Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial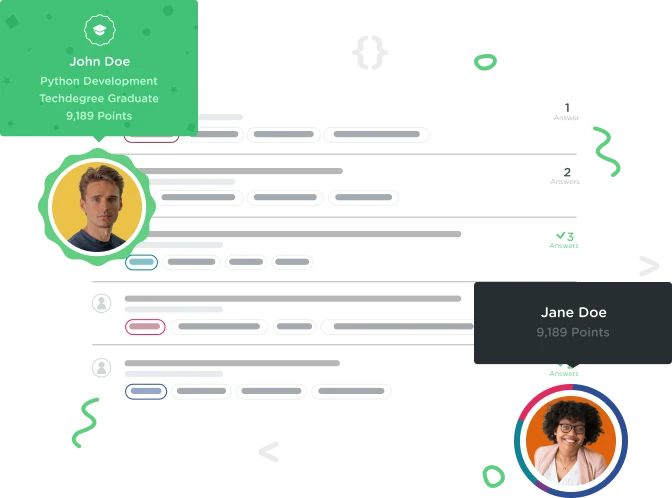
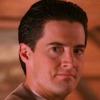
akoniti
1,410 PointsReverse Evens - Code works, but does not pass check
This Python challenge requests:
"Make a function named reverse_evens that accepts a single iterable as an argument. Return every item in the iterable with an even index...in reverse.
For example, with [1, 2, 3, 4, 5] as the input, the function would return [5, 3, 1]."
Despite my code doing just this when tested in the terminal (returning 531 if the input is 12345) , when I check the work the page indicates it "Didn't get the right results" from the reverse_evens function.
Am I misunderstanding the challenge, or have I made a simple error I've overlooked?
def first_4(iterable):
list(iterable)
data_out = iterable[0:4]
print(data_out)
return data_out
def first_and_last_4(iterable):
list(iterable)
data_out_1 = iterable[0:4]
data_out_2 = iterable[-4:]
final_data = data_out_1 + data_out_2
return final_data
def odds(iterable):
list(iterable)
data_out = iterable[1::2]
return data_out
def reverse_evens(iterable):
list(iterable)
data_out = iterable[-1::-2]
return data_out
3 Answers
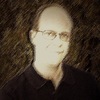
Jason Anders
Treehouse Moderator 145,860 PointsHi there,
Yes, that code works for a list with an odd number of items (eg. 1, 2, 3, 4, 5), but did you test it with a list with a even number of items (eg. 1, 2, 3, 4, 5, 6)? It won't return what the task is looking for.
Hint: for this task, you'll need to employ the use of an if statement
to check the length of the list and then use the appropriate slice, whether the list length is odd or even.
I'm sure you'll get it now. Nice work!
:)
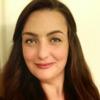
Jennifer Nordell
Treehouse TeacherHi there, akoniti ! You've understood the challenge just fine, but seems you forgot something. The problem here isn't so much with your code but with your test data. Your code returns the correct thing in about half the cases. Namely, it returns the correct thing when there is an odd number of elements in the iterable.
If I were to send in [1, 2, 3, 4, 5, 6]
instead of just the one to 5, I would get back [6, 4, 2]
, but 6, 4, and 2 all reside at odd indexes. My suggestion is to first get all even items and then reverse the list.
Hope this helps!
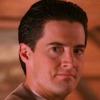
akoniti
1,410 PointsThanks for the quick help!
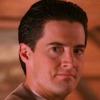
akoniti
1,410 PointsThank you Jason and Jennifer!!
I see now that I was too narrowly focused on making the function do just what the single example showed, instead of doing a little critical thinking about the full requirement, and how my function fell short of meeting it.
Now it is solved:
def reverse_evens(iterable):
list(iterable)
test = len(iterable)
if test % 2 == 0:
data_out = iterable[-2::-2]
else:
data_out = iterable[-1::-2]
return data_out
Thanks again for the super quick help!
akoniti
1,410 Pointsakoniti
1,410 PointsThanks for the quick help!