Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial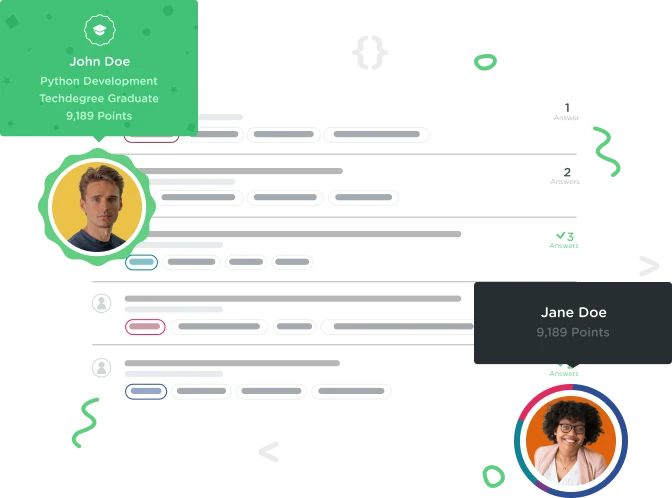

Quanton poulo
6,361 Points"return" statement and value in a method
Hello,
I am a newbie in programming in general and recently I started taking coursed of Ruby on Rail. I still can't understand why and when I need to use "return" in a method and I would like to have some help with this. Please, explain it in dummy text and if it's not too much to ask, I will be glad If you can explain it with a dummy example also :) so I can see it and test it out for myself.
4 Answers
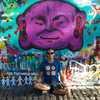
Andrew Stelmach
12,583 PointsA method is equal to its last evaluated statement. For example,
def some_method
name = "Andrew"
end
some_method
=> "Andrew"
'return' is used when you want to return a value 'now' and exit the method. So, here it would make no difference:
def some_method
return name = "Andrew"
end
some_method
=> "Andrew"
'return' is used a lot, because often you want to exit a method early, eg:
def some_method(name)
if name == "Andrew"
return "You are Andrew"
end
puts "You are not Andrew"
end
some_method("Andrew")
=> "You are Andrew"
# Method without 'return':
def some_method(name)
if name == "Andrew"
puts "You are Andrew"
end
puts "You are not Andrew"
end
some_method("Andrew")
You are Andrew
You are not Andrew
=> nil
When you start writing real methods of your own you'll find out how important and frequently used 'return' is. If you need anymore explanation, give me a shout. I recommend you play around in irb to (did you know you can write methods in it and execute them? Try it in workspaces.

Quanton poulo
6,361 PointsHi Andrew,
Thanks for your reply and explanation! It was very clear to me. I made this small code and and I wonder if I used "return" the right way. Would you please check it for me ?
I also have a question about a calling a method. In this code below, when I call the first method (name) and run the code, It will ask me twice " To enter the name", and when I don't call it, It only ask once! But I learned that every method should be called, isn't that right?
Thanks for your time and patients!
'''
def name
print 'Enter your name?'
name = gets.chomp
end
def adress
print "What is your adress #{name}?:"
adress = gets.chomp
if adress == "Leiden"
puts"#{adress} is a very nice city"
elsif
puts "I don't know were that is but that's no problem.\n"
end
end
def cars
print "I heard you like cars! Is that true? (yes or no): "
answer = gets.chomp
if answer == "yes"
print "how many cars do you own? "
cars_amount = gets.chomp.to_i
elsif answer == "no"
return puts"Too bad"
end
if cars_amount >= 3
puts" Wow, that a huge collection"
else cars_amount <= 2
puts"Hhhmm you should buy more cars"
end
end
name adress cars '''

Quanton poulo
6,361 PointsSorry for the weird post. It's my first time posting a code and don't know yet How I am suppose to do it :(

Quanton poulo
6,361 PointsHey Andrew, I found a way to sent the code as one whole. Check this link out
It's a online code interpreter!
hope to hear what you think about the code!