Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial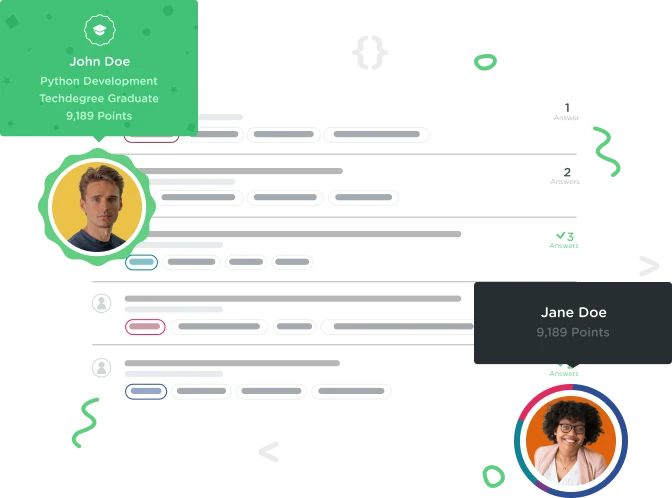

Mathew Yangang
4,433 Pointsreturn teacher with the most courses
I am trying to return the teacher with the most courses in task 4, but don't what i am doing wrong
# The dictionary will look something like:
# {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Each key will be a Teacher and the value will be a list of courses.
#
# Your code goes below here.
def num_teachers(teacher):
number = len(teacher)
return number
def num_courses(teacher):
courses = 0
for course in teacher.values():
courses += len(course)
return courses
def courses(teachers):
course = []
for teacher in teachers.values():
course.extend(teacher)
return course
def most_courses(teacher):
max_count = len(teachers.values())
if teacher > max_count:
name = max_count.update(teacher)
return name
1 Answer
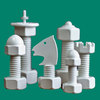
Steven Parker
231,269 PointsFYI, here are the main Python errors, but you won't want to just fix them:
ā if teacher > max_count:
TypeError: '>' not supported between instances of 'dict' and 'int'
ā ā name = max_count.update(teacher)
AttributeError: 'int' object has no attribute 'update'
You'll probably want to revise your basic approach. You might use a loop to compare the size of each courses list in the dictionary to a saved maximum. Then, when the size is greater, save the new maximum and also the teacher's name that goes with it. Then when the loop finishes you can return the name of the last saved teacher.