Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial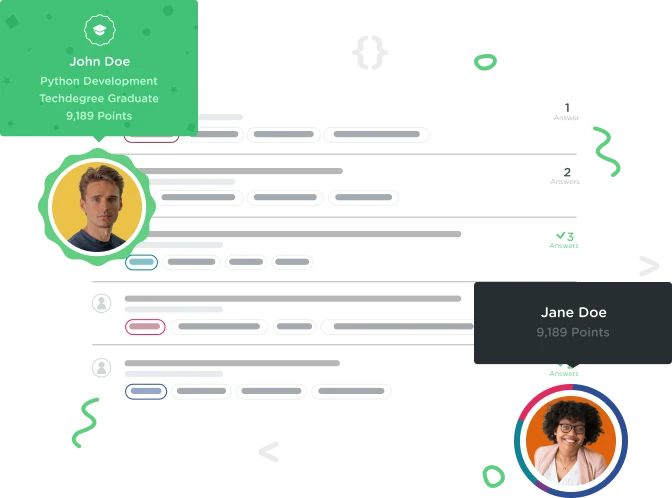

Jamie Baldaro
15,616 PointsReturn the average length of the tongues of the frogs in the array. Use a foreach loop as part of your solution.
I'm ashamed to say i'm completely lost. I've looked at this for ages. re-watched the video time and time again and i still can't fathom it u.u
Would anyone be willing to explain to me how this works exactly?
10 Answers
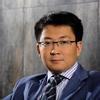
Andrei Li
34,475 PointsIs it ok if I share a correct code? (question to Treehouse Forum admins) I am so happy to solve that I can't hold myself from sharing. Because there are cases where I couldn't find a correct answer in Treehouse forum and it makes me struggle.
namespace Treehouse.CodeChallenges
{
class FrogStats
{
public static double GetAverageTongueLength(Frog[] frogs)
{
double total = 0;
foreach(Frog frog in frogs)
{
total += frog.TongueLength;
}
return total/frogs.Length;
}
}
}

Kyle Waite
4,637 Pointsnamespace Treehouse.CodeChallenges { class FrogStats { public static double GetAverageTongueLength(Frog[] frogs) { double total = 0;
foreach(Frog frog in frogs)
{
total+=frog.TongueLength;
}
return total/frogs.Length;
}
}
}

Mula Goa
Courses Plus Student 1,387 PointsThe answer is
namespace Treehouse.CodeChallenges
{
class FrogStats
{
public static double GetAverageTongueLength(Frog[] frogs)
{
int total = 0;
for(int i = 0; i < frogs.Length; i++)
{
total += frogs[i].TongueLength;
}
return total/frogs.Length;
}
}
}
For those wondering where TongueLength came from the Frog.cs file. There is two file to this exercise, so by using .TongueLength you can using the get property of Tongue Length. For reference the Frog.cs file is
namespace Treehouse.CodeChallenges
{
public class Frog
{
public int TongueLength { get; }
public Frog(int tongueLength)
{
TongueLength = tongueLength;
}
}
}

anil rahman
7,786 Points public static double GetAverageTongueLength(Frog[] frogs)
{
Frog frog = new Frog(7);
int sum = 0;
int count = 0;
foreach(Frog f in frogs)
{
sum += f.TongueLength;
count++;
}
int avg = sum / count;
return avg;
}

anil rahman
7,786 PointsI've kind of just guessed that and it works so im guessing theres a better way to write it. :)
Kathryn Ann
10,071 PointsWhat's the reason for creating a new Frog at the beginning of the method? Other than that, the only change I'd suggest is getting rid of the avg variable for efficiency, since you only use it once. Instead, you could just return sum / count.

Tony Lawrence
3,056 PointsThanks for the example Anil. Though the Frog.cs is still to be considered. I'm still wondering how you got your 'f' value when you made your for each loop, since it's not called in your example class or the Frog.cs.
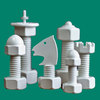
Steven Parker
231,261 PointsYou know how to do an average, right? You add up all the values and then divide by the number of things.
In this case, the "things" are the frog tongues and the values are the tongue lengths.
Your function is going to get a list of Frog objects. In this list, every Frog has a TongueLength. So if you create a loop that takes each one and adds it to a total, and then after the loop divides it by the number of Frogs, you'd have an average length.
Do you have a better idea about what to do now?

Jamie Baldaro
15,616 PointsI want to say yes but no... Not a clue >.< I feel like Mondays are not good days to learn u.u
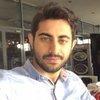
HIDAYATULLAH ARGHANDABI
21,058 PointsTry this answer it will work
double total=0;
for(int i=0;i<frogs.Length;i++)
{
total+=frogs[i].TongueLength;
}
return total/frogs.Length;
Enjoy Coding

Emma Wyman
13,250 PointsThis answer would not work because it is supposed to be a foreach
loop.

Rakhil Chandiram
3,483 Pointsthank you Anil you made it so much easier to understand. :)

Maddalena Menolascina
6,668 PointsI did not quite understand a few basic things... please keep in mind that I will start this whole course again as well as going through the basis again, but I get very confused when I see using variables that we did not initialize in a way that I understand: For example, I get the meaning of the foreach loop, but in anil rahman's code where did the "f" come from? I will study more but a quick answer would be much appeciated Sorry for the silly doubt
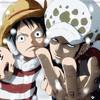
jassem aldhaheri
Python Development Techdegree Student 11,123 Pointsthanks for explaining in a nice way.

Philip Lloyd
2,773 PointsI believe you can skip out the count variable altogether here and just use "frogs.Length" for the size of the array.
With regards to the f question, I'm still learning and know more in the VBA world but I believe it's just a placeholder name so you can reference the Frog within the loop and won't be used outside the loop. So could easily have also said,
foreach(Frog kermit in frogs)
or could have said,
foreach(Frog meh in frogs)
as long as you then said kermit.TongueLength or meh.TongueLength respectively.
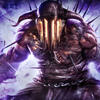
Ricardo Ferreira
9,694 PointsMy question is: where does this .TongueLength come from in the body of the loop. The function's name is GetAverageTongueLength.
alex paige
17,474 Pointsalex paige
17,474 Pointswhere does the singular 'frog' come from?