Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial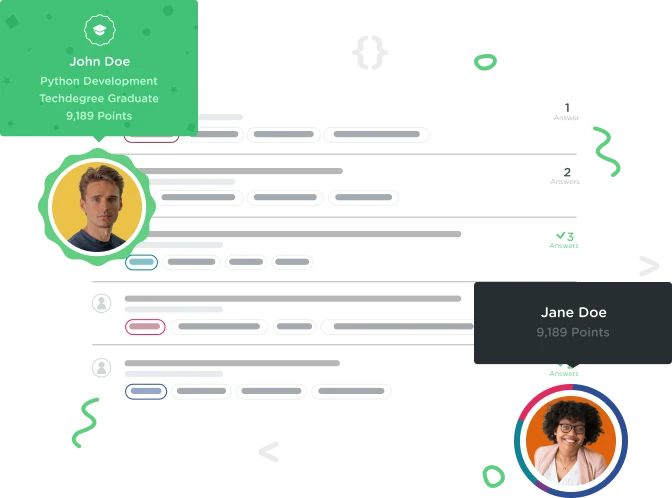

Teri Dent
11,515 PointsReverse Number Game and a tip about random.randint()
NOTE: I am using python 3 here.
If you are a having difficulty having the computer change the guess each time, I have solution that helps.
The random.randint() function takes two parameters. The first is the lowest number and the second is the highest number.
From the previous video we learned to generate a random number between 1 and 10 by using random.randint(1,10).
Here is where you can make the change. Imagine that you can use variables instead of numbers. It looks like this:
random.randint(lowNumber, highNumber)
Set the value of lowNumber and highNumber outside of the while loop. I set the lowNumber = 1 and the highNumber = 10.
Let's say that our number that the computer is trying to guess is 7.
Now, inside the while loop, the computer makes a guess. It guesses 5.
The number that the computer guessed was too low, so we need it to guess a new number from 5 to 10. Thanks to using a variable for lowNumber, we assign the value of the computer's guess to the lowNumber variable and then have it guess again. If the number was too high, we would assign it to highNumber and then cycle through the loop again.
My code looks like this.
import random
import os
os.system('clear')
def game():
guesses = []
highNum = 10
lowNum = 1
print("In this version I am going to try to guess what number you are thinking about.\n")
print("Choose a number between 1 and 10 and I will guess it in 5 or fewer guesses.\n")
while len(guesses) < 5:
secretNumber = random.randint(lowNum, highNum)
print("I think the number is %s." % secretNumber)
guess = input("Higher, Lower, or Correct?\n> ")
if guess == "C":
print("Yay computers are awesome")
break
elif guess == "H":
lowNum = secretNumber
elif guess == "L":
highNum = secretNumber
guesses.append(guess)
else:
print("I am bad at this game.")
play_again = input("Do you want to play again? Y/n \n> ")
if play_again.lower() != 'n':
game()
else:
print("Bye")
game()