Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial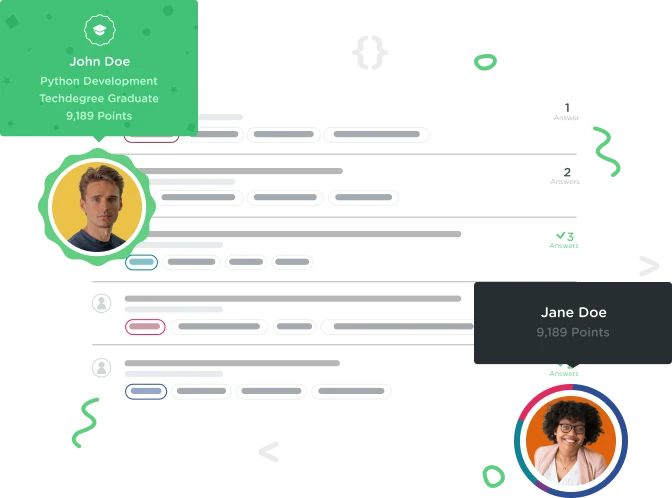

Jaeeun Lee
Courses Plus Student 1,246 PointsReverse Slice
def reverse_evens (iterable): return iterable[::-2]
When I enter `reverse_evens([1,2,3,4,5]) in my console, it returns [5, 3, 1], which is what the task asks for. However, the challenge keeps says it does not return the correct value. Why would that be?
def first_4 (iterable):
return iterable[:4]
def first_and_last_4 (iterable):
return iterable[:4] + iterable[-4:]
def odds (iterable):
return iterable[1::2]
def reverse_evens (iterable):
return iterable[::-2]
5 Answers

Ben Reynolds
35,170 PointsThis one's pretty hard, I'll give you a few hints to get started.
The index you start counting from will be different depending on whether and even or odd number of items was passed in. So I started by setting a variable to get the length of "iterable" and named it "length."
I used an if/else to check if length was even or odd and have two different versions of the return statement. The return statement you're using will work for 1, 2, 3, 4, 5 (odd number of items), but wouldn't work for 1, 2, 3, 4, 5, 6.

Jaeeun Lee
Courses Plus Student 1,246 PointsI think I misunderstood the question. I thought it was asking for even index numbers from the reversed list, not from the original list.

mauricio almonte
1,913 Pointsi did this and not even like this i got it to work, i don't understand where I'm failing.
def first_4(papa):
return papa[0:4]
def first_and_last_4(popo):
return popo[:4] + popo[-4:]
def odds(pipi):
return pipi[1::2]
def reverse_evens(pepe):
nana = len(pepe) -1
if int(nana /2) * 2 == len (pepe) - 1:
return pepe[-1::-2]

Ben Reynolds
35,170 PointsThe logic for my approach would look like this:
def reverse_evens (iterable):
length = len(iterable)
#if length is even:
#return one version of the slice
else:
#return another version of the slice

mauricio almonte
1,913 PointsGod Damwit!!! now i see it, my version only applies to only one scenario. i was like "naaaa they dont need the ELSE: statement"
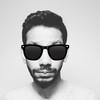
Marzoog AlGhazwi
15,796 Pointscan't find what is wrong with mu code
def first_4(iterable):
return iterable[:4]
def first_and_last_4(iterable):
return iterable[:4] + iterable[-4:]
def odds(iterable):
if len(iterable)%2 == 0:
return iterable[-2::-2]
else:
return iterable[-1::-2]

mauricio almonte
1,913 Points@Marzoog Al Ghazwi you are doing the last challenge, not the 3rd one, your "odds" funtion content is from the last challenge the one that is called "reverse_evens", so you are either missing the third challenge or you need to change the name of the funtion that is being expected
mauricio almonte
1,913 Pointsmauricio almonte
1,913 Pointsdid you see this one? I'm doing exactly what you said, and no luck! im trying this on my own environment and is working!