Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial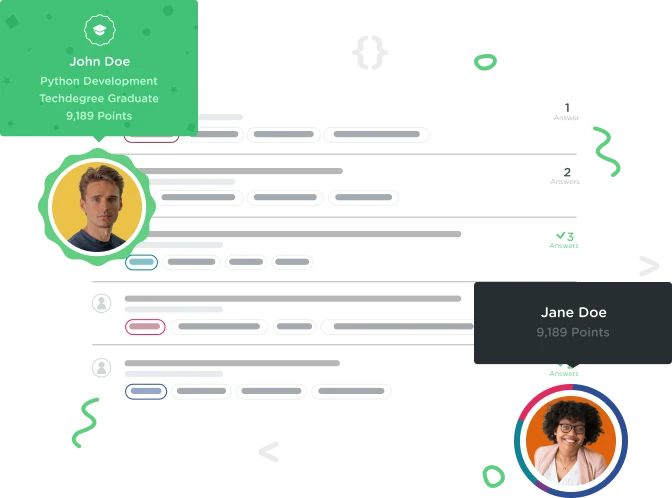
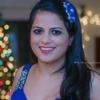
Mona Jalal
4,302 Pointsreturned []
import re
EXAMPLE:
>>> find_words(4, "dog, cat, baby, balloon, me")
['baby', 'balloon']
def find_words(count, string): return re.findall(r'\w*count',string)
or
import re
EXAMPLE:
>>> find_words(4, "dog, cat, baby, balloon, me")
['baby', 'balloon']
def find_words(count, string): return re.findall(r'\w{count}',string)
both return [] why?
import re
# EXAMPLE:
# >>> find_words(4, "dog, cat, baby, balloon, me")
# ['baby', 'balloon']
def find_words(count, string):
return re.findall(r'\w*count',string)
2 Answers
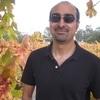
Kourosh Raeen
23,733 PointsHi Mona - The problem is that count
is inside quotes so the number passed into the function is not used. Try creating the pattern using string concatenation:
import re
# EXAMPLE:
# >>> find_words(4, "dog, cat, baby, balloon, me")
# ['baby', 'balloon']
def find_words(count, string):
return re.findall(r'\w{' + str(count) + ',}', string)
Notice that I am casting count
to a string. Also, notice the comma after count
. That is needed since we want words that are count
characters or longer. Without it you'd get those words that are exactly count
characters long.

Unsubscribed User
7,260 PointsHi Kourosh,
I'm in the same boat as Mona. I do not understand why we have to convert count to a string.. In the examples and the video we see that count is a number be it 1, 2, 3,... When they say count number of times, for me it means that count is the same as saying x-amount of times.
I don't understand at all why we are converting a number to a string to input it in the location where a number should be put..
import re
# EXAMPLE:
# >>> find_words(4, "dog, cat, baby, balloon, me")
# ['baby', 'balloon']
def find_words(count, string):
return re.findall(r'\w{count,}', string)
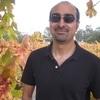
Kourosh Raeen
23,733 PointsHi Kevin - count
is a variable but once you put it inside quotes, as in your code above, then python just treats that as part of that string so its value doesn't get substituted there. it's like the following code:
count = 5
print(count)
print('count')
The first print statement prints 5, but the second one just prints the string "count". So count should be outside the single quotes so that you can get its value. Now, why convert the number to a string? Because the whole pattern we are trying to match needs to be a string. Notice that in the videos the number that is used is inside the single quotes and part of the pattern to match, which is a string. So we need the value of the variable count
but then we need to concatenate that to the other strings to create the pattern and if you don't convert that to a string python will complain.
Hope this makes sense.