Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial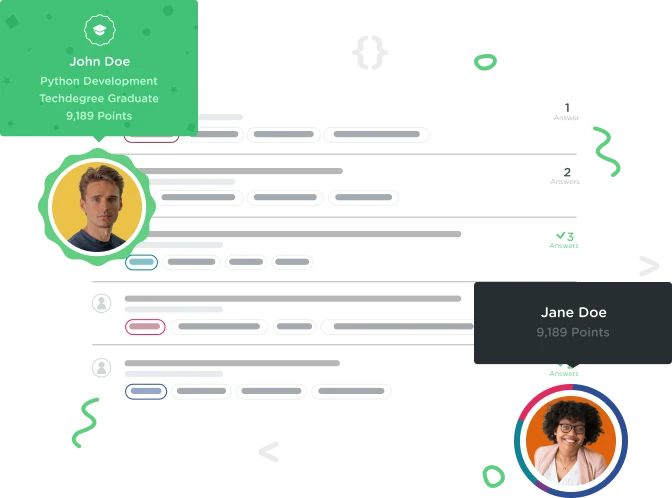

Stefan Vaziri
17,453 PointsReturning the oldest timestamp
I've seen other people's answer in the Community section but I wanted to see if there's a different way to answer this. I got "Bummer! 'function' object is not iterable." Can you tell me what I can do to fix that?
There were some other way to do it (i.e. sorting with min and max method) and I also saw some people use lambda (not in Treehouse but on other python Q&A websites). What is the best way to solve this? Also, Kenneth went over it but can someone explain to me what the difference is between strptime and strftime? He said strptime is for parsing time but I don't really understand it as well as I should.
Thanks in advance!
# If you need help, look up datetime.datetime.fromtimestamp()
# Also, remember that you *will not* know how many timestamps
# are coming in.
import os
import datetime
def timestamp_oldest(dates, *args):
for dates in timestamp_oldest:
dates = datetime.datetime.strftime(dates, '%d %B %Y')
return dates.sorted[-1]
3 Answers
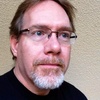
Chris Freeman
Treehouse Moderator 68,423 PointsThis challenge uses POSIX time values (floats) as arguments. Using strptime
and strftime
doesn't apply as they convert between strings and datetime
objects.
datetime.strftime(format)
Return a string representing the date and time, controlled by an explicit format string.
classmethod datetime.strptime(date_string, format)
Return a datetime corresponding to date_string, parsed according to format. This is equivalent to datetime(*(time.strptime(date_string, format)[0:6])). ValueError is raised if the date_string and format canβt be parsed by time.strptime() or if it returns a value which isnβt a time tuple.
For a complete list of formatting directives, see section strftime() and strptime() Behavior
Beyond not using strftime
there were two errors in your code structure.
-
The challenge specifies only one parameter: the arbitrary number of POSIX times. So the function definition should look like:
def timestamp_oldest(*args):
-
In your code you refence the function name as an Iterable
for dates in timestamp_oldest:
This should refer to the function parameters:
for dates in args:
The best approach is to sort the POSIX numbers, lowest number is the oldest. Convert the oldest to a datetime using the fromtimestamp()
method. Return this datetime. Post back if you want the actual code.

Jason Whitaker
12,252 PointsI was able to solve it the following way:
import datetime
def timestamp_oldest(*args):
timestamp_list = []
for arg in args:
timestamp_list.append(arg)
timestamp_list.sort()
return datetime.datetime.fromtimestamp(timestamp_list[0])
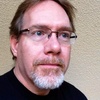
Chris Freeman
Treehouse Moderator 68,423 PointsNicely done. You can take advantage of the power in the "*args
" parameter to get a list without having to loop through it to produce your own version.
The loop can be replaced with a simple conversion:
import datetime
def timestamp_oldest(*args):
# This:
# timestamp_list = []
# for arg in args:
# timestamp_list.append(arg)
#
# Replaced by:
timestamp_list = list(args)
timestamp_list.sort()
return datetime.datetime.fromtimestamp(timestamp_list[0])

Stefan Vaziri
17,453 PointsHere's what I entered and passed:
import os
import datetime
def timestamp_oldest(*args):
return datetime.datetime.fromtimestamp(min(args))
Is there a way to do it with the for loop like how I did in the beginning? I couldn't really figure it out with the for loop adn this one is more concise.
Thanks Chris!
[MOD: Added ```python markdown formatting -cf]

Stefan Vaziri
17,453 PointsHm I see. Yea using the min function is definitely easier.
Thanks again!!
Chris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 PointsIt is possible to use a
for
loop but you might end up just implementing the functionality of a built-in function.This is basically the same functionality as
min()
.