Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial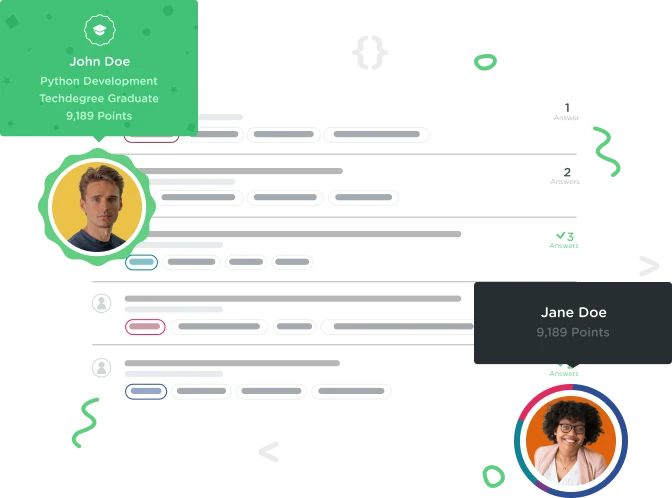

Dickson Cheung
2,573 PointsReturning Complex Values - Objective 1
Eiffel Tower - lat: 48.8582, lon: 2.2945 Great Pyramid - lat: 29.9792, lon: 31.1344 Sydney Opera House - lat: 33.8587, lon: 151.2140
Declare a function named coordinates that takes a single parameter of type String, with an external name for, a local name of location, and returns a tuple containing two Double values (Note: You do not have to name the return values). For example, if I use your function and pass in the string "Eiffel Tower" as an argument, I should get (48.8582, 2.2945) as the value. If a string is passed in that doesnβt match the set above, return (0,0)
So I understand that my switch is not actually returning a valid action but i don't know how to take the doubles given and return them through the function. Would anybody please help or point me in the right direction?
Thanks
// Enter your code below
func coordinates (for location: String) -> (Double, Double) {
switch location {
case "Eiffel Tower": (48.8582,2.2945)
case "Great Pyramid": (29.9792,31.1344)
case "Syndey Opera House":(33.8587,151.2140)
default: (0,0)
}
return (location)
}
2 Answers

andren
28,558 PointsYour code is already extremely close to the correct solution. Returning the values is actually extremely simple. You just need to use the return
keyword. return
can return a double value by itself, and if you use it within a conditional statement like a switch clause it can be used multiple times in a method.
So all you need to do is place the return
keyword before the values. The first case should look like this for example:
case "Eiffel Tower": return (48.8582,2.2945)
And the same goes for the other clauses, including the default
clause. You can then remove the return location
line you currently have in your code as it won't be needed anymore.
Do note though that you have a typo your third switch
clause. You have typed Syndey when you meant to type Sydney. You have to fix that typo in order to complete the challenge.

michael pichler
866 PointsWhere exactly are those values saved? (48.8582, 2.2945) Like, if I wanted to assign it to a constant? Wouldn't that only be possible with an array? This kinda confused me. I did the challenge like this, which seems to be way to complicated, but it worked [code] // Enter your code below func coordinates(for location: String) -> (Double, Double) {
let eiffeltower = [48.8582, 2.2945] let greatpyramid = [29.9792, 31.1344] let sydneyoperahouse = [33.8587, 151.2140] var lon: Double = 0 var lat: Double = 0 switch location { case "Eiffel Tower": lon = eiffeltower[0]; lat = eiffeltower[1] case "Great Pyramid": lon = greatpyramid[0]; lat = greatpyramid[1] case "Sydney Opera House": lon = sydneyoperahouse[0]; lat = sydneyoperahouse[1] default: lon = 0; lat = 0
} return (lon, lat) } [/code]