Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial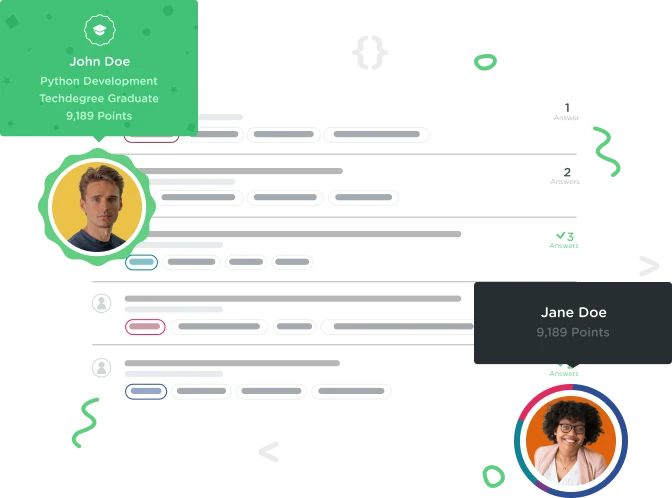

Tom Lawrence
8,685 PointsReturn multiple things from a function
Hi, in this video (06:30) it says you cant return multiple things from a function but in the next video, we return multiple things from a function using the following:
function getRandomNumber(upper) {
var randomNumber = Math.floor( Math.random() * upper ) + 1;
return randomNumber;
}
function getArea(width , length, unit){
var area = width * length;
return area + " " + unit;
}
console.log(getArea(10,20, 'sq ft'));
Can someone clear up what it is you cant do with return as I must be missing something and watched it a couple of times now and a bit lost.
It was mentioned that "you can only return one thing, a string, a number a bool" so then I thought ok well maybe it can only return 1 type but that cant be right either as in the above return statement we have an int and a string! (as in the string " ", not the string held within the unit variable).
The only thing I can think is that
return area + " " + unit;
actually isnt "returning multiple things" it is returning 1 thing which is concatenated, so multiple things joined together making "one thing" is that it?
Thanks
4 Answers
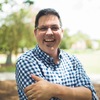
Dave McFarland
Treehouse TeacherSorry for the confusion Tom. Dino Paškvan is correct. With this line of code
return area + " " + unit;
The stuff after return
is evaluated before it is returned. So the area is added to a space which is added to the unit ('sq ft', for example) to create a SINGLE string, which is then returned. It's the same as
return '20 sq ft.';
JavaScript converts numeric values into strings when using the +
operator with strings, so the number in the area
variable is converted to its string version.
And yes, an array can hold multiple items in them -- that's why they're so useful. But when using the return
statement with an array you're still just returning a single thing -- the array.

Dino Paškvan
Courses Plus Student 44,108 PointsThat's exactly it. return area + " " + unit;
actually returns a single string.
Even if you were to return an array (containing multiple elements), or an object (containing multiple properties) that would still count as a single array/object.

Tom Lawrence
8,685 Pointsthanks for your help thats all clear now and no problem Dave, the course was really good!
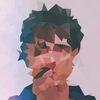
cesare
15,180 PointsCreate and return an array within two (or 3, 4, 5...) variables. :)
function returnArray(a, b){
var c = a + b;
var array = new Array(a, b, c);
return array;
}
var myArray = returnArray(3, 5);
console.log(myArray[0] + " + " + myArray[1] + " = " + myArray[2]);
Fuad Ak
Courses Plus Student 691 PointsFuad Ak
Courses Plus Student 691 PointsThank you Dave for amazing js basic course, I loved it.!