Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial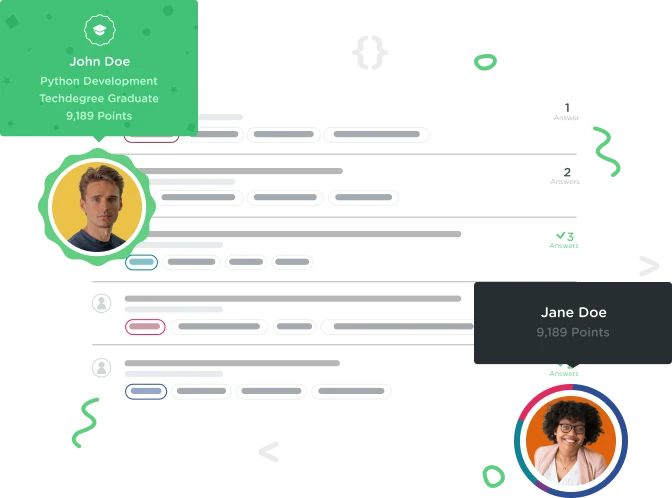

Mathew Yangang
4,433 Pointsreturn list of tuple
I'm trying to return a list of tuple , but can't figure out how
# combo([1, 2, 3], 'abc')
# Output:
# [(1, 'a'), (2, 'b'), (3, 'c')]
def combo([1,2,3],'boy'):
a = [1,2,3]
b = 'boy'
for num_string in a,b:
return a,b.append(list(tuple))
2 Answers
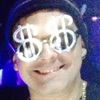
Mustafa Başaran
28,046 PointsHi Matthew,
You do not need to assign values to variables within the function. You only need to set them as parameters to your function such as a, b. In other words, they are placeholders and will be assigned values when you make use of the function after you define it.
def combo(a, b):
# create an empty list so that you can return it as output at the end of the function.
output = []
# iterate over the iterable with a range(len()) construction. a and b have the same length by assumption
# this loop will give you all the index values starting from 0 to len(a) - 1
for i in range(len(a)):
# get the items from a and b with index position i and assign them to a tuple, tup
tup = (a[i], b[i])
# append tup to the list, output
output.append(tup)
# the loop is finished. return the list, output
return output
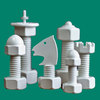
Steven Parker
229,732 PointsIt looks like you have a few other issues going on there as well. Here's some hints:
- the function parameters should just be names, they may represent lists or strings
- you will used the passed-in data by referencing those names, no literal data items
- you can initialize an empty list before the loop
- in the loop, you can add to your list but not return it yet
- creating a tuple can be done by just surrounding two items separated by a comma in parentheses
- return the new list after the loop finishes

Mathew Yangang
4,433 PointsThanks Steven, but i don't understand step 2 on passed in data by referencing the names. Can you please elaborate a little bit on that?
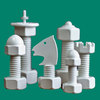
Steven Parker
229,732 PointsThe first 2 go together, so for example:
def combo(a, b): # so if the parameters are named "a" and "b"
# a = [1,2,3] then you would not assign fixed values to them
# b = 'boy'