Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial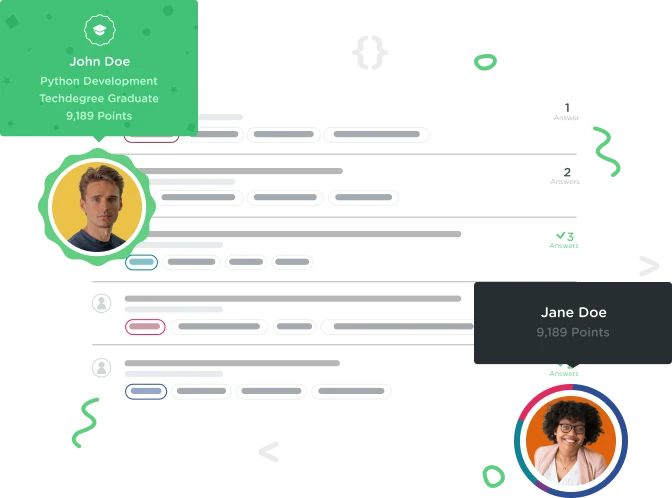
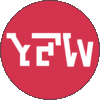
syalih
16,468 PointsReverse LinkedList?
Hi, can anyone please briefly explain me this below code what is exactly going on actually I don't get it from "for value in linked_list" because Node' object is not iterable" then how it is working in this function?
def reverse(linked_list):
"""
Reverse the inputted linked list
Args:
linked_list(obj): Linked List to be reversed
Returns:
obj: Reveresed Linked List
"""
new_list = LinkedList()
node = linked_list.head
prev_node = None
for value in linked_list:
new_node = Node(value)
new_node.next = prev_node
prev_node = new_node
new_list.head = prev_node
return new_list
llist = LinkedList()
for value in [4,2,5,1,-3,0]:
llist.append(value)
flipped = reverse(llist)
is_correct = list(flipped) == list([0,-3,1,5,2,4]) and list(llist) == list(reverse(flipped))
print("Pass" if is_correct else "Fail")
2 Answers
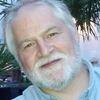
Jeff Muday
Treehouse Moderator 28,720 PointsThe key to the iterable is the __iter__() and that it must use a generator (Python yield keyword).
Another way to make a linked list is to use Python list() as a parent class. Though, in some respects, this defeats the point of the exercise to make a LinkedList() that would follow operation of the classic data structure.
In my current career, which is in academics and research, I rarely, if ever, work with Linked Lists. Not because these structures aren't fundamental, but because these are abstracted in libraries like NumPy, SciPy, TensorFlow, etc. These are in the libraries but never directly used by the application programmer. This is especially true of modern languages (Python, R, Ruby, Java) which have automatic garbage collection. So in some ways the concept of LinkedList() as a fundamental design pattern in these languages is antiquated. In the same way learning about assembly and machine language is antiquated. We use lists, dictionaries, objects and don't have to worry about allocation or disposal.
Why Learn about Linked Lists?
The Linked List is a rich structure used to build up other structures like (abstract) Stacks, Queues, Double-Linked Lists, Trees, and so on. These structures are ever-present in the "stack" and "framework", but as modern programmers and developers we are focused on getting applications into use or to market and don't have to re-invent the wheel!
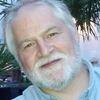
Jeff Muday
Treehouse Moderator 28,720 PointsWithout seeing the definition of the class LinkedList() it is impossible to tell. Here is a link that has a nice treatment of Linked Lists in Python.
https://stackabuse.com/python-linked-lists/
My guess is that your definition of LinkedList() class DOES NOT HAVE an __iter()__ method.
If that is the case, it will not yield values and cannot be used like a Python iterable (in a for loop). There are some linked lists which use a next() method or getter.
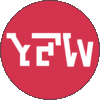
syalih
16,468 Pointshere is whole code please check and thanks for the resource for LinkedList :)
class Node:
def __init__(self, value):
self.value = value
self.next = None
class LinkedList:
def __init__(self):
self.head = None
def append(self, value):
if self.head is None:
self.head = Node(value)
return
node = self.head
while node.next:
node = node.next
node.next = Node(value)
def __iter__(self):
node = self.head
while node:
yield node.value
node = node.next
def __repr__(self):
return str([v for v in self])
def reverse(linked_list):
new_list = LinkedList()
node = linked_list.head
prev_node = None
# A bit of a complex operation here. We want to take the
# node from the original linked list and prepend it to
# the new linked list
for value in linked_list:
new_node = Node(value)
new_node.next = prev_node
prev_node = new_node
new_list.head = prev_node
return new_list
llist = LinkedList()
for value in [4,2,5,1,-3,0]:
llist.append(value)
flipped = reverse(llist)
is_correct = list(flipped) == list([0,-3,1,5,2,4]) and list(llist) == list(reverse(flipped))
print("Pass" if is_correct else "Fail")
syalih
16,468 Pointssyalih
16,468 PointsOhh now I totally get it thanks.