Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial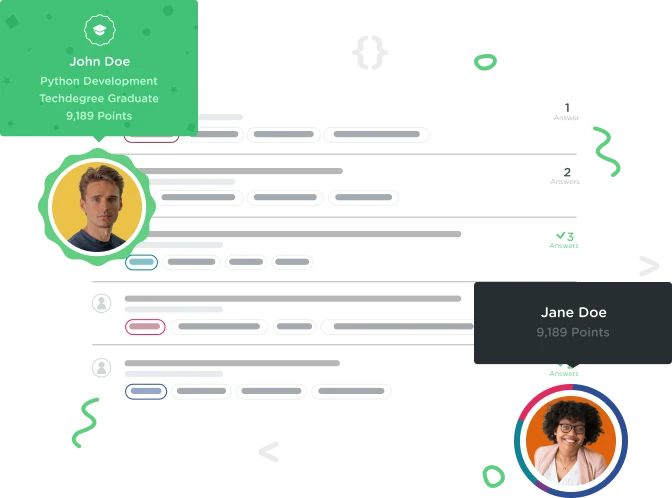
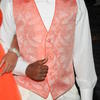
Rashii Henry
16,433 PointsReturning Integers in a string?
why does the following code print out the numbers 49 - 54 in ascending order?
where is the console retrieving these values?
- (void)viewDidLoad
{
[super viewDidLoad];
_nString = [NSString stringWithFormat:@"123456"];
for (int i = 0; i < _nString.length; i++)
{
NSLog(@"%i",[_nString characterAtIndex:i]);
}
}
2 Answers

Don Miller
1,773 PointsYou may want to cast it as an integer or make it an integer. Otherwise, Michael is correct that the value of @"1" is a unicode value, not the number 1.
- (void)viewDidLoad
{
[super viewDidLoad];
_nString = [NSString stringWithFormat:@"123456"];
for (int i = 0; i < (int)_nString.length; i++)
{
NSLog(@"%i",[_nString characterAtIndex:i]);
}
}
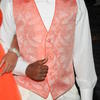
Rashii Henry
16,433 PointsThanks to you both.
I just want to be able to return the lowest number in any given string.
Im not sure if I'm taking the right approach. I was going to use containsString.
I've casted the length in the for loop to an int like you did above and I'm still receiving ASCII characters in the console.
I also tried adding intValue at the end of the declaration. but implicit conversions aren't allowed with ARC.

Michael Owens
5,591 PointsBy lowest number, I'm assuming lowest single digit number. First your for loop isn't parsing the string correctly. There are many ways to do this.
Second you may have to typecast the output as well.
NSLog(@"%i",[(int)_nString characterAtIndex:i]);
hope that helps
Michael Owens
5,591 PointsMichael Owens
5,591 PointsIt is returning the characters from your string "123456" in ASCII value
In other words:
49 = '1'
50 = '2'
51 = '3'
.
.
.
For a look at the ASCII table look here: http://www.asciitable.com/