Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial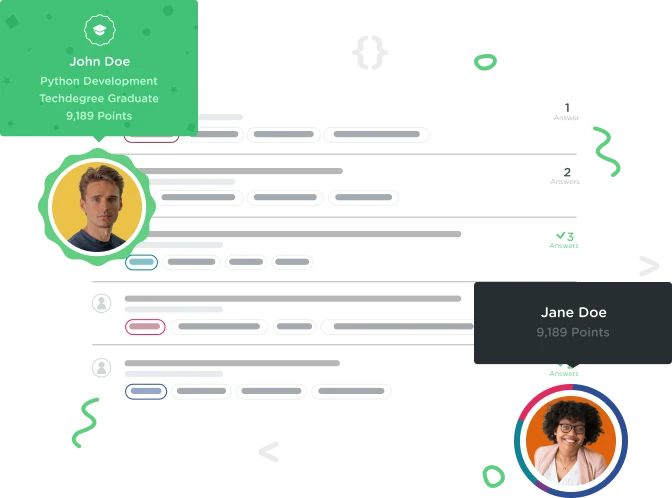

Bob Goldsmith
5,444 PointsReturn Values Challenge - How does the function affect an Array?
I finally got the correct answer, but it left me even more confused. I don't understand how the first two return statements would apply to an array. I created an array to try and figure it out.
function arrayCounter(array) {
if (typeof array === "string") {
return 0;
}
if (typeof array === "number") {
return 0;
}
if (typeof array === "undefined") {
return 0;
} else {
return array.length;
}
};
var my_array = [ ];
console.log(arrayCounter(my_array));
I understand that if I give my_array a bunch of numbers and/or strings, it will return the number of however many elements there are. I'm assuming that if I leave it empty (as above), it returns 0 because it is an undefined array and the third return statement is executing.
I don't understand this part though:
if (typeof array === "string") {
return 0;
}
if (typeof array === "number") {
return 0;
}
What would these statements do to an array to make it return 0 and what would my_array need to look like in order for them to trigger?
4 Answers
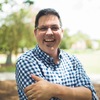
Dave McFarland
Treehouse TeacherWith the code you show, you won't ever pass any of those conditional statements. That's because you ARE passing an array. However, check out this code:
function arrayCounter(array) {
if (typeof array === "string") {
return 0;
}
if (typeof array === "number") {
return 0;
}
if (typeof array === "undefined") {
return 0;
} else {
return array.length;
}
};
var my_array = [ 1,2,3 ];
console.log(arrayCounter(my_array)); // 3
var name = "dave";
console.log(arrayCounter(name)); // returns 0, because it's a string
var age = "22";
console.log(arrayCounter(name)); // returns 0, because it's a number
var nothing;
console.log(arrayCounter(nothing)); // returns 0, because the var is not defined

Jason Anello
Courses Plus Student 94,610 PointsHi Bob,
Your my_array
variable isn't an undefined array it is an empty array. Meaning an array whose length is zero. You have an array it just doesn't have any elements in it yet. So you're actually executing the else
block there and returning the length of the array. You've discovered one of the problems with this function. If the function returns 0, you don't know whether that's because the array was empty or if one of the other 3 values were passed in.
The 3 if blocks are for checking if you've tried to pass something else into the function that's not an array.
For example,
var myString = "some string";
console.log(arrayCounter(myString)); // logs 0 because the type of the argument was a "string"
var myNumber = 45;
console.log(arrayCounter(myNumber)); // logs 0 because the type of the argument was a "number"
var myUndefinedVariable;
console.log(arrayCounter(myUndefinedVariable)); // logs 0 because the type of the argument was "undefined"
You used the example of the array containing numbers or strings but it doesn't matter what the array contains. It could contain a bunch of boolean values and this function should still return its length.
So the idea here is that before returning the length of the array, you want to make sure that what was passed in is actually an array and not one of those other values.
The solution you posted above is essentially what the challenge wanted you to come up with based on what you know so far.
This function isn't very robust and has a few problems. One of them was already mentioned. If the function returns 0, it's not clear why it returned 0.
The other problem is that you could pass a boolean, or a function, or an object into this function and it's going to try to return the length of those arguments because you're not specifically checking against those.
George Cristian Manea mentioned the .isArray
method. This would be a more robust solution but beyond what is taught.
function arrayCounter(array) {
if(Array.isArray(array)){
{
return array.length;
}
return 0; // It must not have been an array.
}
A better final return value might be -1 instead. This avoids the ambiguity mentioned earlier of returning 0. If a -1
is returned, you know it wasn't an array. If a zero is returned, you know it was an array whose length was zero.

Bob Goldsmith
5,444 PointsThank you Jason for explaining that so fully. Much appreciated.

Jason Anello
Courses Plus Student 94,610 PointsGlad it helped.
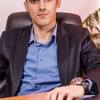
George Cristian Manea
30,787 PointsActually you can not do that, "typeof" is used on variables or objects, if you want to check if an array is an array you can use this function if(Array.isArray(your_array)){
return "ok";
}
. The one with the string and number can be trigger if you use a simple variable.
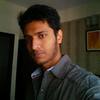
Ajinkya Borade
Courses Plus Student 16,635 Pointsfunction arrayCounter(item) {
if (typeof item === "string") {
return 0;
}
if (typeof item === "number") {
return 0;
}
if (typeof item === "undefined") {
return 0;
} else {
return item.length;
}
};
var my_array = [];
console.log(arrayCounter(my_array));//returns 0 as lengh of your array it is zero
It is not returning zero becasue its 'undefined', but cus its an zero length array.
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 PointsDave beat me to it.
It looks like we had the same train of thought too with the code example.