Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial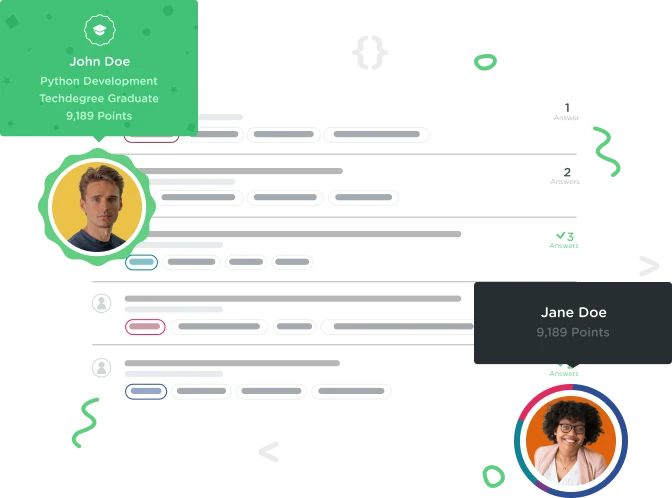

Leo Marco Corpuz
18,975 Pointsreverse evens
Can't solve the last part of this challenge
def first_4(numberSet):
return numberSet[0:4]
def first_and_last_4(numberSet):
return numberSet[:4] + numberSet[-4:]
def odds(numberSet):
return numberSet[1::2]
def reverse_evens(numberSet):
return numberSet[-2::-2]
1 Answer
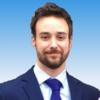
adamzemmoura
29,481 PointsYou can break this task down into two parts.
1) Get all the numbers corresponding to even indexes. Note: itβs even indexes here so requires a step of 2 eg. Index 0, then 2, then 4 and so on. We can do this using slices by leaving the start and stop blank, which gives us the default behaviour of starting at the beginning and going all the way to end and specifying a step of 2 to get only the even indexes.
numberSet[::2]
2) Reverse the list returned from step 1. Using slices to do this we can again leave the start and stop blank as we did in step 1 and specify -1 as the step, which means : start at the end and go through the list backwards, which is one way to reverse the order.
numberSet[::-1]
Steps 1 and 2 can be combined on one line making your function :
def reverse_evens(numberSet):
return numberSet[::2][::-1]
Hope this helps.
Leo Marco Corpuz
18,975 PointsLeo Marco Corpuz
18,975 PointsThanks!