Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial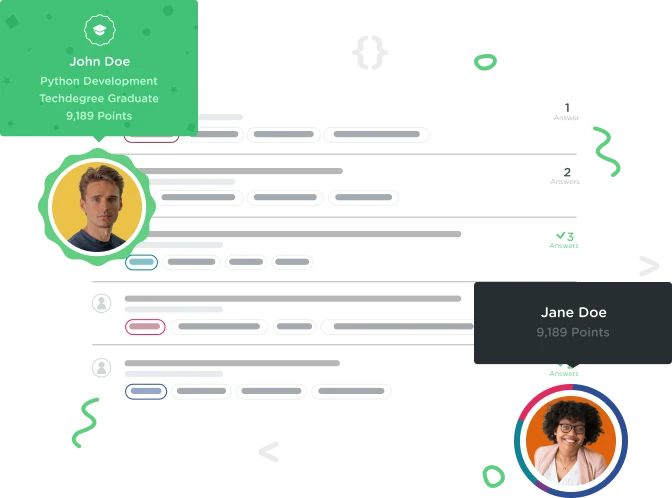

Luka Nixon
2,303 PointsReturning and retrieving a string.
So im trying to return the name in my StoryActivity.java and then call it in my Story.java file, im trying to return userName. here are the files :
public class StoryActivity extends Activity{
private Story mStory = new Story();
private ImageView mImageView;
private TextView mTextView;
private Button mChoice1;
private Button mChoice2;
private static String mName;
private Page mCurrentPage;
public static final String TAG = StoryActivity.class.getSimpleName();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_story);
Intent intent= getIntent();
mName = intent.getStringExtra(getString(R.string.key_name));
if (mName == null){
mName = "Friend";
}
mImageView = (ImageView)findViewById(R.id.storyImageView);
mTextView = (TextView)findViewById(R.id.storyTextView);
mChoice1 = (Button)findViewById(R.id.choiceButton1);
mChoice2 = (Button)findViewById(R.id.choiceButton2);
loadPage(0);
}
public static String getName(String userName){
userName = mName;
return userName;
}
private void loadPage(int choice){
mCurrentPage= mStory.getPage(choice);
Drawable drawable = getResources().getDrawable(mCurrentPage.getmImageId());
mImageView.setImageDrawable(drawable);
String pageText= mCurrentPage.getmText();
pageText = String.format(pageText, mName);
mTextView.setText(mCurrentPage.getmText());
if(mCurrentPage.ismIsFinal()){
mChoice1.setVisibility(View.INVISIBLE);
mChoice2.setText("PLAY AGAIN");
mChoice2.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
finish();
}
});
}
else{
mChoice1.setText(mCurrentPage.getmChoice1().getmText());
mChoice2.setText(mCurrentPage.getmChoice2().getmText());
mChoice1.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
int nextPage = mCurrentPage.getmChoice1().getmNextPage();
loadPage(nextPage);
}
});
mChoice2.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
int nextPage = mCurrentPage.getmChoice2().getmNextPage();
loadPage(nextPage);
}
});
}
}
}
Then here is my
package model;
import com.lukan.signalsfrommars.R;
import ui.StoryActivity;
public class Story {
private Page[] mPages;
public Story() {
String userName = StoryActivity.getName(userName);
String name = userName;
mPages = new Page[7];
mPages[0] = new Page(
R.drawable.page0,
"On your return trip from studying Saturn's rings, you hear a distress signal that seems to be coming from the surface of Mars. It's strange because there hasn't been a colony there in years. Even stranger, it's calling you by name: \"Help me,"+ name +", you're my only hope.\"",
new Choice("Stop and investigate", 1),
new Choice("Continue home to Earth", 2));
mPages[1] = new Page(
R.drawable.page1,
"You deftly land your ship near where the distress signal originated. You didn't notice anything strange on your fly-by, but there is a cave in front of you. Behind you is an abandoned rover from the early 21st century.",
new Choice("Explore the cave", 3),
new Choice("Explore the rover", 4));
mPages[2] = new Page(
R.drawable.page2,
"You continue your course to Earth. Two days later, you receive a transmission from HQ saying that they have detected some sort of anomaly on the surface of Mars near an abandoned rover. They ask you to investigate, but ultimately the decision is yours because your mission has already run much longer than planned and supplies are low.",
new Choice("Head back to Mars to investigate", 4),
new Choice("Continue home to Earth", 6));
mPages[3] = new Page(
R.drawable.page3,
"Your EVA suit is equipped with a headlamp, which you use to navigate the cave. After searching for a while your oxygen levels are starting to get pretty low. You know you should go refill your tank, but there's a very faint light up ahead.",
new Choice("Refill at ship and explore the rover", 4),
new Choice("Continue towards the faint light", 5));
mPages[4] = new Page(
R.drawable.page4,
"The rover is covered in dust and most of the solar panels are broken. But you are quite surprised to see the on-board system booted up and running. In fact, there is a message on the screen: \""+ name +", come to 28.543436, -81.369031.\" Those coordinates aren't far, but you don't know if your oxygen will last there and back.",
new Choice("Explore the coordinates", 5),
new Choice("Return to Earth", 6));
mPages[5] = new Page(
R.drawable.page5,
"After a long walk slightly uphill, you end up at the top of a small crater. You look around, and are overjoyed to see your favorite android, %1$s-S1124. It had been lost on a previous mission to Mars! You take it back to your ship and fly back to Earth.");
mPages[6] = new Page(
R.drawable.page6,
"You arrive home on Earth. While your mission was a success, you forever wonder what was sending that signal. Perhaps a future mission will be able to investigate...");
}
public Page getPage(int pageNumber){
return mPages[pageNumber];
}
}
1 Answer

Daniel Hartin
18,106 PointsHi Luka
Objects or classes are not references to one single entity a class is like a blueprint, a design sheet of how an object is supposed to work. When you create an object and change variables based on that class the blueprint doesn't change only the newly created object does.
Imagine we have a book full of blank pages (your storyActivity) as you start writing the pages gain values (like setting variables) if we say later in the code get me a new storyActivity the code returns a new book full of blank pages, (I hope this makes sense lol). If we want those variable values we need to pass them along somehow (there are various methods like creating a holder class that extends Serializable or Parcelable, but these are more advanced) I think that easiest way would be to pass the username to the story class using a constructor method like the below
public class StoryActivity extends Activity{
private Story mStory;
private ImageView mImageView;
private TextView mTextView;
private Button mChoice1;
private Button mChoice2;
private static String mName;
private Page mCurrentPage;
public static final String TAG = StoryActivity.class.getSimpleName();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_story);
Intent intent= getIntent();
mName = intent.getStringExtra(getString(R.string.key_name));
if (mName == null){
mName = "Friend";
}
mStory = new Story(mName); //here we have changed the new class to take a string parameter
mImageView = (ImageView)findViewById(R.id.storyImageView);
mTextView = (TextView)findViewById(R.id.storyTextView);
mChoice1 = (Button)findViewById(R.id.choiceButton1);
mChoice2 = (Button)findViewById(R.id.choiceButton2);
loadPage(0);
}
public static String getName(String userName){
userName = mName;
return userName;
}
private void loadPage(int choice){
mCurrentPage= mStory.getPage(choice);
Drawable drawable = getResources().getDrawable(mCurrentPage.getmImageId());
mImageView.setImageDrawable(drawable);
String pageText= mCurrentPage.getmText();
pageText = String.format(pageText, mName);
mTextView.setText(mCurrentPage.getmText());
if(mCurrentPage.ismIsFinal()){
mChoice1.setVisibility(View.INVISIBLE);
mChoice2.setText("PLAY AGAIN");
mChoice2.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
finish();
}
});
}
else{
mChoice1.setText(mCurrentPage.getmChoice1().getmText());
mChoice2.setText(mCurrentPage.getmChoice2().getmText());
mChoice1.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
int nextPage = mCurrentPage.getmChoice1().getmNextPage();
loadPage(nextPage);
}
});
mChoice2.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
int nextPage = mCurrentPage.getmChoice2().getmNextPage();
loadPage(nextPage);
}
});
}
}
}
And the story class would be like...
package model;
import com.lukan.signalsfrommars.R;
public class Story {
private Page[] mPages;
private String name;
public Story(String username) { //Here is where the new constructor receives the string from your StoryActivty which we can //store in a member variable (I used the naming convention mName as it would mean altering all your reference in your text)
name = username;
mPages = new Page[7];
mPages[0] = new Page(
R.drawable.page0,
"On your return trip from studying Saturn's rings, you hear a distress signal that seems to be coming from the surface of Mars. It's strange because there hasn't been a colony there in years. Even stranger, it's calling you by name: \"Help me,"+ name +", you're my only hope.\"",
new Choice("Stop and investigate", 1),
new Choice("Continue home to Earth", 2));
mPages[1] = new Page(
R.drawable.page1,
"You deftly land your ship near where the distress signal originated. You didn't notice anything strange on your fly-by, but there is a cave in front of you. Behind you is an abandoned rover from the early 21st century.",
new Choice("Explore the cave", 3),
new Choice("Explore the rover", 4));
mPages[2] = new Page(
R.drawable.page2,
"You continue your course to Earth. Two days later, you receive a transmission from HQ saying that they have detected some sort of anomaly on the surface of Mars near an abandoned rover. They ask you to investigate, but ultimately the decision is yours because your mission has already run much longer than planned and supplies are low.",
new Choice("Head back to Mars to investigate", 4),
new Choice("Continue home to Earth", 6));
mPages[3] = new Page(
R.drawable.page3,
"Your EVA suit is equipped with a headlamp, which you use to navigate the cave. After searching for a while your oxygen levels are starting to get pretty low. You know you should go refill your tank, but there's a very faint light up ahead.",
new Choice("Refill at ship and explore the rover", 4),
new Choice("Continue towards the faint light", 5));
mPages[4] = new Page(
R.drawable.page4,
"The rover is covered in dust and most of the solar panels are broken. But you are quite surprised to see the on-board system booted up and running. In fact, there is a message on the screen: \""+ name +", come to 28.543436, -81.369031.\" Those coordinates aren't far, but you don't know if your oxygen will last there and back.",
new Choice("Explore the coordinates", 5),
new Choice("Return to Earth", 6));
mPages[5] = new Page(
R.drawable.page5,
"After a long walk slightly uphill, you end up at the top of a small crater. You look around, and are overjoyed to see your favorite android, %1$s-S1124. It had been lost on a previous mission to Mars! You take it back to your ship and fly back to Earth.");
mPages[6] = new Page(
R.drawable.page6,
"You arrive home on Earth. While your mission was a success, you forever wonder what was sending that signal. Perhaps a future mission will be able to investigate...");
}
public Page getPage(int pageNumber){
return mPages[pageNumber];
}
This way the value is passed along the chain of objects we create and we don't need to reference back to another object.
I hope this made sense, some of the concepts of OOP are a little confusing at first.
I haven't tested any of the code here so let me know if something doesn't work and I'll try to take a look
Daniel
}
Luka Nixon
2,303 PointsLuka Nixon
2,303 PointsThank you it works great!