Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial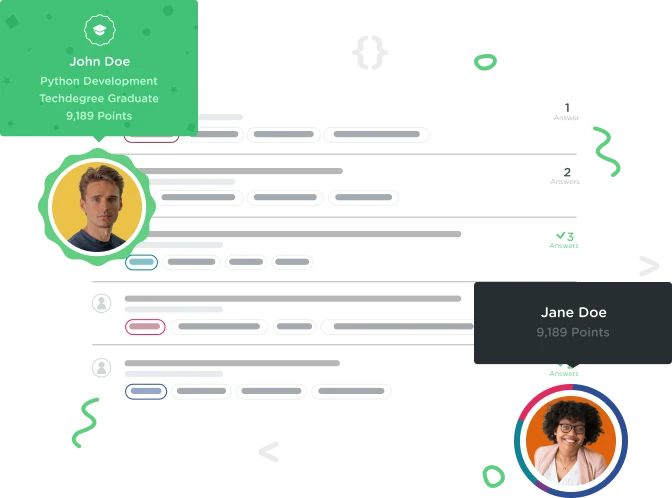
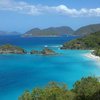
Gina Russo
8,742 Pointsreturn num;
I just want to make sure I'm understanding this correctly. The "num" variable is being saved in the "randomNumber" variable isn't it? I think I'm answering my own question here, but just want to be sure. Thank you!
var randomNumber = getRandomNumber(10);
var guess;
var guessCount = 0;
var correctGuess = false;
function getRandomNumber( upper ) {
var num = Math.floor(Math.random() * upper) + 1;
return num;
}
6 Answers

David Tunon
4,312 PointsThank you Marcus. I understand a lot more now than I did 20 minutes ago. Give this man a raise!!

Damien Watson
27,419 PointsHi Gina,
The 'num' variable is being defined inside the 'getRandomNumber' function as a local variable and isn't available outside of the function. In this instance, it is being used only to help explain the return value.
var randomNumber = getRandomNumber(10);
var guess;
var guessCount = 0;
var correctGuess = false;
function getRandomNumber( upper ) {
var num = Math.floor(Math.random() * upper) + 1;
return num;
}
If you didn't want to do anything else but return the random number, you could chop the function down to be:
function getRandomNumber( upper ) {
return Math.floor(Math.random() * upper) + 1;
}
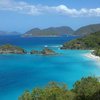
Gina Russo
8,742 PointsThanks Damien, Here's all of the code...I'm just trying to understand how the returned "num" value gets assigned to the "randomNumber" variable. (and why can't I get the code to show like yours is showing? I added the three ticks and "javascript"...sorry about that!
var randomNumber = getRandomNumber(10);
var guess;
var guessCount = 0;
var correctGuess = false;
function getRandomNumber( upper ) {
var num = Math.floor(Math.random() * upper) + 1;
console.log(num);
return num;
}
do {
guess = prompt('I am thinking of a number between 1 and 10. What is it?');
guessCount++;
if (parseInt(guess) === randomNumber) {
correctGuess = true;
}
} while (!correctGuess)
document.write('You guessed the number ' + randomNumber + ' in ' + guessCount + ' attempts.');
console.log(randomNumber);
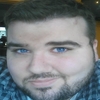
Marcus Parsons
15,719 PointsHey Gina,
Use this photo as a guideline for how to format your code. The 3 backticks should be on their own separate lines. Also, ensure there are blank spaces above the first 3 backticks and below the last 3 backticks.

Damien Watson
27,419 Points'getRandomNumber' is a function which can receive a variable, process it and return a result. In the example below, 'randomNumber' is set to the result 'return'ing from 'getRandomNumber'. The function returns a value, the 'num' is just how that value is tracked inside the function.
You can call a function what ever you like, which helps if it describes what the function does. Breaking it down, this is what is happening:
// Pass '10' into 'getRandomNumber', this returns a value which is stored in 'randomNumber'
var randomNumber = getRandomNumber(10);
function getRandomNumber( upper ) {
var num = Math.floor(Math.random() * upper) + 1;
return num;
}
// If you were to remove the 'function' stuff out of the above, it would look like:
var randomNumber = Math.floor(Math.random() * 10) + 1;
Does this help explain it?
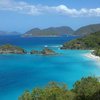
Gina Russo
8,742 PointsThanks Marcus!!
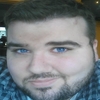
Marcus Parsons
15,719 PointsNo problem! :)
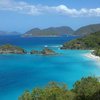
Gina Russo
8,742 PointsAbsolutely!! Thank you so much Damien :)

Damien Watson
27,419 PointsWelcome :)

David Tunon
4,312 PointsFollow up questions: Why do we need to first assign the function to a variable and then return a variable in order to return a value?
function getRandomNumber( upper ) {
var num = Math.floor(Math.random() * upper) + 1;
return num;
Is there a way to do both in one step? Such as...
function getRandomNumber( upper ) {
return num = Math.floor(Math.random() * upper) + 1;
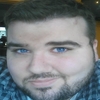
Marcus Parsons
15,719 PointsHey David,
You definitely don't have to set num at all here. You could just return everything in the return statement:
function getRandomNumber (upper) {
return Math.floor(Math.random() * upper) + 1;
}

David Tunon
4,312 PointsThank you Marcus. Is the advantage that you can use the return value as a variable more easily? If not, can you help me to understand why we would return a value as a variable?
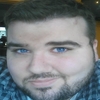
Marcus Parsons
15,719 PointsThere's no reason to set a variable here because num
is destroyed as soon as the function completes its execution. You couldn't use num
outside of this function (as is) since it is created as a local variable and remember that JavaScript operates on a function scope where function variables are local variables and all other variables are global.
If, however, you left the "var" keyword off of num
, it will be hoisted into the global scope and available to other pieces of code, including functions. However, this is not advised unless you specifically need that variable to be available in other functions; in this case, you do not.
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsNo problem, David! Happy Coding!