Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial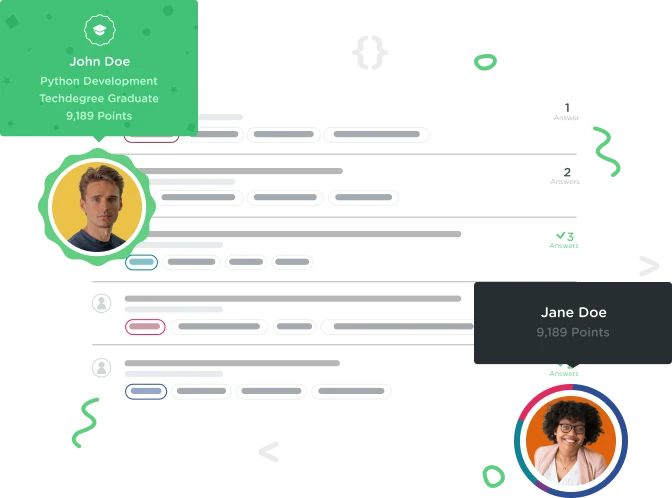

Jacinda Zhong
3,695 PointsReturning from a proc vs. lambda
The correct answer to the question "what does the return keyword do in a lambda?" is "Returns from the lambda", but doesn't the a lambda actually go back to the parent method, from which it was called? Maybe it's the wording of this answer that is confusing me. In the code example from Jason, the returned value was the puts statement in the parent method, not in the lambda. So wouldn't the correct answer be "Returns from the calling method"?
2 Answers
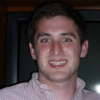
Richard Luick
10,955 PointsI struggled to understand this at first since the wording was a little confusing but I think I was able to figure it out so I'll do my best to answer (eventhough this post is a couple months old).
I believe he is saying that when return is called in the proc, then that return value is used as the return for the proc and the entire method. Therefore, the rest of the method is not executed after the proc. However, in a lambda, it will return only from the lambda and then continue to execute the rest of the method. Since the rest of the method is executed, the return value that you see is going to be the last line of the method.
That was my interpretation of the video so I hope this helps!
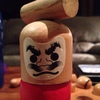
Eric Carey
6,820 PointsWell, it helped me out. The wording was indeed tricky. I'll add this for anyone who hits this question in the future.
It might help you to add "puts" before variable.call like this:
def return_from_proc
variable = proc { return "Returning from proc inside method" }
puts variable.call
return "Returning from proc as last line from method"
end
def return_from_lambda
variable = lambda { return "Returning from lambda inside method" }
puts variable.call
return "Returning from lambda as last line from method"
end
If you do that, you'll see that in return_from_proc, literally nothing runs after the "proc { return...". In return_from_lambda, however, you get both sentences.
My non-perfect understanding is that this happens because lambdas are essentially methods, but procs are just convenient snippets of code. This is why procs don't throw an error if you pass them an incorrect number of arguments. They aren't methods, so they don't have any of the functionality and checks that you get with a method.
Lambdas on the other hand are methods and so have their own return value, require the right number of arguments, etc. Really you could re-write the return_from_lambda method like this:
def return_from_pseudo_lambda
def pseudo_lambda
return "Returning from lambda inside method"
end
puts pseudo_lambda()
return "Returning from lambda as last line from method"
end
puts return_from_pseudo_lambda
You'll get the same output, but you had to write way more code.
So in short, think of lambdas as a kind of anonymous/un-named method that can speed up the writing of your code, whereas procs are simply convenient groups of code.