Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial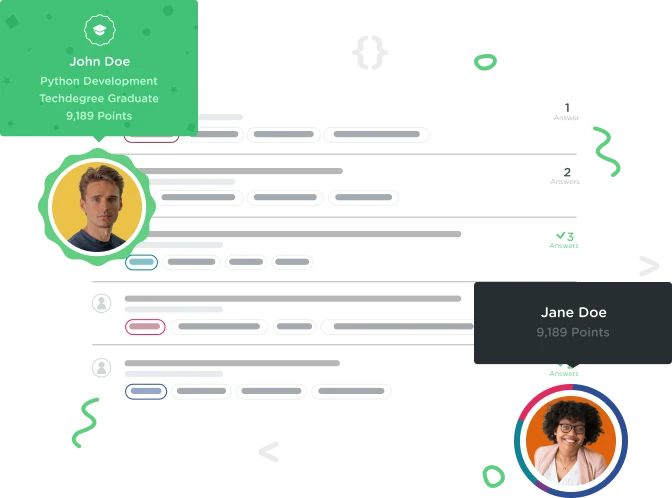

Solomon Alexandru
3,633 PointsReturning sum of object parameters
I've been working on a project where I have to create a method that returns the sum of two objects. public class Money {
private final int euros;
private final int cents;
public Money(int euros, int cents) {
if(cents > 99) {
euros += cents /100;
cents %= 100;
}
this.euros = euros;
this.cents = cents;
}
public int euros() {
return euros;
}
public int cents() {
return cents;
}
public String toString() {
String zero = "";
if (cents<= 10) {
zero = "";
}
return euros + "." + zero + cents + "e";
}
public Money plus(Money added) {
return new Money(this.euros, this.cents) + added; // THIS IS THE TROUBLE
}
}
I want to use the method like this:
public class AplicatiaDoi {
public static void main(String[] args) {
Money a = new Money(10, 0);
Money b = new Money(5, 0);
Money c = a.plus(b);
System.out.println(a);
System.out.println(b);
System.out.println(c);
a = a.plus(c);
System.out.println(a);
System.out.println(b);
System.out.println(c);
}
}
Is there an easy way to do it?
2 Answers
Rebecca Rich
Courses Plus Student 8,592 PointsHi Solomon,
From what you have written right now, here is how you could think about public Money plus(Money added) { ... }
:
Inside of the plus method you have access to the Money object you are calling plus on (i.e. this) as well as the Money object that is getting added to that object (added). You also have access to your getter methods euros()
and cents()
. What you need to do is add the euros together and then add the cents together and create a new Money object.
Starting with the euros, we know that we are adding this.euros
and added.euros()
.
Similarly with the cents, we know that we are adding this.cents
and added.cents()
.
You have written your Money constructor so that it will take care of any issues with cents > 99
so we can simply create the plus method as such:
public Money plus(Money added) {
return new Money(this.euros + added.euros(), this.cents + added.cents());
}

Solomon Alexandru
3,633 PointsThank you a lot for your solution. I wrote another syntax, but yours is simpler. If you're curios, here is the syntax:
public Money plus(Money y) {
Money m = new Money(euros, cents);
int totalCents = y.cents + this.cents;
int totalEuros = y.euros + this.euros;
if (totalCents > 100) {
totalEuros = totalEuros + (int) (totalCents / 100);
totalCents = (int) (totalCents % 100);
}
m.euros = totalEuros;
m.cents = totalCents;
return m;
}