Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial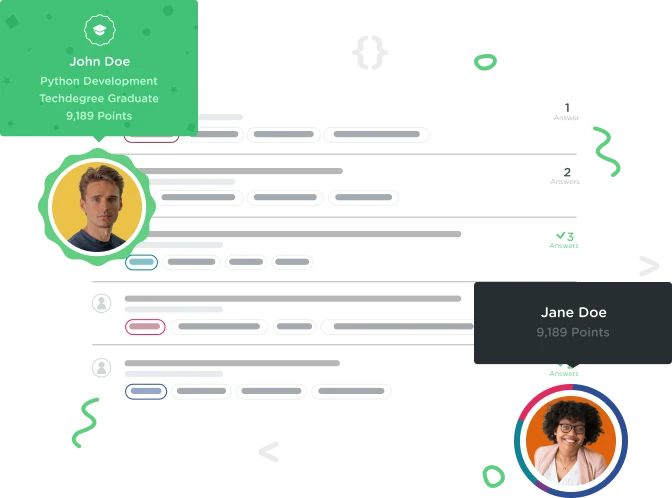

Cody Smith
4,510 PointsReturning the program to where it threw an acception
I'm trying to do the Arithmetic Calculator Practice Project in C#. Below is what I've come up with:
using System;
namespace Treehouse.Calculator { class Calculator { static void Main() {
bool calculator = true;
while (calculator)
{
//Prompt user to enter the first number in their calculation
Console.Write("Enter the first number in your operation: ");
string entry1 = Console.ReadLine();
if(entry1.ToLower () == "quit")
{
return;
}
else
{
try
{
double number1 = double.Parse(entry1);
//Prompt user to enter an operation
Console.Write("Enter an operator ( +, -, *, or /): ");
string sign = Console.ReadLine();
if(sign == "quit")
{
return;
}
else
{
//Promt user for another number
Console.Write("Enter the second number: ");
string entry2 = Console.ReadLine();
double number2 = double.Parse(entry2);
if(entry2 == "quit")
{
return;
}
else
{
//Print results to the screen
if(sign == "+")
{
Console.WriteLine(number1 + " + " + number2 + " = " + (number1 + number2));
}
else if(sign == "-")
{
Console.WriteLine(number1 + " - " + number2 + " = " + (number1 - number2));
}
else if(sign == "*")
{
Console.WriteLine(number1 + " * " + number2 + " = " + (number1 * number2));
}
else if(sign == "/")
{
Console.WriteLine(number1 + " / " + number2 + " = " + (number1 / number2));
}
}
}
}
catch(FormatException)
{
Console.WriteLine("That is not valid input.");
continue;
}
}
//Repeat until the user types "quit" at any of the prompts.
Console.WriteLine("Goodbye");
}
}
} }
Two things: 1) When you enter an invalid character into any of the questions, it will tell me "That is not a valid input" and return me to the first question. I want it to return to the question that you entered the incorrect info on. So if on question 2 "Enter an operator ( +, -, *, or /): " I entered hi, it would say "That is not valid input." and return to that question.
2) What can be cleaned up to make it look cleaner. It's looking long and bulky.
Thanks!
1 Answer
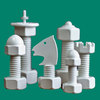
Steven Parker
231,269 PointsTo prevent starting completely over on an error, you could have a separate retry loop with it's own "try" and "catch" for each question.
And to make the code more compact, you could look for places where similar things are done more than once and create and call a function to do them. Prompting for and inputting a number might be a possible candidate.