Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial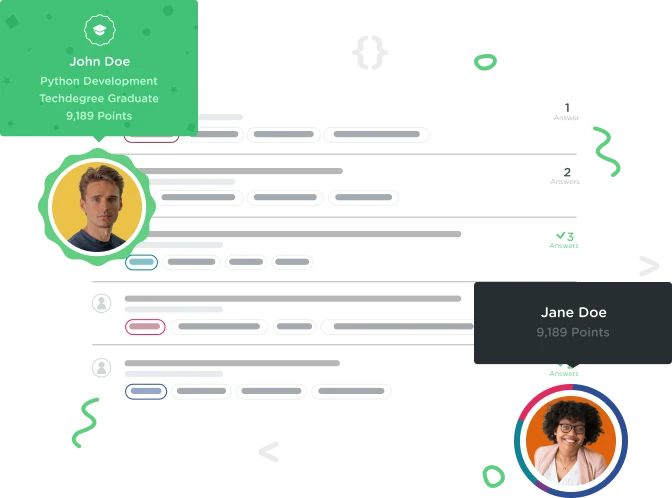
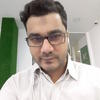
Owais Ul Haq
2,020 Points"Return" keyword in javascript
Please let me know why we use return keyword and what does the keyword do. Instead we can call the function by the name for example
function myprogram(){ alert('Enter any value'); } myprogram();
3 Answers

Alexander La Bianca
15,959 PointsYou use the return keyword to get a value out of the function. You want to do this if you want to use the value you 'returned' later on in your program.
function add(value1, value2) {
var sum = value1 + value2;
return sum //this will return the value out of the function so we can use it outside of the function
}
var x = 1;
var y = 2;
var theSum = add(x,y);
//theSum is now 3
If you did not return the variable sum like I did above. You could not have used it as sum only exists in the scope of add() Think of your function as a tunnel. You are providing parameters into the function (in this case value1 and value2). Then you are returning a value out of the function (in this case sum). It is what comes out of the end of the tunnel in such a case.

KAREN ROSS
3,026 Points6....?

Enemuo Felix
1,895 PointsIf i understand you correctly Alex, we use the return keyword so that we can use the function value outside the function scope and also because a particular function can only be executed once in a script so a return keyword is needed to hold the value of the function once it's executed and terminates?

Alexander La Bianca
15,959 PointsNot exactly. You can run a function as many times as you want. The "return" keyword is used to return a particular value that only lives in the function scope. For example the 'sum' value you the add(value1,value2) function ONLY lives in that function's scope. So we can't access it outside of the function. UNLESS we return it.
var x = 1;
var y = 2;
var theSum = add(x,y); //will return sum from inside add() and assign it to 'theSum'
var anotherSum = add(x,theSum) //will return the sum inside add() and assign it to 'anotherSum'
var theLastSum = add(y,anotherSum)
Go through this example and tell me what the value of theLastSum is. If you find out, you will understand the concept of "return" :)
Tanner Williams
1,315 PointsTanner Williams
1,315 PointsAre the parameters separate from variables? Do the names value1, value2 have any significance?
Alexander La Bianca
15,959 PointsAlexander La Bianca
15,959 PointsNo the value1 and value2 only have significance when you access them inside your function. You can name them whatever you want, as long they make sense (don't name them some random letter sequence). In the above example x - is value1 and y is value2. You can think of value1 and value2 as the function parameters and x and y the function arguments.