
<option value=<?php echo $price * 2 ?>>2 ($<?php echo $price * 2 ?>)</option>
<option value=<?php echo $price * 3 ?>>3 ($<?php echo $price * 3 ?>)</option>
<option value=<?php echo $price * 4 ?>>4 ($<?php echo $price * 4 ?>)</option>
<option value=<?php echo $price * 5 ?>>5 ($<?php echo $price * 5 ?>)</option>
</select>
</div>
</div>
<?php } ?>
<input type='submit' value='submit'>
</form>
I added the form functionality, all products are under the same form.
I created a receipt.php file that just dumps the $_POST variable
<?php
var_dump($_POST);
?>
As you can see, you now get a key pair value with the name of the product and its price. The way you set it up, it doesnt tell you how many of a product were ordered, only the total price.
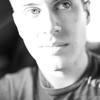
Eric Ewers
13,976 PointsIs there a way to format this output so that it's more readable? This is what I got:
<?php
array(15) { [0]=> string(2) "60" [1]=> string(1) "0" [2]=> string(1) "0" [3]=> string(1) "0" [4]=> string(1) "0" [5]=> string(1) "0" [6]=> string(1) "0" [7]=> string(1) "0" [8]=> string(1) "0" [9]=> string(1) "0" [10]=> string(1) "0" [11]=> string(1) "0" [12]=> string(1) "0" [13]=> string(1) "0" [14]=> string(1) "0" }
?>
Also, I'm just going to use the output in a variable, since I already have a form.
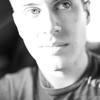
Eric Ewers
13,976 PointsI answered my own question, but I was really hoping to not have the actual "keys" but the string like "Director's Chair"
Code:
<?php
echo '<pre>';
$order = print_r($_POST['product']);
echo '</pre>';
?>
Output:
<?php
Array
(
[0] => 60
[1] => 200
[2] => 0
[3] => 0
[4] => 0
[5] => 0
[6] => 0
[7] => 0
[8] => 0
[9] => 0
[10] => 0
[11] => 0
[12] => 0
[13] => 0
[14] => 60
)
?>
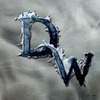
Hugo Paz
15,622 PointsCan you post your code please.
The way i did above I'm getting the product name as the key.
array(15) { ["Director's_Chair"]=> string(1) "0" ["4'_x_6''_H_Table"]=> string(1) "0" ["6'_x_30''_H_Table"]=> string(2) "90" ["8'_x_30''_H_Table"]=> string(1) "0" ["2_-_6''_x_4'_Wide_Step_Risers"]=> string(1) "0" ["4'_x_30''_H_Draped_Table"]=> string(1) "0" ["6'_x_30''_H_Draped_Table"]=> string(1) "0" ["8'_x_30''_H_Draped_Table"]=> string(1) "0" ["Extensions_to_make_above_42''_H"]=> string(1) "0" ["2_-_6''_x_4'_Wide_Step_Draped_Risers"]=> string(1) "0" ["High_Top_Table_with_Linen"]=> string(1) "0" ["30''_Round_Table_w/_cover_30''_H"]=> string(1) "0" ["Waste_Basket_w/_Liner"]=> string(1) "0" ["Tripod_Easel"]=> string(1) "0" ["Literature_Racks"]=> string(1) "0" }
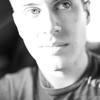
Eric Ewers
13,976 PointsYou're very sneaky lol!
You changed this line:
<select name="<?php echo $product?>" class="product form-control">
The reason I had it as "product[]" was because I needed to also get the sum of the selected values in order to charge the person.
<?php
// Returns the sum of all selected and converts price
$amount = 0;
foreach((array)$_POST['product'] as $sum) {
$amount += $sum;
$stripe_amount = $amount * 100;
}
?>
Herein lies the issue:
a) Get the product name, but no price.
<?php
<select name="<?php echo $product?>" class="product form-control">
?>
b) Get the price, but no product name.
<?php
<select name="product[]" class="product form-control">
?>
Any ideas?
Hugo Paz
15,622 PointsHugo Paz
15,622 PointsHey Eric,
Check if this works. I rewrote some things, please test both files to see the result.
product.php
receipt.php
In the form I am passing both the quantity and the total price for that product. I separate both values with a pipe (|).
I then use the array method explode to get both values.
Eric Ewers
13,976 PointsEric Ewers
13,976 PointsThis works perfectly!
The only thing is that it gets ALL form data, when it should only get this particular select box. I have other select boxes on the page that I can't have interfering.
Eric Ewers
13,976 PointsEric Ewers
13,976 PointsCancel that!
-SOLVED-
Only 1 last question, I promise:
How can I store the data from echo statement into a variable? I need to use the output of all the products/prices in my email body.
Hugo Paz
15,622 PointsHugo Paz
15,622 PointsHi Eric,
Pretty simple, create a variable which will store the email body and concatenate each iteration of the foreach loop into it.
The echo in the end is just to show that it is storing the correct information.