Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial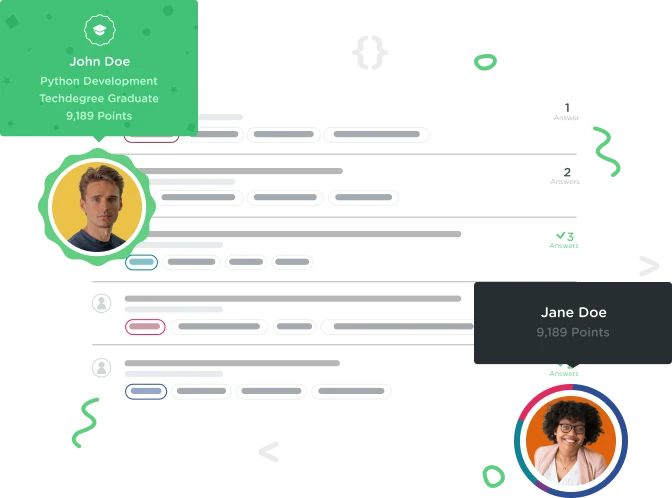

Hinh Phan
2,564 PointsREVERSE GAME HELP: I can't run my code. Spot my fault pls.
import random
def replay():
play_again = input("Let try again? Y/n")
if play_again.upper() == 'Y':
game()
else:
print("See you again")
break
def computer_answer(low, high):
return random.randint(low, high)
def game():
low = int(input("Pick your min number: "))
high = int(input("Pick your max number: "))
secret_num = int(input("Pick a number between {} and {} for computer to guess: ".format(low, high))
print("Computer, you got 5 times to guess. Let's go.")
limit_guess = 5
computer_guesses = []
while limit_guess < 5:
try:
computer_guess = computer_answer(low, high)
except ValueError:
print("Computer didn't get it")
continue
else:
if computer_guess == secret_num:
print("You win the game. It was {}.".format(secret_num))
break
elif computer_guess < secret_num:
print("Almost there. My number is greater than {}."format(computer_guess))
else:
print("Almost there. My number is lower than {}."format(computer_guess))
computer_guesses.append(computer_guess)
else:
replay()
game()
4 Answers
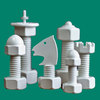
Steven Parker
231,275 PointsI see 4 major issues:
- line 9 has a "break", but it is not part of a loop
- line 17 needs another close parenthesis to match the opens
- lines 33 and 35 both need a period between the string and the "format"
You might also have some logic issues to work on, but that should get you going again.

Hinh Phan
2,564 PointsThe code work, but the while loop doesn't. Give me some tips please, Sir Steven?
import random
def replay():
play_again = input("Let me try again? Y/n")
if play_again.upper() == 'Y':
game()
else:
print("See you again")
def game():
low = int(input("Pick your min number: "))
high = int(input("Pick your max number: "))
secret_num = int(input("Pick a number between your max number and min number: "))
print("Guess my number between the my min number and max number: ")
computer_guess = random.randint(low, high)
print(computer_guess)
limit_guesses = 5
while limit_guesses < 5:
try:
computer_guess == secret_num
except ValueError:
print("Give me a number")
break
else:
if computer_guess == secret_num:
print("Wowhoo..I got it")
replay()
elif computer_guess < secret_number:
print("Almost there. My number is greater than {}.".format(computer_guess))
else:
print("Almost there. My number is lower than {}.".format(computer_guess))
limit_guesses.append(computer_guess)
else:
replay()
game()
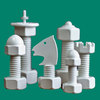
Steven Parker
231,275 PointsIt looks like you might not be using "try" correctly. That comparison on line 23 isn't likely to generate an exception (or do anything else, for that matter).
You already test the same comparison on line 28, so I suspect that what you really want in the "try" area is something to read in some input from the user and convert it into a number.

Hinh Phan
2,564 PointsTks for your help. Here is my code now. Give me feedback for improvement Please Mr .Steven.
import random
def replay():
play_again = input("Give me a chance buddy? Y/n")
if play_again.upper() == 'Y':
game()
else:
print("See you again")
def pick_a_number():
low = int(input("Pick your min number: "))
high = int(input("Pick your max number: "))
secret_number = int(input("Pick a number between your max number and min number: "))
print("Guess my number in my min number and max number: ")
computer_guess = random.randint(low, high)
print(computer_guess)
pick_a_number()
def game():
while True:
player_hint = input("Tell me if I'm WRONG or RIGHT: ")
if player_hint.upper() == 'RIGHT':
print("Wohooo I got you, buddy :)")
break
elif player_hint.upper() == 'WRONG':
print("I'll do it again")
replay()
else:
print("Think of a number buddy")
game()
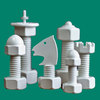
Steven Parker
231,275 PointsIt looks like your original question has been answered, and the code will run now.
At this point, you can just try giving it different input and check how it functions. It should be easy to spot anything you might want to change about how it works.

Hinh Phan
2,564 PointsSound good. Tks again for advises, Mr Steven.