Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial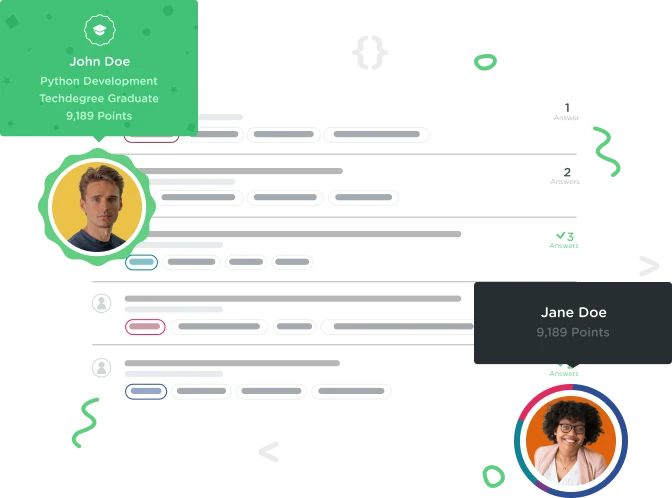

Hideki Matsuura
3,860 Pointsreverse_evens
Don't quite know what I did wrong in reverse_evens. I tested it out in the shell and got [5,3,1] from the list [1,2,3,4,5]
def first_4(itr):
return itr[:4]
def first_and_last_4(itr):
return itr[:4] + itr[-4:]
def odds(itr):
return itr[1::2]
def reverse_evens(itr):
return itr[::-2]
5 Answers
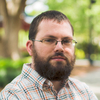
Kenneth Love
Treehouse Guest TeacherHey everyone, thanks for commenting!
I've update the CC to have better validation on that function. Hideki Matsuura, unfortunately your original code is wrong because it captures the even indexes from the back of the iterable. That happened to work out correctly if the iterable was an even number of members long but the actual passing code should work regardless of the contents of the iterable or the length of it.
I'm sure you can get past this challenge, though!

Jason Anello
Courses Plus Student 94,610 PointsHi Hideki,
Your solution is only going to work when the last item is at an even index. If the last item is an odd index then it will return all the odd indexes in reverse order.
One way to solve the problem is doing 2 slices. The first slice to get the even indexes and then a slice on that to reverse it.

Bauyrzhan Rakhmanov
3,958 PointsHello Everyone! for the last challenge this solution didn't help:
def reverse_evens(iterable):
return reverse_iterable[-1::-2]
this also doesn't work:
def reverse_evens(iterable):
reverse_iterable = iterable[::-1]
return reverse_iterable[::2]
Any ideas? Thanks in advance!
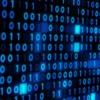
Alexander Davison
65,469 PointsIf the list is [1, 3, 5]
, however, it shouldn't return any values. It should return an empty list, because the numbers are all odd.
To fix this, you can use the modulo operator % to calculate the remainder of the division of two numbers. Example:
10 % 3 = 1
This is because 10 divided by 3 has a remainder of 1.
You can use this to see if the number is even or odd like this:
Let's say we're in the Python shell
>>> num = 5 # This is an odd number
>>> num % 2 == 0
False
>>> num = 4 # This is an even number
>>> num % 2 == 0
True
>>> # As you see, using num % 2 == 0 can indicate whether a number is odd or even
With this fact of num % 2 == 0
can return if the number is odd or not, you can finish your program like this:
def reverse_evens(itr):
evens = []
for num in itr:
if num % 2 == 0:
evens.append(num)
return evens[::-1]
I hope this helps.
~Alex

Jason Anello
Courses Plus Student 94,610 PointsThe challenge is dealing with even indexes.
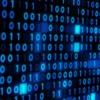
Alexander Davison
65,469 PointsOh... Whoops. XP
Dustin James
11,364 PointsThe following will pass the challenge:
def first_4(list):
return list[0:4]
def first_and_last_4(list):
first = first_4(list)
second = list[-4:]
return first + second
def odds(list):
return list[1::2]
def reverse_evens(list):
return list[-1::-2]
The following would be test case safe:
def reverse_evens(list):
try:
if len(list) % 2 != 0:
reverse_even = list[-1::-2]
else:
reverse_even = num[-2::-2]
except TypeError:
print("Non iterable item")
else:
return reverse_even
Or even another way to solve this is to get the even indices with a slice [::2] and then return a slice in reverse order [::-1]
def reverse_evens(list):
list = list[::2]
return list[::-1]
I hope this helps.

Jason Anello
Courses Plus Student 94,610 PointsHi Dustin,
Your first solution for reverse_evens is the same as what was posted in the question. It's not going to pass the challenge every time for the reason I mentioned in my answer. I think the challenge is running a random test case each time.
In your second solution, I wasn't sure which part of the code would generate a ValueError.
Dustin James
11,364 PointsJason,
In the event a non-iterable was given to the function, a ValueError would occur. Obviously the ValueError will not occur due to the challenge stating a single iterable would be given.

Jason Anello
Courses Plus Student 94,610 PointsCan you give an example of what could be passed in and which part of the code would raise a ValueError?
Maybe you meant a TypeError or something else?
Dustin James
11,364 PointsJason,
I did mean TypeError. Thank you. I have edited the answer. Again, the use of Try, Except, Else is just for good practice.